Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
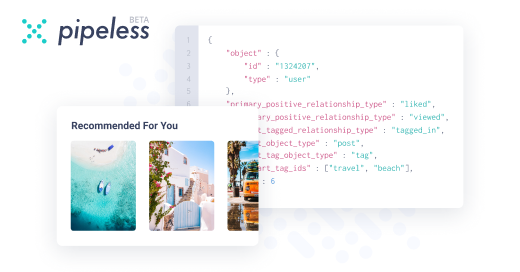
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
hexc11167y@martikyan everything I find is binary search trees, or code review examples that have a bunch of people saying there are memory leaks etc.
-
BigBoo23037y@hexc What part do you have problems with implementing?
This is a fairly simple thing but I can help you out if you need help. I will not just give you working code though. -
hexc11167y@BigBoo in most programming languages, you can make a simple tree by just defining nodes and attaching them through methods defined in the node class, because most languages memory is managed and the data member for parent and children are references, it makes it really easy to use/implement. In CPP, I'm not sure how you could get the same ease of use / flexibility, unless you define all nodes on the heap using "new" and are suuuper carfull when you need to clean them up with delete. The other option is to have a 2nd "tree" class that contains the references to the nodes, which makes clean up easy but then you have to worry about another class to deal with which makes it less user-friendly. I was thinking of possibly making it so each node that doesn't have a parent holds all the nodes in that tree and if a node is removed, it's give it's references and they are removed from the old root but this is really awkward to code(but more user-friendly).
-
BigBoo23037y@hexc
You can just create a node class which has the following
class Node {
private:
Node * parent;
std::vector<Node> children;
public:
Node(Node parent){this->parent = parent}
Node(){this->parent = nullptr;}
addNode(Node child){child.parent = this; children.push_back(child);}
};
Now, this might not be perfect. I just wrote this on my phone and I've been awake for like 10 minutes π
But vector is like arraylist in Java. it's memory managed for you. However, it will take up space on the heap for it's current capacity.
This is usually nothing to worry about unless you have huge nodes.
Then you could read up about making a vector of unique pointers.
Unique pointers are pointers you can't share but they are memory managed for you.
I hope this helps in any way. -
BigBoo23037y@hexc No it doesn't.
Why would it? It's not inside the vector.
And if it would. Just set all children's parent after each add. -
BigBoo23037y@hexc you can also use smart pointers if you want to allocate them individually.
So that each element is memory managed.
I guess shared is more appropriate than unique when you need a parent. -
BigBoo23037y@hexc Sorry for spamming you.
I got what you meant after thinking a while.
But you could also use reserve the vector with as many spaces as you need if you know beforehand. Like if a node never has more than 32 children.
children.reserve(32);
In the constructor does the trick. -
BigBoo23037y@hexc Hm. I've never used it to get a pointer for this. It might be so that the grandparent has to tell the child who the parent is.
If you catch my drift.
Related Rants
-
xjose97x20Just saw a variable in C named like this: long time_ago; //in a galaxy far away I laughed no stop.
-
Unskipp24So this happened last night... Gf: my favorite bra is not fitting me anymore Me: get a new one ? Gf: but it ...
-
sam966911
Hats off to this lady .... I would have just flipped the machine
Is there a simple CPP equivalent to a tree node in Java that has a parent and a arraylist of children? Or will I have to write out a 500+ line class to get the same functionality? π€
question
c++
cpp