Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
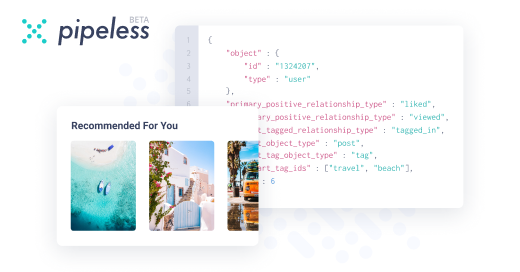
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
Using a trait and resolving it should create a jump table I think. If you're not using dynamic traits, it should be branchless by definition.
-
bad-frog5524y@HitWRight ok, if i understood it the right way,
accessing a method is indeed branchless, but it doesnt really solve my problem tho.
the idea behind me using a jump table is instead of feeding an identifier to the object, i feed it an index.
something like so:
object.method_list[index](arg);
lets say your function has 4 modes, instead of having to write 4 if statements, one for each mode you can have a jump table and feed it the index directly
and there's also the branchless conditionnals question.
lets say i want a looping array. instead of having a condition to check if i exceed array boundaries i can write something like this:
array[index * (index < array_size)]
(index < array_size) evaluates to 0 or 1 and i can use it to reset the index to 0.
now i know both of these cases should be covered by the compiler, but it isnt necessarily so and becomes more unlikely with more complex branchless conditions... -
Voxera115854y@bad-frog first question, do you need such optimization and if you do, test before looking for more complex code.
If rust does not contain any easy way to achieve what you are trying yo do, consider why, the developers of the language might have had a good reason. -
bad-frog5524y@HitWRight finally fucking got it.
the jumptable is not a part of the trait.
the jump table is to be made of traits.
but thats hacky af if anyone asks me.
now onto the branchless conditionnals.
if rust is supposed to be the next C its bound to have something of the sort -
bad-frog5524y@Voxera doesnt really answer my questions, doesnt it?
the very reason i love C is the freedom it gives you.
branchless conditionnals and jump tables are not all about optimisation.
once you get used to it, it also faster to write.
and in some cases it gives much more readable code.
quick example: i have an assignement that requires implementing a simple graphical engine.
instead of a wall of ifs and elses i got 3 lines.
2 which turn a heading, in rads into an index, one which launches the adequate function from a jump table.
from the optimisation standpoint, sometimes your gain is in orders of magnitude, because C doesnt only makes everything faster but allows for more efficient algorythms in the first place -
@bad-frog yeah the traits are there to document the jump table. It might feel hacky coming from low level, but the abstraction makes sense 99% of the time.
The branchless conditions are still a hot topic iirc. Mostly the developers working on encryption need it. And honestly couldn't find who else would want that, since having LLVM optimize code ir does catch most usual cases where you would want branchless programming.
There should be a RFC for compilation flags to not optimize out the usual way how one might implement such conditions. -
korrat6344yFor your array indexing example: you can write the same code in Rust. All you have to do is cast the bool to usize afterwards using as.
-
@bad-frog So yeah looking over it. It doesn't seem that rust has a more advanced way to approach branchless. But truth be told if you want to achieve most things you do in C. I do recommend reading on unsafe code. It allows to play with the pointer magic and let's go of some handholding.
-
bad-frog5524y@HitWRight or just implement it at the language level so that the compiler takes branchless as is.
like they did with asm from what i gather.
os builders might want branchless and everything numbers heavy so everything graphical or ai or scientific might want branchless too. especially since for the latter two optimisation directly translates into hard cash (not spent).
im cool with having a new C, but on the other hand, the language cant get in the way of programming. its hard enough as it is:p -
bad-frog5524y@korrat so what you say, is that you do like an inline if statement that you cast into int.
ok, could live with that. although since theres more going on readability would take a hit...
not that i could complain about, coming from a C background:p -
Voxera115854y@bad-frog no it did not answer the question :)
And yes your solution can be more readable and or performant
But one of rust major design principles is to make code less bug prone while still be performant enough.
And as you said, you do not know if the compiler might actually use jump tables under the hood.
That’s why I suggested to test, rust is a very modern language and might just be designed to handle some optimization that c and c++ cannot do due to much less strict syntax and more options.
I apologize if I sounded to critical but I want to challenge the statement that an index is more readable.
Short code is not always more readable and especially if the next dev is not used to jump table solutions the code can bu much harder to understand.
I have made the same mistake many times of assuming that just because i understand it directly its good, but as the writer I often already know the context which might not be clear to someone else.
