Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
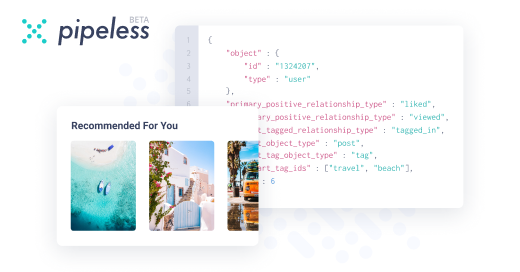
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Related Rants
So here the function that does same thing as new operator in javascript:
// we define our function Person that assigns properties to THIS that points to some object
const Person = function(name, lastName) {
this.name = name;
this.lastName = lastName;
}
// in Person prototype property we define our functions
Person.prototype.getName = function() {
return this.name;
}
Person.prototype.getLastName = function() {
return this.lastName;
}
// function that simulates new operator
// first argument is a function that would act as constructor
// second argument is an arguments that would be passed to constructor
function New(func, ...args) {
// with Object.create() we create a new object and assign [[__proto__]] from "func" prototype property
let object = Object.create(func.prototype);
// here we're calling "func" with THIS pointing to object
func.apply(object, args);
// then we return it
return object;
}
let person = New(Person, "Name", "LastName");
console.dir(person);
// so this is how prototype OOP works in javascript
random
function_as_class
javascript
operator_new
prototype