Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
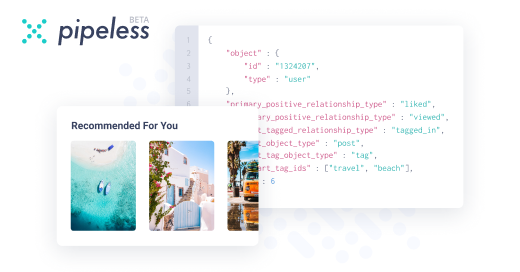
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
Demolishun35878306dHere is one that just moves the ifs around and puts the statements on one line (in one case). This just complicates the logic:
https://stackoverflow.com/questions...
The same number of evals will occur regardless. -
Demolishun35878306dAlso, please, STOP DEFINING FUNCTIONAL PROGRAMMING IN RELATION TO WHAT IS OOP! Its description should be able to make sense on its own.
-
Liebranca1186306dI could replace a switch with a table, and the table definition with an emitter. Is it simpler? No. Is it faster? If you count the cycles, then no.
So let's say I write a compiler instead, and bury the inconvenient computational reality beneath a *literal* brazilian carnival fuckpile of abstractions so that the """if-less""" code is a wee bit prettier and performant.
I can then have an army of olimpic jackoffs act as my righteous missionaries and embark on a crusade to spread the gospel: conditionals are evil and you don't get it cause your brain is a fool of stewpid.
That's the functional crowd, so fuck them. There are good ideas. Minimizing global state, being wary of side effects and making the output of functions predictable are good ideas. Being an inbred evangelizing cocksucker, not so much.
Head under the fucking hood. Code can be simpler *and* easier to maintain, you just have to stop adding dumbass abstraction where none is needed. -
Demolishun35878306d@Liebranca yes, studying some of the functional approaches I have learned to avoid mutation in certain circumstances. I have also learned to use const a lot more to prevent the next guy from screwing up a constant. I also tend to use function pointers a lot in my code. I do need to spend more time learning what lambda calculus is about though. It sounds interesting. I have a genuine interest in learning the benefits, but I keep finding head scratchers that don't make sense..
-
Lensflare18140306dvar lengths = []
for(var i=0; i<strings.length; i+=1) {
lengths.append(strings[i].length)
}
vs.
var lengths = strings.map { s => s.length }
There are some exceptions but mostly functional is much clearer than imperative.
Functional style describes the desired result kind of declaratively.
You describe your intent and let the function take care of how to get there.
Imperative style describes how to get to the desired result step by step.
The intent isn‘t always clear. It‘s like writing down the ingredients and the cooking instructions without knowing what the result is supposed to be.
And it‘s true: Once you get used to it, you don‘t want to go back to imperative style. -
jestdotty6226306difs?
like, filter?
I actually used to make switch statements in js by making objects.. uhhh.. I dont even remember for what. ironically js probably has switch statements and I never used them
like you could define what happens if X is 15, attach it to o[15] = doSomething
then o[x] && o[x]()
whether thats good or not everyone will say no, but I swear I had good reasons for doing this often (that I now cant rememberr)
maybe it was like a custom kernel process id lookup table thing or something idr
definitely good when working with messy data and you wanna "sort"
functional programming, everyone kept telling me to do Haskell, but I guess Im lazy. I think its just for the enlightened that see code as a series of tubes data goes through, kind of like fluid dynamics. and I guess if you get over the hump the brain can natively think in a series of tubes
technically filter is an if statement, though I don't know if I've encountered a filter that could have more than two branches? must exist -
Demolishun35878306d@Lensflare I use those functions in C++ when it makes sense. What I don't get is when you have multiple operations on the same list. Do I write a lambda the performs those in order? Do I run the loop 3 times? What if I have to operate on 2 lists simultaneously? With those I am stuck with a trad for loop.
Edit: With Python I could just zip the lists. That was really convenient. -
Lensflare18140306dIn my example, the imperative code reads like this:
Make a new lengths collection.
Iterate over all indices of the strings collection.
For each index, use it to access the respective string in the strings collection and get the length of that string.
Append the string length to the lengths collection.
The functional code reads like this:
Map each string from the strings collection to its length.
This is a trivial example. Real world examples get much worse. -
Liebranca1186306d@Demolishun What I understand is that being able to make more assumptions about an expression -- having higher predictability -- allows one to reduce it further, much more than it would be possible when unknowns are involved.
Think of functions as symbols within such an expression. If you can make assumptions about the functions themselves, then this reduction can be applied to an entire program; so less code and less work, generally speaking.
The idea translates cleanly to writting machine instructions; I'm able to consistently apply it. But I got this from basic algebra, not calculus.
Main problem is that mathematics is not programming. There is overlap but it's definitively not the same discipline, and trying to force them to be is more detrimental than anything. -
Lensflare18140306d@Demolishun I wasn‘t you to answer that quickly. My last comment was a continuation of my first comment.
Now to your last comment:
Every functional style language or lib has all the primitives to allow you to chain and compose functions to reach any result. E.g. zip should be available everywhere.
Sometimes it is easier to come up with an imperative solution rather than a pure functional one and that‘s ok. Use what fits best or what gets the job done.
Functional style is not a club that prohibits you from using anything else. It‘s just a great tool that allows you to be more effective and more expressive. -
Lensflare18140306d@Demolishun
> What I don't get is when you have multiple operations on the same list. Do I write a lambda the performs those in order?
Yes.
Either chain 3 map functions doing each of your operations, or write those operations in order in a single map function.
When I talk about FP, I don‘t distinguish between functions and lambdas.
map is a function that takes a function as its single parameter
Having a hard time thinking the alternates to if statements is a good idea. I was genuinely curious how this was done. The examples I am finding seem to just spread the logic everywhere across multiple objects. To me this makes the logic objectively less clear. I didn't understand the obsession with objects until I saw the examples that creates a fuckton of boiler plate objects. How someone can say this is preferred over a few if statements boggles my mind. I actually am trying to understand the functional mindset as well. It is not going well for me. I can sorta see some value in using a map. Technically a lookup could be faster. But again it spreads the code all around adding more boilerplate.
https://blog.bitsrc.io/reduce-if-el...
https://dev.to/phouchens/...
Is it because these are contrived examples? I initially searched to find ways of reducing ifs in a functional approach. I did find it in the second example. I was however hoping to find that by lazy eval or something. I see people making references to how one you "get it" functional logic is easier to understand and evaluate. I cannot tell if this is straight up gaslighting or my brain is just too fucking imperative.
rant
ifs