Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
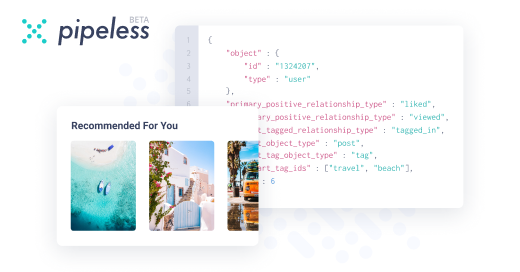
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
TIL python list variable asignment points to the original instance
I know this isn't reddit but today I noticed that in python when you asign a list variable to another variable in python, any change to the new variable affects the original one. To copy one you could asign a slice or use methods returning the list:
l = [1,2,3,4]
l2 = l
l2.append(5)
print(l)
#Outputs [1,2,3,4,5]
l3 = list(l2) # also works with l2[:]
l3.append(6)
print("l2 = ", l2, "\nl3 = ", l3)
#Outputs l2 = [1,2,3,4,5]
# l3 = [1,2,3,4,5,6]
I wonder how the fuck I haven't encountered any bug when using lists while doing this. I guess I'm lucky I haven't used lists that way (which is strange, I know). I guess I still have a long way to go.
undefined