Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
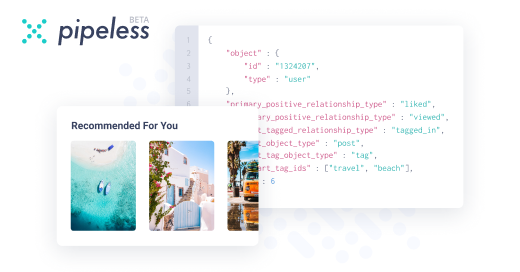
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "c++ lua"
-
So, you start with a PHP website.
Nah, no hating on PHP here, this is not about language design or performance or strict type systems...
This is about architecture.
No backend web framework, just "plain PHP".
Well, I can deal with that. As long as there is some consistency, I wouldn't even mind maintaining a PHP4 site with Y2K-era HTML4 and zero Javascript.
That sounds like fucking paradise to me right now. 😍
But no, of course it was updated to PHP7, using Laravel, and a main.js file was created. GREAT.... right? Yes. Sure. Totally cool. Gotta stay with the times. But there's still remnants of that ancient framework-less website underneath. So we enter an era of Laravel + Blade templates, with a little sprinkle of raw imported PHP files here and there.
Fine. Ancient PHP + Laravel + Blade + main.js + bootstrap.css. Whatever. I can still handle this. 🤨
But then the Frontend hipsters swoosh back their shawls, sip from their caramel lattes, and start whining: "We want React! We want SPA! No more BootstrapCSS, we're going to launch our own suite of SASS styles! IT'S BETTER".
OK, so we create REST endpoints, and the little monkeys who spend their time animating spinners to cover up all the XHR fuckups are satisfied. But they only care about the top most visited pages, so we ALSO need to keep our Blade templated HTML. We now have about 200 SPA/REST routes, and about 350 classic PHP/Blade pages.
So we enter the Era of Ancient PHP + Laravel + Blade + main.js + bootstrap.css + hipster.sass + REST + React + SPA 😑
Now the Backend grizzlies wake from their hibernation, growling: We have nearly 25 million lines of PHP! Monoliths are evil! Did you know Netflix uses microservices? If we break everything into tiny chunks of code, all our problems will be solved! Let's use DDD! Let's use messaging pipelines! Let's use caching! Let's use big data! Let's use search indexes!... Good right? Sure. Whatever.
OK, so we enter the Era of Ancient PHP + Laravel + Blade + main.js + bootstrap.css + hipster.sass + REST + React + SPA + Redis + RabbitMQ + Cassandra + Elastic 😫
Our monolith starts pooping out little microservices. Some polished pieces turn into pretty little gems... but the obese monolith keeps swelling as well, while simultaneously pooping out more and more little ugly turds at an ever faster rate.
Management rushes in: "Forget about frontend and microservices! We need a desktop app! We need mobile apps! I read in a magazine that the era of the web is over!"
OK, so we enter the Era of Ancient PHP + Laravel + Blade + main.js + bootstrap.css + hipster.sass + REST + GraphQL + React + SPA + Redis + RabbitMQ + Google pub/sub + Neo4J + Cassandra + Elastic + UWP + Android + iOS 😠
"Do you have a monolith or microservices" -- "Yes"
"Which database do you use" -- "Yes"
"Which API standard do you follow" -- "Yes"
"Do you use a CI/building service?" -- "Yes, 3"
"Which Laravel version do you use?" -- "Nine" -- "What, Laravel 9, that isn't even out yet?" -- "No, nine different versions, depends on the services"
"Besides PHP, do you use any Python, Ruby, NodeJS, C#, Golang, or Java?" -- "Not OR, AND. So that's a yes. And bash. Oh and Perl. Oh... and a bit of LUA I think?"
2% of pages are still served by raw, framework-less PHP.32 -
I had to open the desktop app to write this because I could never write a rant this long on the app.
This will be a well-informed rebuttal to the "arrays start at 1 in Lua" complaint. If you have ever said or thought that, I guarantee you will learn a lot from this rant and probably enjoy it quite a bit as well.
Just a tiny bit of background information on me: I have a very intimate understanding of Lua and its c API. I have used this language for years and love it dearly.
[START RANT]
"arrays start at 1 in Lua" is factually incorrect because Lua does not have arrays. From their documentation, section 11.1 ("Arrays"), "We implement arrays in Lua simply by indexing tables with integers."
