Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
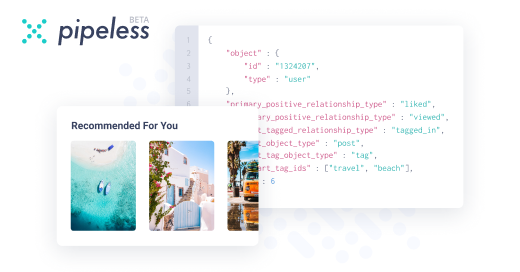
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
While I love PHP, it allows doing some dirty things like for example
$array = [1,2,3]
foreach($array AS $item) {/*whatever*/}
echo $item; //outputs 3
Its matter of that PHP is not "forgetting" variables unless the entire scope of sth like function changes.
But it has actual uses. Consider this example:
file 1:
$foo = "hello world";
require_once "template.php"
file template.php:
<h1><?=$foo?></h1>
(without this it would be literally impossible to do clean templates in pure PHP) -
inaba45665y@DubbaThony It doesn't have any actual use cases though. You example shows proper scoping in action since, as pr the docs: _When a file is included, the code it contains inherits the variable scope of the line on which the include occurs._
-
@inaba
Yes, but that's consequence of this behaviour - remember that in PHP every include/require is added onto stack. -
SomeNone7105yA language with no scope is a WTF in itself, it's so… 70's…
Regarding the if statement: that's one reason I like functional languages where if is just an expression returning a result, and you can use the following:
let ret = if sth then 1 else 2 -
kamen69165yJust declare the var outside of the if (before it's written to) and go think of more serious problems.
-
Root795845y@DubbaThony Ugh. I get why, but that is so dirty.
I prefer Ruby's approach where you use instance vars on the controller's instance. The view is a child of that instance, so it has access, and partial templates are grandchildren so they have access to both scopes. I'm simplifying it a bit (the data is copied rather than shared), but it's a very clean OOP approach. -
@kamen lol i know, i just daily do php and had to do some JS. In php you dont have to declare variable (actually my IDE would throw at my face unused variable - overwritten before used) so that felt natural to me thats how i ended up here.
-
inaba45665y@DubbaThony You should read your example again. You declare the variable foo, then, and this here is important, _you import the file you use foo in in the same scope you declared foo in_. It is not a consequence as it is stated in the documentation. Again, from the docs:
> When a file is included, the code it contains inherits the variable scope of the line on which the include occurs. Any variables available at that line in the calling file will be available within the called file, from that point forward. However, all functions and classes defined in the included file have the global scope.
https://php.net/manual/en/... -
@inaba
Fair enough. Im pointing out that if you call file and it throws exception for example and you have xdebug for example you will clearly see that stack depth incerased from this inclusion. -
inaba45665y@DubbaThony Yes that is exactly what calling a function does but it has nothing to do with what you're talking about and it doesn't demonstrate whatever side effect you think might be happening.
-
-
@inaba
I mean php has some non-standard scoping. In meaning, if you call function, ofc you dont derrive scope, but when you include file, its internally treated one way or another like function call, but derrives scope.
Its in generall mess but imho it makes sense and I can justify it. But i like strict languages to point of beeing obscure, thats why i created this rant.
Idk which language allowed you this but i loved it:
//pseudocode
int a = 1, b=1;
{ //scope from nowhere. Becouse you can and its valid.
int a = 2;
Echo a, b; //21
}
echo a; // 1
Not really usefull but intriguing and strict as sh**t. -
inaba45665y@DubbaThony > In meaning, if you call function, ofc you dont derrive scope, but when you include file, its internally treated one way or another like function call, but derrives scope.
You're stating this like it's something surprising when, again, this is exactly what the documentation says happens. And regarding your example, none of the languages I've used (barring php) would allow you to do that because you can't redeclare an already declared variable. -
I don't like in php that it throws error when some variable doesn't exists. In javascript you get undefined. You need to check for availability with isset function in php.
-
inaba45665y@Pogromist If a variable doesn't exist in JS you get a ReferenceError thrown. If it has only been declared you get undefined
-
inaba45665y@Pogromist in javascripts arrays are basically just objects wiht a length property and then number properties with their __proto__ set to Array.prototype (and of course a little bit more syntactic sugar) IE
const arr = { length: 2, "0": "Foo", "1": "Bar", "__proto__": Array.prototype };
And since accessing properties with [] gives you undefined you get undefined when something doesn't exist. This is why you can also do
const arr = [];
arr["foo"] = "bar"
console.log(arr.foo)
Bascially, but ofc there's a lot more to arrays than just that. None of it really that important for every day use tbh
Related Rants
Today I discovered first thing I personally prefer in JS over PHP.
let's say you have:
//pseudocode
if(sth){
let ret = 1;
} else {
let ret = 2;
}
if(sth_else){
return ret.toPrecision(2);
}
return ret.toPrecision(8);
In PHP this will work. JS will throw up becouse it managed to separate scopes. Its actually what I would like to be the case with PHP.
rant
php
js