Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
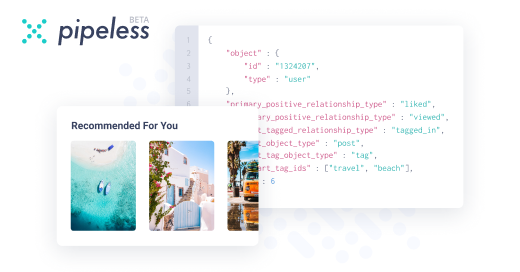
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
dmonkey21567yIn MIPS architecture it's two pseudoinstructions: one for the division and another to get the remainder.
The former takes about three or four instructions, the latter it's just a mov.
Don't know about Intel and/or AMD, but I think it might be similar... -
olback107547yThis is certainly not the fastest or most efficient way but it's one of the easiest to add conditions to.
-
olback107547y@billgates Fizz and Buzz are in the object on lines 1-4. The rest of the code loops from 0 to 100, then for every loop, looping through the object and checking if i % Number(key) = 0. So adding another condition like mod 7, would be easy. Just add '7': 'Bazz' to the object.
-
donuts235727y@olback yes took me a while to read that the function was printing for each and Number I guess is done internal int to string function
-
Root784387ydef fizzbuzz(count=100)
1.upto(count) do |number|
line = ""
line += "fizz" if number%3 == 0
line += "buzz" if number%5 == 0
printf(line + "\n")
end
end
fizzbuzz() -
anekix3897yThe whole point of doing run time analysis is that you do not have to worry about how much time an instruction takes to execute. Same instruction on a very old hardware might take more time than a newer one but the run time remains same if you are using asymptotic notations ( which you are) 😉
-
anekix3897y@billgates it will always be O(N) no matter what trick you use. Because To print fizz bizz you have to check all elements at least once so for the input size N the run time will always be O(N). I suggest you read a little on run time analysis , it will give you a more clear idea on why such optimisations are useless
Related Rants
Was reading about FizzBuzz/Algo again and I guess the modulo run-time.
The most optimal solution I came across is like:
if (x % 15) FizzBuzz
else if (x % 3) Buzz
else if (x % 5) Fizz
else x
But then how long does % take? Should you care?
I was thinking it should use variables:
var f = x % 3 == 0
var b = x % 5 == 0
if (f && b) FizzBuzz
else if (f) Fizz
else if (b) Buzz
else x
question
algo
fizzbuzz