From chapter 2 of the Lua docs, we know there are only 8 types of data in Lua: nil, boolean, number, string, userdata, function, thread, and table
The only unfamiliar thing here might be userdata. "A userdatum offers a raw memory area with no predefined operations in Lua" (section 26.1). Essentially, it's for the API to interact with Lua scripts. The point is, this isn't a fancy term for array.
The misinformation comes from the table type. Let's first explore, at a low level, what an array is. An array, in programming, is a collection of data items all in a line in memory (The OS may not actually put them in a line, but they act as if they are). In most syntaxes, you access an array element similar to:
array[index]
Let's look at c, so we have some solid reference. "array" would be the name of the array, but what it really does is keep track of the starting location in memory of the array. Memory in computers acts like a number. In a very basic sense, the first sector of your RAM is memory location (referred to as an address) 0. "array" would be, for example, address 543745. This is where your data starts. Arrays can only be made up of one type, this is so that each element in that array is EXACTLY the same size. So, this is how indexing an array works. If you know where your array starts, and you know how large each element is, you can find the 6th element by starting at the start of they array and adding 6 times the size of the data in that array.
Tables are incredibly different. The elements of a table are NOT in a line in memory; they're all over the place depending on when you created them (and a lot of other things). Therefore, an array-style index is useless, because you cannot apply the above formula. In the case of a table, you need to perform a lookup: search through all of the elements in the table to find the right one. In Lua, you can do:
a = {1, 5, 9};
a["hello_world"] = "whatever";
a is a table with the length of 4 (the 4th element is "hello_world" with value "whatever"), but a[4] is nil because even though there are 4 items in the table, it looks for something "named" 4, not the 4th element of the table.
This is the difference between indexing and lookups. But you may say,
"Algo! If I do this:
a = {"first", "second", "third"};
print(a[1]);
...then "first" appears in my console!"
Yes, that's correct, in terms of computer science. Lua, because it is a nice language, makes keys in tables optional by automatically giving them an integer value key. This starts at 1. Why? Lets look at that formula for arrays again:
Given array "arr", size of data type "sz", and index "i", find the desired element ("el"):
el = arr + (sz * i)
This NEEDS to start at 0 and not 1 because otherwise, "sz" would always be added to the start address of the array and the first element would ALWAYS be skipped. But in tables, this is not the case, because tables do not have a defined data type size, and this formula is never used. This is why actual arrays are incredibly performant no matter the size, and the larger a table gets, the slower it is.
That felt good to get off my chest. Yes, Lua could start the auto-key at 0, but that might confuse people into thinking tables are arrays... well, I guess there's no avoiding that either way.13 -
EEEEEEEEEEEE Some fAcking languages!! Actually barfs while using this trashdump!
The gist: new job, position required adv C# knowledge (like f yea, one of my fav languages), we are working with RPA (using software robots to automate stuff), and we are using some new robot still in beta phase, but robot has its own prog lang.
The problem:
- this language is kind of like ASM (i think so, I'm venting here, it's ASM OK), with syntax that burns your eyes
- no function return values, but I can live with that, at least they have some sort of functions
- emojies for identifiers (like php's $var, but they only aim for shitty features so you use a heart.. ♥var)
- only jump and jumpif for control flow
- no foopin variable scopes at all (if you run multiple scripts at the same time they even share variables *pukes*)
- weird alt characters everywhere. define strings with regular quotes? nah let's be [some mental illness] and use prime quotes (‴ U+2034), and like ⟦ ⟧ for array indexing, but only sometimes!
- super slow interpreter, ex a regular loop to count to 10 (using jumps because yea no actual loops) takes more than 20 seconds to execute, approx 700ms to run 1 code row.
- it supports c# snippets (defined with these stupid characters: ⊂ ⊃) and I guess that's the only c# I get to write with this job :^}
- on top of that, outdated documentation, because yea it's beta, but so crappin tedious with this trail n error to check how every feature works
The question: why in the living fartfaces yolk would you even make a new language when it's so easy nowadays to embed compilers!?! the robot is apparently made in c#, so it should be no funcking problem at all to add a damn lua compiler or something. having a tcp api would even be easier got dammit!!! And what in the world made our company think this robot was a plausible choice?! Did they do a full fubbing analysis of the different software robots out there and accidentally sorted by ease of use in reverse order?? 'cause that's the only explanation i can imagine
Frillin stupid shitpile of a language!!! AAAAAHHH
see the attached screenshot of production code we've developed at the company for reference.