So if readability is important (which is almost all projects with more than one dev) and if performance is not paramount (also mostly the case) I alway go for a more verbose coding style and delegate optimization to the compiler.
This often includes breaking out thing into functions that the compiler almost always will inline anyway and similar.
:) -
bad-frog5524y@Voxera basically i agree with everything you say.
in my school we have a peculiar constraint:
whenever we write code in C a function cannot be longer than 35 lines, you may have only 5 functions per file and sometimes you are limited to one file.
well, that is to say that i have seen many instances of code shortening that made the file all but unreadable (peer learning/correction).
not to mention all the times i rewrote a project because of said readability...
but in some cases it makes the whole thing much more radable. here's a snippet of original code its (its even less than i remembered):
getting the index:
index = (int)((game->job_hdg / (2 * PI)) * 8);
launching the function:
game->solve_x[index](game, x);
could have avoided passing "self" (game) as an argument, but im bound to 35 lines rule with this project: passing it externally makes for a shorter code
(cont.) -
bad-frog5524y@Voxera
as you can see, it can be very practical sometimes.
no repetition of clauses lessens probability of error.
having your formula in one place makes changing the behaviour of the whole selection trivial
and from the optimisation standpoint the advantages are obvious. especially if it were compound, nested condtions.
and since i dont want to bother with array overflow i can add buffer functions in the list to handle array overflows (p.ex duplicates at index max_size + 1).
i dont know about rust compiler, but i highly doubt it could anticipate the shenanigans im able to pull off.
and thats the very appeal of C. having control over the details.
i totally agree with you that rust has obvious advantages, and the same with all other language, but until it will give the programmer as much control as you get in C it wont replace it... -
@bad-frog that's why it's likely to replace C.
Given your example if someone comes and need to change the index function, suddenly a lot of parts how you allocate memory might break. You then introduce a possible out of bounds or similar exception. Especially if there is a border case you've (or more likely someone else) missed.
That's why people avoid indexed access.
Rust language is more than the language itself, tho. It standardizes how a new developer is introduced to the ecosystem. The compiler is not only the converter but the teacher. And that is where the language and community shines, since the one constant problem every language has is newbie developers.
C++ and C will get you results faster. But Rust will save you time in the long run, because you avoid common programming mistakes everyone makes.
Can't mention how many times I've felt quite stupid introducing race conditions and the compiler catching them before the first run :D -
Voxera115854y@bad-frog I understand that under such conditions and for highly optimized code it could be valid but had any one in my teams trued to get something like that through code review it would have been a hard no.
Magic constants, abbreviated method names, context less variable (index).
But I fo mot work with games but pharmaceuticals, correctness is magnitudes more important than performance every day of the week. :) -
bad-frog5524y@HitWRight @Voxera well, as much as i agree that in mass production speed of implementation and ease of use is important, there are plenty of places where execution speed is much more important than ease of use.
(again), i am not saying that rust couldnt replace C, it could if it gave the same level of control as C.
but what you say is that ease of use beats speed everytime. which is fundamentally not true. especially if a slower language translates into needing (much) more infrastructure for the same task.
rust acknowledges this need in its paradigm with the separation between safe and unsafe code, and the existence of the latter.
exactly to emulate the freedom that you have in C but said freedom is still artificially limited.
and until this wont change it wont really replace C. its a matter of function.
but it will make C jobs even better paying:) -
Voxera115854y@bad-frog actually, what I said is that for highly optimized code it can be valid, for example game engines or high throughput applications or tight very frequent loops.
But for most cases performance will be limited by conditions outside of the code, like database or network latency an d in all those cases you want to strive for readable easy to understand and extend code.
Even for an operating system you will many times be limited by hardware and bugs is mor if a problem than lower performance.
But when you really need to squeeze out every ounce if performance, sure I am pretty certain that C and C++ will stand their ground for many many years and I really do not think that that is the main target for rust.
Especially since you can combine them. -
bad-frog5524y@Voxera oh. i must have misread your comment. because i completely agree with what you just typed.
well, maybe except the "every last bit" in "every last bit of performance".
turns out C++ is a common language to build game engines of (i count in c++ because its paradigm is very similar to C), and i would hardly classify game engines performance as critical.
lets take unity for an example: it is bloated, it is slow, and it is leaking. it works, for sure, but in no means its good code.
now i see two reasons why they choose C-like:
-the enormous codebase of c++
-the enormous gains in algorythm efficiency: thanks to the fact that you can easily manipulate memory structure to solve logic problems, dicouraged by high level languages devs.
