Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
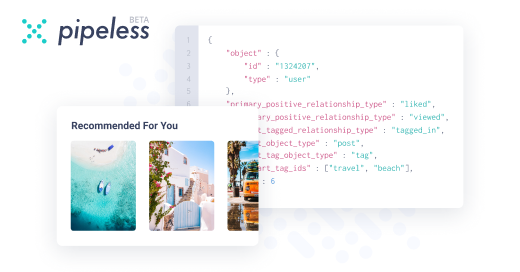
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Related Rants
package main
// go is very frustrating. in their efforts to keep the language simple, they've broken its consistency :(
// A A is just some arbitrary interface
type A interface {
Foo()
}
// B is an interface requiring a function that returns an A
type B interface {
Bar() A
}
// Aimpl implements A
type Aimpl struct{}
// Foo is Aimpl's implementation of A
func (a Aimpl) Foo() {}
// Bimpl attempts to implement B
type Bimpl struct{}
// Bar is Bimpl's attempt at implementing B.
// problem is, if Bar returns an Aimpl instead of A, the interface is not satisfied
// this is because Go doesn't support implicit upcasting on returns from interfaced objects.
// if we were to simply change the declared return type of Bar() to 'A', without changing
// the returned value, Bimpl will satisfy B.
func (b Bimpl) Bar() Aimpl {
return Aimpl{}
}
var _ B = Bimpl{}
func main() {
}
undefined
go