Disclaimer: I do not stand responsible for any eventual headaches or gauged eyes caused by the named image.
(for those interested, the robot is G1ANT.Robot, https://beta.g1ant.com/)4 -
>dad nagging to learn python
>i hate python
>cuz i hate snakes
>whatever
>so started learning it
>with some awesome video tutorials
>even though i like the instructor
>i find the language
>boring
>uhh
>why do u use this?
>oh and you say it is easy 4 begineers
>oh good
>then why does only
>del keyword gets highlighted in pycharm
>just to look cool i guess
>lua is way better
>hope lua is more used than python
>and more supported
>but i still like C#
Moral: C# rocks10 -
Here's one that involves Windows, Linux (at the same time!), WInZip, Python, Lua and Minecraft, sort of.
So, when I get depressed I often find that old 2011 Minecraft videos help a lot from the nostalgia boost. If its stupid, but it works, it isn't stupid. Anyways, I was thinking about how much fun it must have been to just fuck around with code and make something like Minecraft. Naturally, I got a huge code boner and really wanted to do something I hadn't in a while: binding c to a higher level language.
This time around, I wanted to try Python. C + Python seems like a good pair. I watched a tutorial and it seemed pretty interesting and simple enough but I remembered that I actually like Lua a lot better than Python, so I went to the download page of Lua.
The download is a tar.gz so I let out a sigh and start typing "WinZip" into google. But no, fuck that, I hate 3rd party decompression programs on Windows. They all just give me this eerie feeling.
"This would be so much fucking easier on Linux"...
I remember that I haven't tried the Windows Subsystem for Linux. I guess it's time, isn't it?
I read the docs of how to do it. Nice little touch, they tell you how to enable WSL from PowerShell but don't mention the GUI way to do it. It's genuinely a nice touch.
So I get everything installed and go to the app store to choose a distro. I want Ubuntu. I click the Install button...
...
... "Something unexpected happened"
Windows and their fucking useless error messages. Jesus, okay. I restart computer. Same issue. I update Windows. Same thing. Uninstall WSL. Reboot. Install WSL. Reboot. Same thing. HOLY SHIT.
Went to bed. Woke up. Tried to install Ubuntu.
"Yea ok lul i'll work this time for no reason"
Finally unzipped Lua.4 -
I think I'm not as socially awkward as I once believed. I realize I just have nothing in common with the majority of people.
I don't watch sports, I don't care about cars, or fantasy football, or have any hobbies non-developers would find interesting.
If you want to talk about software patterns, finite automaton, Lua/C APIs, etc, then fuck yeah I'll talk to you all day long.5 -
Just found out about Yue, a GUI library for Node.js, Lua and C++ (and owners of the "gui" package on npm).
It is so awesome! The RAM usage is so low compared to Electron! Of course it has its limitations and doesn't use HTML + CSS + JavaScript, but you can still build really good applications with it!
I'll show you what I'm making at the moment soon, so stay tuned!
Anyways I've built the same application in Electron and Yue, here's the comparison of the RAM usage:16 -
------------Weeklyrant-------------------------------------
So I bought a smart watch to go with my Samsung Galaxy back when I was 12, and upon inspecting the watchface maker app I came across lua scripting files. This amazed me. Animations, complex math, hexadecimal color system, variables, sensors...
I spent about four months
learning/experimenting with lua until I discovered arduino and C++.
I am now 14 and have been fascinated with robotics and learned java and dos since.2 -
That specific moment a C# Developer (me) makes an API for Modding his own game but the mod language is Lua.
(And it works)6 -
What is your opinion on hopping from one language to another?
So far I have been programming for a little over a year and have used Python, Lua, Javascript amd C++, planning on trying Java in the very near future.
I've had quite a positive experience with switching languages so far, especially when starting out. Some concepts I wouldn't understand, but after seeing them from a perspective of a different language I finally got it. Do you think it's good to know a lot of languages, or in the long run is it better to master one?8 -
Another update on my 3D Software Engine:
Big progress since my last rant.
I now have a simple lightning with the Gouraud Shading Algorithm!
Looks really cool now!
