Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
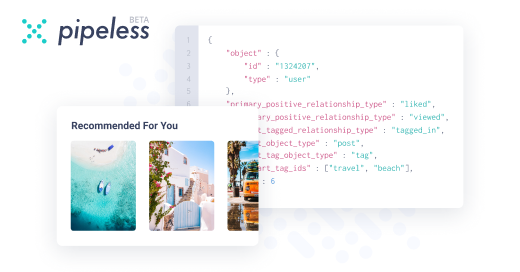
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
theuser47565yThe roughtest solution would be, for each evaluated fibonaci number, you can parse it to a string and then split them into a list. Then its just the case of looking at the len(array).
-
Write the algorithm on paper without looking at the code. Once you figure it out there, put it in code.
-
fuckwit12135yDoes python have a number type that I'd not constrained by the usual bit restrictions? Meaning can they be. Infinitely big?
Secondly with that recursive algorithm you will probably never find that number.
Memoization will definitely help but I think that this still would take a very long to find that number -
Don't use recursion for that. Just use a loop and store the two last numbers. Recursion doesn't like stack frames in the hundreds, let alone the thousands.
And ensure that your number type can store numbers that big. Floats aren't good for this, you'll need a number type with _way_ more bytes. I'd suggest you look for a better number type, and double check the exact implementation. Eg. Floats can be arbitrarily large, but they will eventually lose accuracy. -
dmonkey21835y
Related Rants
I want to print the first number in the Fibonacci sequence to contain over 1000 digits. I got fibbonaci to work but I cannot seem to figure out how to implement the "Contain over 1000 digits".
Do I make a list to store the fibbonaci numbers in then do a statement?
My Python 3 Code:
def fibonacci(num):
if num == 2 or num ==1:
return 1
return sum([fibonacci(num - 2), fibonacci(num - 1)])
print(fibonacci(7))
question
python