Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
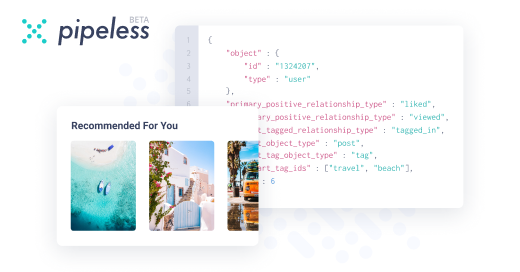
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
In Cpp its as simple as
#include <limits>
int imax = std::numeric_limits<int>::max(); -
aritzh7347yYou depend on integer overflow being deterministic for that, and, if I recall correctly, it is undefined behavior, so it might not work on some platforms. Much more reliable to use the <limits> header, like @dontPanic suggested.
-
Wack61367yIf you are able to work with the individual bits and you know the length of your datatype you could "construct" the value yourself. However I'd go with the limits too.
-
Aaand in Java simply:
int aReallyBigInteger = Integer.MAX_VALUE;
(For those that don't know/use Java int is a primitive type and Integer is object-based type) -
Root778217yI thought this was common knowledge?
I've been using unsigned int -1 For like fifteen years lol
(Though it is technically undefined behavior, so maybe not that common? idk)
I have not researched it so I'm not sure if this is a widely known thing but I figured out a sort of hacky way to get a max integer value:
-Declare an unsigned int.
-Subtract 1
-Divide 2
That is your max signed int value
int main(){
unsigned int a = 0;
a--;
std::cout << a / 2 << std::endl;
}
rant