Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
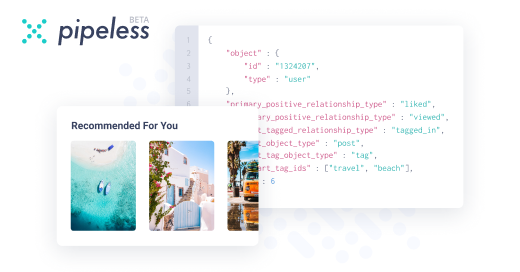
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
#include <ctime>
#include <vector>
int main()
{
std::srand( std::time(NULL) );
std::vector<char> arr( std::rand() );
return 0;
}
could work. srand sets the seed for the rand random number generator to the result of time(NULL), which returns the milliseconds from some time in 1970 - eg changes every milisecond - and vector is a class for dynamic arrays. a vector allocates a memory range on the heap(RAM) and stores a pointer to it. you could just aswell allocate an array at runtime yourself using
char* arr = new char[rand()];
which leaves you with a pointer to the beginning of an array which is sizeof(char)*rand() bytes long.
hopefully this helped -
Binary747yYou can use this compile time random number generator: https://youtu.be/rpn_5Mrrxf8 which returns constexpr number which can be used to initialize the size of an array
-
@funvengeance
std::array holds a static array, declared like
Type name[const_size];
In C++ you are often concerned with performance and optimization and therefore the to-go approach for arrays are fixed size arrays. Resizable arrays must always be ready to change their size, which means you may have to copy the entire array to another place in memory which is guaranteed to be large enough to hold the new size. -
int[] myRandomLengthArray = {1, 2, 3, 4};
(Four was chosen randomly by a fair dice roll)
Related Rants
-
xjose97x20Just saw a variable in C named like this: long time_ago; //in a galaxy far away I laughed no stop.
-
Unskipp24So this happened last night... Gf: my favorite bra is not fitting me anymore Me: get a new one ? Gf: but it ...
-
sam966911
Hats off to this lady .... I would have just flipped the machine
they say "you can do anything on C++"
I can't even initialize an array with a random length
rant
c++
cpp