Btw for those who are interested, I am following a tutorial but I'm translating everything to Lua/LÖVE2D. Here's the link to the tutorial:
https://davrous.com/2013/06/...8 -
what kind of dumb fuck you have to be to get the react js dev job in company that has agile processes if you hate the JS all the way along with refusing to invest your time to learn about shit you are supposed to do and let's add total lack of understanding how things work, specifically giving zero fucks about agile and mocking it on every occasion and asking stupid questions that are answered in first 5 minutes of reading any blog post about intro to agile processes? Is it to annoy the shit out of others?
On top of that trying to reinvent the wheels for every friggin task with some totally unrelated tech or stack that is not used in the company you work for?
and solution is always half-assed and I always find flaw in it by just looking at it as there are tons of battle-tested solutions or patterns that are better by 100 miles regarding ease of use, security and optimization.
classic php/mysql backend issues - "ooh, the java has garbage collector" - i don't give a fuck about java at this company, give me friggin php solution - 'ooh, that issue in python/haskel/C#/LUA/basically any other prog language is resolved totally different and it looks better!' - well it seems that he knows everything besides php!
Yeah we will change all the fucking tech we use in this huge ass app because your inability to learn to focus on the friggin problem in the friggin language you got the job for.
Guy works with react, asked about thoughts on react - 'i hope it cease to exists along with whole JS ecosystem as soon as possible, because JS is weird'. Great, why did you fucking applied for the job in the first place if it pushes all of your wrong buttons!
Fucking rockstar/ninja developers! (and I don't mean on actual 'rockstar' language devs).
Also constantly talks about game development and we are developing web-related suite of apps, so why the fuck did you even applied? why?
I just hate that attitude of mocking everything and everyone along with the 'god complex' without really contributing with any constructive feedback combined with half-assed doing something that someone before him already mastered and on top of that pretending that is on the same level, but mainly acting as at least 2 levels above, alas in reality just produces bolognese that everybody has to clean up later.
When someone gives constructive feedback with lenghty argument why and how that solution is wrong on so many levels, pulls the 'well, i'm still learning that' card.
If I as code monkey can learn something in 2 friggin days including good practices and most of crazy intricacies about that new thing, you as a programmer god should be able to learn it in 2 fucking hours!
Fucking arrogant pricks!8 -
Who thought Lua was a good idea for extending gameplay functionality??
It's weakly typed, has no OOP functionality and no namespace rules. It has no interesting data structures and tables are a goddamn mystery. Somebody made the simplest language they could and now everybody who touches it is given the broadest possible tools to shoot themselves in the foot.
Lua's ease of embedding into C++ code is a fool's paradise. Warcraft 3's JASS scripting language had way more structure and produced much better games, whilst being much simpler to work with than Lua.
All the academics describing metatables as 'powerful extensionality' and a fill-in for OOP are digging the hole deeper. Using tables to implement classes doesn't work easily outside school. Hiding a self:reference to a function inside of syntactic sugar is just insanity.
Nobody expects to write a triple-A game in lua, but they are happy to fob it off to kids learning to program. WoW made the right choice limiting it to UI extensions.
Fighting the language so you can try and understand a poorly documented game engine and implement gameplay features as the dev's intend for 'modders', is just beyond the pale. It's very difficult to figure out what the standard for extending functionality is, when everybody is making it up as they go along and you don't have a strongly-typed and structured language to make it obvious what the devs intended.
If you want to give your players a coding sandbox, make the scripting language yourself like JASS. It will be way better fit for purpose, way easier to limit for security and to guarantee reasonable performance. Your players get a sane environment to work in and you just might get the next DOTA.
Repeatedly shooting yourself in the foot on invisible syntax errors and an incredibly broad language is wasted suffering for kids that could be learning the programming concepts that cross all languages way quicker and with way more satisfying results.
Lua is hot garbage for it's most popular application, I really don't get it. Just stop!24 -
I don't get it.
I tried Kotlin on Android just for fun, and it doesn't support binary data handling, not even unsigned types until the newest version. Java suffers from the same disease.
How does one parse and process binary data streams on such a high end system? Not everything is highlevel XML or JSON today.
And it's not only an Android issue.
Python has some support for binary data, and it's powerful, but not comfortable.
I tried Ruby, Groovy, TCL, Perl and Lua, and only Lua let's you access data directly without unnecessary overhead.
C# is also akward when it comes to data types less than the processer register width.
How hard can it be to access and manipulate data in its natural and purest form?
