Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
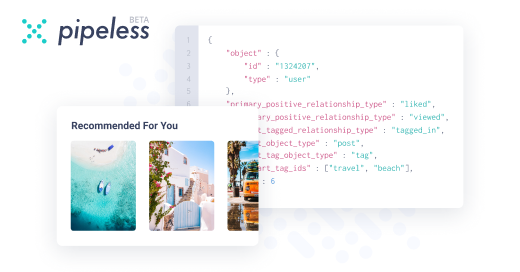
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Related Rants
As I was refactoring a class in a TypeScript project, I changed calls from `this.config` to `this.getConfig()`.
Suddenly, the tests were failing as somehow the live credentials were used from within the test.
Digging deeper I discovered this.
interface Base {
public config;
public getConfig();
}
So far so good. Wondering why config needs to be public, though nothing too shabby, let's look further:
class MyImpl implements Base {
constructor() {
this.config = this.getConfig()
}
getConfig = () => someGlobalVar;
}
┻━┻︵ \(°□°)/ ︵ ┻━┻
Why would you do this? This breaks dependency injection completely.
In the tests, we were of course doing:
testMe = new MyImpl();
testMe.config = testConfig;
So even though you have a getter, you cannot call it safely as the global var would take precedence. It's rather used as a setter within the constructor. WTF.
Sad part is that this pattern is kept throughout the entire codebase. So yeah for consistency!?
(And yes, I found a quick workaround by doing
getConfig = () => this.config || someGlobalVar;
though still, who in their right mind would do something like this?)
rant
dependency injection
oop
typescript
tdd
you are doing it wrong
javascript