Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
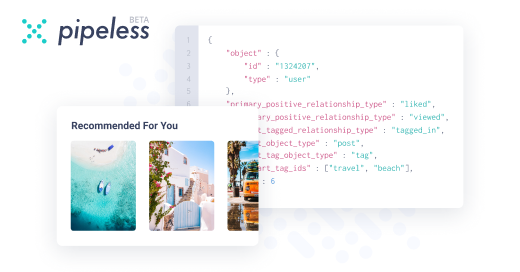
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
Second what @netikras said.
Also, there are many portions that would benefit from being expressed as pattern matching instead of elif chains. It will likely perform better.
Enumerations should be considered for the numeric magic values and constants.
Professional bit, if you intend to put it into a resume: considering replacing the polarizing usage of gay with underwhelming or painful. -
Boi I feel that itch in my fingers to jam everything into maps:
private static final Map<String, Integer> INPUT_HANDLERS = new HashMap<>(){{...}}
private static final Map<Integer, String> OUTPUT_HANDLERS = new HashMap<>(){{...}}
private static final Map<Integer, Function<Integer, String>> INSTRUCTIONS_INTERPRETERS = new HashMap<>(){{...}}
private static final Map<String, String> COMMAND_PROCESSORS = new HashMap<>(){{...}}
Yes, there's some additional memory usage. And a few additional stack entries. But that'd give the code more structure and more readability IMO... -
@netikras
Reading through this makes me wish python had supported point free style. There's lots of this that could benefit from being composable. -
Parzi88334y@SortOfTested i don't know what you mean by pattern matching and i thought i cleaned up the comments, that one's my bad
@netikras listen man sometimes i forget how if statements work because python doesn't always like them please remind me what you mean by "maps/dicts" they sound familiar but i can't remember
and also where would those go -
@Parzi
Forgot it's still in pep. Been noodling with stuff recently. In future releaes python will change a loooot 😆
https://python.org/dev/peps/... -
Parzi88334y@SortOfTested i'm sorry when the fuck did some beautiful bastard go "hey python could really benefit from case statements" that would've made my life so much fucking easier
-
@Parzi
Gotta follow the proposals 😋
As to Maps:
Hashmaps store O(1) lookups of values based on a keyed value.
Instead of
if(value == "other value")
evenMoreValue = constant
You can just do
evenMoreValue = dict.get(value)
https://edureka.co/blog/...
Too lazy to dig up the real pydocs, internet search is poisoned. -
I dislike your use of global variables.
This:
ROM_in = open(romName,"rb")
ROM_data = str(hexlify(ROM_in.read())).strip("b'").strip("'") #make hex string and strip py3's surrounding garbage off
ROM_in.close()
del ROM_in
Should be replaced with
with open(romName, "rb") as rom:
data = hexlify(rom.read()).decode()
Do not delete the file object manually, Python will do it anyway (it just might take some time).
Don't name your variables "...byte" if they contain a string, while you are at it, add type hints (https://python.org/dev/peps/...).
Document functions with docstrings, instead of writing a comment before them.
Mind the style guide of Python, commonly PEP-8 is followed (there are formatters for you, like autopep8 or black). -
Parzi88334y@sbiewald please read the opcode processor to see why that mess there makes my life so much easier later (also you can see that i don't either from comments in the code, it looks godawful but python namespaces are weird sometimes and "use a class" just makes it one var instead of several)
-
@Parzi They are key-value pairs.
There are several functions in your code that have large if-else trees with consistent simple input/output types. So
if charIn == " ":
inputIO = 0
elif charIn == "l":
inputIO = 1
charIn == "o":
inputIO = 2
elif charIn == "r":
inputIO = 3
else:
return "INVCHAR"
could become smth like
INPUT_HANDLERS = {
" ": 0,
"l": 1,
"o": 2,
"r": 3
}
def inputHandler(charIn):
global inputIO
charIn = charIn[0].lower();
if charIn in INPUT_HANDLERS :
inputIO =
INPUT_HANDLERS.get(charIn[0].lower())
return "INVCHAR"
return ""
Might be a problem with dicts having numbers as keys though. IDK how you Py folks usually deal with that.
As for COMMAND_PROCESSORS -- the values in the dict would be callback functions (if that's possible in Py)
P.S. thanks to python's syntax and for using indentation to define blocks -- good luck reading my example :D -
Parzi88334y@SortOfTested oh, that
i considered that but at the time that was implemented it was a bitch and a half to actually do since nothing was clear-cut and shit was being changed a lot because the docs were written by a 14 year old based on a CPU only partially made in minecraft, i'll prolly add that though yes -
@SortOfTested ewww....
definitely not a fan of weakly typed elements. Not to mention ninja-typed ones (when you don't see what/who/how many are coming) -
Parzi88334y@netikras i can read it fine, i'm just amazed you typed out code from your monitor into devrant because if you copy-pasted it you wouldnt've missed an `elif` (edit i just noticed you swapped the fail and success return values too)
anyways
i would very much like to not look up callback functions because i had to do that once in a python class i took just so i could pass things to a function called by pressing a button in tkinter and it looks so much worse and it fucking hurts me physically just thinking about it
you're right on the input handler part tho and i'd assume you could just str() the int keys? -
@netikras
Me either, I was pulling lines out of his code.and normalizing to keep it as simple as possible. -
@Parzi I copy-pasted a couple of the first "if" cases to show you the point :) No need to trash dR's comments with excessive code blocks ;)
well, suit yourself. I for one would definitely use functions as dict/map values in this use-case :) -
Parzi88334y@netikras fair enough, but also god no on the func part because that also means every command on the interpreter becomes its own func i have to specify globals in because why default to globals if they're defined already?
-
@Parzi
> edit i just noticed you swapped the fail and success return values too
Mea culpa, apologies. I'm a bit too distracted with my work tasks :) working on 4 tasks ATM + dR + a kiddo running around... typos happen :) -
Parzi88334y@netikras nah you're good i'm just tired as shit and missed it, it's 3:40AM for me atm
-
Parzi88334y@sbiewald just noticed when rereading this, when you were talking about the ROM loader, you were talking about not naming things "byte" if they're not bytes but uh
they are bytes, they're just 1 byte in a str instead of a bytes() object for ease of decoding (read: my sanity) -
Parzi88334y@netikras turns out i'm stupid and a function dictionary is a lot easier than I thought it'd be (and tried to do it in the past) https://stackoverflow.com/questions...
might do that since it's not bad according to this
If anyone has like an hour or so free at some point, I would appreciate help with optimizing some code. https://github.com/ParzivalWolfram/...
(also i have to write docs on this conceptual CPU because the actual docs are a massive shitshow and this was compiled over like weeks of asking the dude who made it questions)
rant