Why do the so called modern programming language ignore this simple aspect that is needed on an everyday basis?11 -
I started with C#at the age of 12, it was way too complicated and I learned Lua for Computer craft instead. Next I learned Ruby for RPG Maker and finally Javascript for web Dev stuff.
Now comfortable enought with Javascript but put off by its quirks I learned Java for compiling faulty minecraft mods, but I only fully learned it in school.
At the same time I learned python and quite liked it for scripting, but ultimately it was not a good match for my projects.
Disapointed with Java I returned to C# and liked it quite a lot, but started learning C++. After touching my first Microcontroller I learned C and I've stuck with it as my favorite language.
Along the way I picked up Kotlin, in case I need to do some Java shit. Much better.
But how did I come to an understanding of programming. Well I got better after each time I got a layer deeper until I hit silicon.
I had tinkered with electronics since I was 15 so I just had to study some boolean mathematics in school and some vintage computers architecture and instruction sets and...
Then I finally understood how that shit I wrote in Lua way back when was actually executed by my hardware.
Allways dig deeper and you'll find enlightenment eventually. -
Hey everyone!
I'm on the hunt for new and exciting languages!
I'll state the ones I already know:
Python, Haskell, C(++), C#, Java, JavaScript, Ruby, Rust, Lua, about every kind of Basic, some branches of Lisp, BrainF**k, assembly, Octo (Chip-8) and GML(basically JavaScript).
I've also learnt some styling languages:
Html, CSS, Markup and Markdown.
Some misc languages too: Regex and a runny bit of the Wolfram Language.
Also I'm kind of limited to Windows, Linux and Android, as I do not own any Apple hardware except I have access to an old iPad, so are languages like Swift still good?
Thanks!28 -
Chased a bug for nearly a week. Huge code base, over 2mn lines consisting of a mess of C++, Python and Lua glued together.. Wrote a very complex distributed computational framework. End up with a elusive compiler bug in GCC.. FML
-
I was looking for alternatives of MC that are atleast usable, and found a thing called Minetest. This apparently is a Voxel Engine/Scriptable game, where you create games, that consist of mods/modules and other resources.
The cool part of it is, that mods and games etc. get handled by the game itself in a package manager type fashion, so the only thing you as a user have to do is selecting them in the ui, and putting them into your world.
It's this easy because the content is managed by a content database. This engine is built with multiplayer support by default.
Now comes the interesting part: apparently a few devs sat together and made a whole MC clone in this engine, and have called it Mineclone 2. I was testing it recently on a server and have to say, that it doesn't appear to be some low effort clone, but to my surprise is an actual playable and nicely looking game. So far i'm having fun with playing and even modding it.
Since the core is written in C++ and the mods and games content is written in LUA, you can easily writte new stuff for it, and even look at other mods stuff, to find out how to make it compatible or how to do certain things. The licenses usually allows to reuse and redistribute.
If you're looking for something like that, give Minetest + Mineclone2 a spin.6 -
Sitting in my beautiful chalet on this beautiful park. My garden nicely mowed. Sun in shining. Was in the zone the whole day. Now it's time to shift some gears and fetch some desperado's (will pass out after three probably, didn't drink for long time). Walk towards neighbor and drink together.
Life can be so beautiful14 -
Rant portion:
Fuck me, there's not a ton of great resources for Lua. I have the book, and it's actually fucking incredible, but as soon as I have a question which I would usually Google, either it's a SO question that almost hits the mark (but absolutely does not answer my initial question) or a mailing list that DOES answer my question but holy FUCK it's difficult to read!
I 100% recommend the Lua book, though. It's remarkably helpful and covers just about every little detail of the language and it's corresponding c API, and even some of how Lua works behind the scenes.
Non-rant portion:
Finished up the first version of my library and now I'm binding it to Lua and this time around I'm using all the best practices including setting and checking metatables so that Lua can't segfault. It's going great, I properly learned about the Lua stack, and I feel good. Cross-platform double-buffered command line via a scripting language... What a way to enter 2020. Everything went so smooth that I got to 3am before I realized what even happened.1 -
I discovered a language I didn't know AND i like.
It's not under active development anymore, but I decide it has a nice syntax. It's made by the writer of craftinginterpreters. There are still people writing some extensions for it.
I decided to implement socket support in it.
That went very well and the result is just BEAUTIFUL. But now, i have a collection of socket functions that require a file descriptor (sock) for every function like write, read and close. We're not living in the 90's. I want to do sock.send(), sock.write() and sock.close(). So socket as an object.
