Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
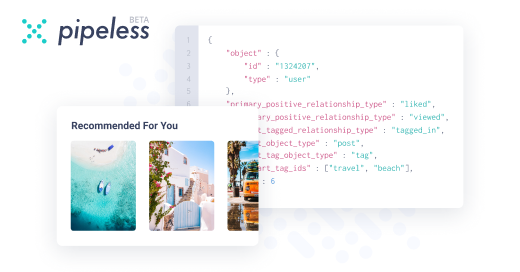
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
inaba45666yI'm amazed how you don't even need to write a for loop in php to get a for loop.
Also, any reason you need to wait for 50 minutes? -
inaba45666y@ganjaman setTimeout(callback, ms);
The reason why there's a callback is because it needs to be non-blocking. A sleep function like in PHP wouldn't work since it would result in the event loop being blocked, which would mean you wouldn't be able to use the page at all since the event loop also draws the content and stuff.
Also made a codepen https://codepen.io/anon/pen/xyXyYW
(Also the fact that the callback is in the beginning is dumb as hell) -
@inaba oh yeah, i just put an empty log in the callback. Man i havent used js in like a year
-
rant1ng44416yI found that on github in my lazy, I'm tired of working today way that I attempted to solve this problem
Was kind of similar to a lot of other examples that I didn't feel like trying to understand.
I get it, Javascript is a delicate, asynch flower.
k. I'll tackle this in the morning.
I still hate it.
sue me. -
tbodt7366yYou don't need the asyncForEach thing, you can just use a for loop:
for (const num of [1,2,3]) {
}
or, if you prefer, Promise.all + map:
await Promise.all([1,2,3].map(num => {
})) -
@ganjaman 420 msecs is a bit fast for rolling one up though... Or do I just suck at rolling?
-
@tbodt the OP wanted to have something like Sleep(3000) in JavaScript, which would be a blocking wait. That was my question what could possibly go wrong with such an approach, and that's why the waiting isn't done like that in JS. Of course, asynchronous wait is a bit more code than Sleep(3000). For good reason.
I need to delay execution of code in a for loop, how do I do that?
PHP: Sleep(3000)
Javascript:
const waitFor = (ms) => new Promise(r => setTimeout(r, ms))
const asyncForEach = (array, callback) => {
for (let index = 0; index < array.length; index++) {
await callback(array[index], index, array)
}
}
const start = async () => {
await asyncForEach([1, 2, 3], async (num) => {
await waitFor(50)
console.log(num)
})
console.log('Done')
}
start()
Fuck you Javascript
rant
wtf javascript