Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
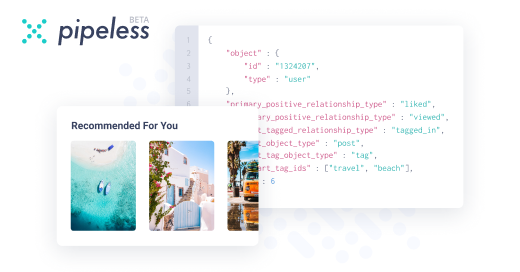
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
fuckwit12096yIn C array sizes have to be constant for the compiler to know how big that array is. try to put a 'const' before your variable that defines the size.
-
hashr8086yyou can use const keyword to define variable 'n'.
const int n = 100;
initializing const variable is compulsary -
Voxera112156yIf you cannot use const you could fake it by defining the function as one dimensional and calculating the index by multiplying the first dimension by n and adding the second.
Its not very elegant code but it works.
The underlying problem is that thats basically what the compiler converts your code to, but without const as mentioned, the compiler does not know how long it should jump to reach the next row. So you have to do the calculation manually. -
Voxera112156y@irene sure, but you end up with a similar problem. An array is just a pointer with some helpers, using a raw pointer does not remove the need to calculate the offset.
At least as far as I remember, but its been 15 years ;) I might have missed something. -
Voxera112156y@irene Ok, but my case was for the situation where the 2d array does not have a constant size but can be sent arrays of different sizes.
In which case the function cannot know the correct size.
Nv. Just me rambling about things I have not used for a long time :P
Related Rants
So I was writing some C code, pretty simple code. I had to pass a matrix to a function. The matrix had been globally defined as arr[100][100]. But the actual size of the matrix was stored in 2 vars m, n. Now when defining the function if I do like this:
void fun(int a[][n])
The code doesn't work as expected but when defined as:
void fun(int a[][100])
Works perfectly.
I have no idea why this is happening, any insight will be very helpful.
question
c