I wrote a wrapper and it is freaking TWO times slower! Hows that even possible.
I've made wrapping to object optional now. Bit disappointing.
The language shows off with benchmarks on their page. Their fibers can even be faster than Elixr. Yeah, if you only use the fiber and nothing else from language. I benchmarked string concat for example against python: 1000 times slower or so.
The source code of wren is so freaking beautiful. Before Lua was my favorite language regarding source. The extensibility is so great that I prefer to work on this one instead of my own language. They kinda made exactly what I wanted. I can't beat that.
For if you're interested: https://wren.io/
The slot way of communicating between host language (C) and child language (wren) seems odd at beginning but i became fan of it.
Thanks for listening to my ted talk.
What's your opinion about wren (syntax)?25 -
Couldn't be arsed with all the conditional compilation that angelscript required, so I dumped right back to good ol' lua for now.
Got lua in, vm started, loading strings and pushing/popping the stack.
Got SDL actually drawing as intended.
I don't know even half of what I'm doing.
Apparently header files that end in ".hpp" are specific to c++, while .h are for c headers.
I like the new SDL2 though, little bit different than SDL1. Not a lot of tutorials cover the difference, but I could kinda suss out from the documentation where I needed to adapt, even though I'm still pretty loose on the library, on the docs, and on c++ itself.
Still just a learning project.
Also, I'm continually surprised there isn't a portable, platform independent tool or little language just for replacing all pseudo-languages out there like .bat and .sh, and .zsh
Maybe even just a tool that standardizes it all, then takes config files that map the new standard to system dependant commands, so you can download the damn thing, configure the relevant environment variables, drop in the platform dependent configuration (or your browser or package tool detects what platform you are on and chooses the relevant package/download for your platform), write a console script and the tool automatically translates, and emits the system-relevant commands to that platform's console (so you don't even need much platform-specific code to do things like file access). -
If languages had slogans...
1) Java -- Buy one get two for free on your delicious NPEs.
2) C -- I burn way too much calories talking, let's do some sign language. Now see over there... 👉
3) Python -- Missing semi-colon? Old method. Just add an extra space and watch the world burn.
4) C++ -- My ancestors made a lot of mistakes, let's fix it with more mistakes.
5) Go -- Meh. I can't believe Google can be this lazy with names.
6) Dart -- I'm the new famous.
7) PHP -- To hide your secrets. Call us on 0700 error_reporting(0)
8) JavaScript -- Asynchronous my ass!
9) Lua -- Beginners love us because arrays start at 1
10) Kotlin -- You heard right. Java is stupid!
11) Swift -- Ahhh... I'm tasty, I'm gonna die, someone please give me some memory.
12) COBOL -- I give jobs to the unemployed.
13) Rust -- I'm good at garbage collection, hence my name.
14) C# -- I am cross-platform because I see sharp.
15) VB -- 🙄
16) F# -- 😴8 -
Got VS running, SDL up and running and outputting, and angelscript included. Only getting linker errors on angel at the moment, not on inclusion, but on calling engine initialization.
Who knows what it is. Devs recommended precompiling but I wanted to compile with the project rather than as a dll (maybe I'm doing something stupid though, too new to know).
Goal is to do for sdl, cpp, and angelscript, what LOVE2d did for lua. Maybe half baked, and more just an experiment to learn and see if I can.
Would be cool to script in cpp without having to fuck with compilers and IDEs.
As simple as 1. write c++, 2. script is compiled on load, 3. have immediate access to sdl in the same language that the documentation and core bindings are written for.
Maybe make something a little more batteries-included than what lua and love offer out of the box, barebones editors and tooling and the like, but thats off in the near future and just a notion rather than a solid plan.
Needed to take a break from coding my game and here I am..experimenting with more code.
Something is wrong with me.8 -
With a background of predominantly C style languages and Lua, it's quite refreshing to be able to jump into Python and feel comfortable in it's usage. I've been running through some exercises (https://www.practicepython.org/) and am having a pretty jolly time with it.
Just wanted to share that I'm having a great day so far, and that I hope you do too! :D -
Any opions/experience with Lua? Im using this language right now in my internship. Its suprisingly easy, but not as popular as javascript or c#.8
-
What programming books do you all recommend?
Language wise any books on C, GoLang, Python, Rust, and LUA are welcome
And topic wise I’m interested in books about computer science theory, network programming, low level programming, and backend programming are welcome.
