Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
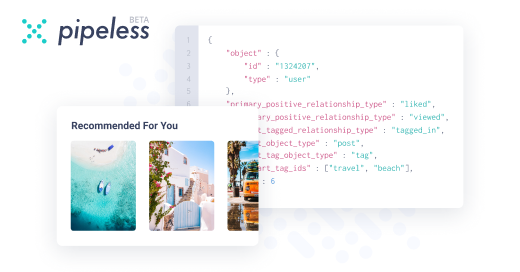
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
If x and y don't change then yes; you're creating new functions that always do the same thing each time the function is invoked instead of just once.
-
donuts235725y@spongessuck well x and y would be parameters extracted from req, user input.
But I wasn't sure if Node would be smart enough to optimize it. But I guess maybe that's the difference between compiled and interpreted languages though not sure how C#, Java treat anonymous functions and classes either... -
p100sch14225y@billgates they just create an anonymous object that references the scope and object that called it and executes the anonymous function as it's own method. At least in Java and C#. Can lead to memory leaks.
-
donuts235725y@p100sch so in that case they are similar to the above in JS?
I don't think though the anonymous function can refer to variables other than the ones passed in though (x,y) => { return x + y; }? -
p100sch14225y@billgates It can access everything present in the scope. As I said in C# and Java that is true as well. Just Java does not let you override them directly. You can read and call methods or functions on them.
-
- It makes much more sense to wrap the functions with promises.
- functions that should be inlined, you can inline yourself, or just make a normal function, and call it.
- scope weirdness in node is weird. Accessing the scope does not cause a leak, bc the function belongs to the same scope, and gc'ed along with it. But when you trigger some sort of a long running thing in the function, that will prevent the scope from gc.
In JS/Node, is there a performance and memory cost to nested functions?
I.e.
function handle(req, res)
{
var x = 5, y = 10;
function add() { return x + y; }
function multi() { return x*y; }
res.send(add() + multi());
}
As opposed to taking out the 2 functions and making them accept x,y as parameters.
question