Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
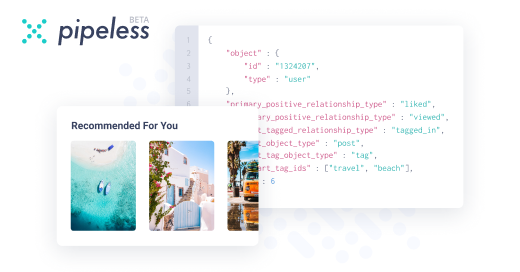
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
VINDIC2845y@shoop I am doing this out of hobby and enjoyment even tho I do want to get better I will leave actually securing my stuff to professionals like the people that run PGP
-
XOR is just that, XOR. It's entirely symmetric.
If you want asymmetric encryption, use RSA and encrypt a symmetric key with it. I'm sure something is wrong here, but I don't feel like analyzing this. -
VINDIC2845y@PrivateGER its ok GER I am really new to this but your right I should check into asymetric keys but as a novice I just am okay making a symetricKey for right now
-
A symmetric encryption should not even mention public or private keys, there only is a single key to both encrypt and decrypt with. I don't really see what you're trying to do here.
-
Voxera111075y@VINDIC how do the keys relate?
How do the encryption use them?
Or is the naming confusing things?
Public key and private key in cryptography usually indicates a crypto where one key can encrypt but only the other can decrypt.
Xor usually uses the same key that then need to be kept secret. -
VINDIC2845y@PrivateGER So I am thinking if you reverse xor and divide the public key and private you will get a 3rd hidden number that can be used to reverse the message but there is two points of failure there I know that I am pretty bad but Right now I am trying to get something that works.
I know its just pretty stupid -
VINDIC2845y@Voxera I am trying to make a specific system so you need to have the private key to swcrypt by xoring permutaions and multiplying by 20 using the original private key to generate a stronger key than enterd than using multiplication to get the public key so you can get a 3rd random number using division to use the private key and 3rd number to reverse the message.If that makes any sense
-
VINDIC2845y@PrivateGER it honetsly is but again I am doing this as a hobbyist for fun not to secure my AZZ
I would be using pgp or just regular AES if I was doing that
BVut I will try to fix :D -
Voxera111075y@VINDIC the problem I see is that unless the key gen code is secret, meaning its actually part of the key, any permutations on the key can most likely be reverse engineered.
Related Rants
using System;
using System.Text;
using System.Text.Encodings;
//Bitwise XOR operator is represented by ^. It performs bitwise XOR operation on the corresponding bits of two operands. If the corresponding bits are same, the result is 0. If the corresponding bits are different, the result is 1.
//If the operands are of type bool, the bitwise XOR operation is equivalent to logical XOR operation between them.
using System.Text.Unicode;
using System.Windows;
using System.IO;
namespace Encryption2plzWOrk
{
class Program
{
static void Main(string[] args)
{
//random is basically a second sepret key for RSA exhanges I know there probaley is a better way to do this please tell me in github comments.//
Random r = new Random();
int random = r.Next(2000000,500000000);
int privatekey = 0;
int publickey = 0;
string privateKeyString = Console.ReadLine();
byte[] bytes3 = Encoding.ASCII.GetBytes(privateKeyString);
foreach(byte b in bytes3)
{
privatekey = b + privatekey;
}
int permutations = random/ 10000;
if(privatekey < 256)
{
while(permutations > 0)
{
foreach (byte b in bytes3)
{
privatekey = privatekey + (privatekey ^ permutations)*20;
}
}
}
publickey = privatekey*random;
Console.WriteLine("your public key is {0}",publickey);
}
}
}
would this be considerd ok HOBBYIST encryption and if not how would I do a slow improvment I used bitwise to edit bits so thats a check :D
question
hobbyist
crypto