I know it’s a wide variety of topics but some are stuff Im currently doing, I’ve already messed with and just really want to learn more or focus on, or plan to do it when I get around to it6 -
My first programming lan was Lua. And they who know that lan knows, that I may was confused when I switched to a 'normal' programming lan like c# or java, because when you init a string you just type: a = ":)". but you can still set it to an int: a = 10. So every vars in Lua aren't sticked to a type. The arrays also can have any kind of var in it.
So I never learned what a String, int, ... is. I didn't understood why a method can't just return anything or why an array has a length.1 -
!rant I've been meaning to learn Python for quite some time.
I've worked with Java, PHP, C, C#, JS, Ruby, even a bit of Lua. Any good books to recommend?3 -
In 2011,when i was 12, i was playing Garry's Mod with a couple of friends, and i don't remember the circunstance, but one of my friends said: "I wonder how games i made". I have no idea why i was never curious about this subject before,since i played A LOT of videogames, but this question did stick to my mind, so i decided that i would search about it. Searching, i discovered that Garry's Mod used the Source engine, and that it was made in LUA. Tried LUA. Understood very little. Lost my interest. And then, i would only attempt to program again back in 2015, where i learned C++ in high school. Then i learned SQL, and now learning Java. I also discovered that i LOVE programming, and now i have plans to graduate on CS.
-
I am learning Lua because I am developing in a game. My question is would there be a way to load C into Lua6
-
I started with a free trial of neobook (anyone remember that?), and then moved on to MS SmallBasic. At some point I had discovered Roblox and was stuck with that and lua for a few years. Eventually I started learning C# from a course, but never really used it much so I kinda forgot it.
School got a lot more busy for me and so I wasn't really able to do much programming for a few years, and even when I did, it was mostly bash and docker stuff. Then in the beginning of last year, I was able to start learning Go, which is now my current language of choice. -
I've been doing stuff on my free time after school for about 3 years now. And i cant keep working on projects without losing motivation or getting stuck without a solution and then giving up, i've also tried working with a lot of teams and friends but it seems like everything i do or i work on ends up cancelled or full of issues and roadblocks. any advice?4
-
Dabbled in primary school on Microsoft Front Page, but actually programming would have been the WoW private server scene. Started on C++, got confused, tried LUA, loved it, came back to C++ was still confused but could get things done. And then the story goes on and on.1
-
Wtf is this ESP32 shit and it's hype?
I bought one because I thought JS on a microcontroller? That's gotta be fun!
I'm a hobbiest when it comes to MCUs and I do JS as a job, so I tought I'm made for this and I know at least as much as all the kids on the internet doing it.
Nothing makes sense with this shit. You have to flash wildly compiled modules of WHATEVERTHEFUCK with fucken python development-kits which have something to do with Lua to give you some kind of node-REPL which answers you with a bunch of strangely-looking errors starting with "stdin:x:".
If this NODE-MCU shit is made for JS why is there stuff about Lua everywhere you go with this, I don't get a single thing. Now I'm sitting on about 3 different git repos of sdks or what do I know and know less than before.
Oh and there is actually not a single tutorial really targetting the esp32. it's all about that 82xx-model.
Then I start googling around a bit more - It's not even ES6, it's just some ES3/5 shit. Why would you even do this. That's actually harder to manage than classic C/C++. You get no gain with it. Fuck me.
Wtf bro.23 -
Lua/ C
Java/ C
Swift/ Objective C
Visual Basic / C or something
C / C#
……..
👆last reversed yet, that looks like a funny face 😑…
Where, am I?…8 -
Hi everyone hows it going today? been learning alot lately Question? when working with lib2cpp.so files whats the best inspector for them? and what do these files contain? (example: gamelib.so)
i know a .so file is C++ so i think it has something to do with offsets and memory ranges something like that.
but im trying to open one lol
we have moved to andlua and i learned the api fully
app: https://andnixsh.com/2020/05/...
AndLua+ app is a lightweight scripting tool that allows you to easily perform script programming and testing on your Android phone. This is a very useful tool for those who need script (android development or modding) programming. AndLua+ is based on the open source project lua. It uses a simple and beautiful lua language, which simplifies cumbersome Java statements. At the same time, it supports the use of most Android APIs, free installation and debugging, and makes your development on your mobile phone easier and faster. The permission requested is for you to write a program to use, please rest assured to use.