(cont.) -
bad-frog5524y@Voxera
then there's the ages old saying that everything is faster in C, but its bs to be honest. oop languages have proven multiple times that they can be as fast if not faster at a particular task.
but thats not impressive of itself since the underlying libraries are more often than not written in C so it boils down to optimisation.
the biggest difference is in the efficiency of the algorythm itself. compilers can catch some cases where it will optimise the code by itself, but not always. also there's the question how code and data is handled by the processor.
and thats why wrapping C doesnt cut it.
to get similar performance you would have to wrap your entire code.
for instance, if you declare a variable as static, it will remain loaded in the processor throughout the execution of the program. -
bad-frog5524y@Voxera
now can gcc, a compiler that has been around for decades, detect by itself variables that should remain on the stack?
no. for that you would have to run a program first and analyze memory behaviour, then recompile it.
but you cant be sure about your results, because the program can run in a different manner where said variable becomes useless bloat which slows down your entire process because it takes up valuable space in your stack, forcing more frequent loading from ram. if its a single pointer, its no big deal, but if it ends up being half of your variables, you might have an issue with that, especially if you use recursivity.
the programmer is the one to make that call. compilation would take the time of a unit test if it were otherwise, if only it could generate an adequate unit test -
bad-frog5524y@Voxera
on the other hand, i am not trying to convince you that front-end should be written in C, obviously. but the backend oftentimes should be, and in fact, it is.
you may use sql syntax to access your database, but the underlying processes are written in C and C++.
and if you need a custom solution, you will be presented with the following consideration:
do you go with slower code, requiring less specialisation from your hires but requiring more infrastructure to serve the task or do you go with faster code, requiring less infrastructure?
well, ML AI's are handled user level by python, but the underlying libraries are written in low level languages: C, c++, and pascal are the industry choices from what i gather.
do these require ultimate optimisation to be useful? -no.
if it costs you to hire the best of the top 100 brains in the world, it wont be done. conversely, it doesnt mean they dont want optimised code, as it translates to being able to compute more using less HW. -
bad-frog5524y@Voxera
now that i think of it, the only instance where you need your code to be optimized to death, is military robotics (in the large sense as in tomahawk missiles are robotized). -
Voxera115854y@bad-frog In the comparison between developer time and hardware, hardware is in many if mot most cases cheaper than developer time.
And yes most libraries and sql databases and more are written in C or C++.
But that is most likely due to most having been around many years and when they started C and C++ had not only performance benefits but also memory.
As you said, in many cases “performance” is not just fast algorithms and instructions but memory.
The big problem is that memory management is also one of the main cause if bugs.
Thats where languages like rust aims to help.
By offering good enough performance but exceedingly better and less bug probe memory management and multithreading you can build better software and C++ can still be used where you really need full control, like performance and cryptology.
I think C++ will stay long after I leave the world.
Its just to much built in it to replace existing. -
bad-frog5524y@bad-frog errata: static variable is not duplicated when in recursive, and aerospace/military HW is coded at the asm level, since it is (or rather should) be custom hardware. (however, since darpa seems to have started recruiting based on skin colour and gender instead of merit, it might change in the near fufutre)
-
bad-frog5524y@Voxera yeah, with the libraries and freedom it offers c++ is here to stay. pure C could and should be out-phased as a production language, as c++ gives you more logical tools to play with and is much more actively maintained.
and memory management isnt that hard, you just need to be structured in your work. once you get used to it, it becomes trivial given the tools you are given to play with (want to keep track of everything? just make a list. big array, self adjusting array or linked list depending on your needs.)
sure, you have to learn how the machine you code on works to actually take advantage of coding in C-like (because manual optimisation can make your code slower), but for me it is easier than anticipating how high level languages work.
fewer components: less complexity.
instead of having to deal with HW optimisation only as in C, i have to deal with higher level considerations like programming style pushed by the language influencing the whole data structure on top. -
bad-frog5524y@Voxera all in all i think we can agree that languages will be different because of the paradigms they are based on, and looking for a one-size-fits-all solution is very hard, and in the end considering economical imperatives, probably wont happen.
ps C should be out-phased in production with actual computers.
i dont think it wil ever disappear from microcontrollers though, as it is simpler than c++ and thus writing a compiler for it is easier...
hey devs, quick question:
does rust support branchless conditionnals and jump tables, and if yes where to look for syntax?
i found no example for branchless so far and jump tables are scary in rust (and i need a library to use them, it seems).
inb4 "why in the hell/ what possible use those have?!":
i use branchless to make a looping array and jump tables whenever there's more than 4 if statements with equal probability, and the deciding factor is a numeric value, so quite often.
question
rust c shenanigans