Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
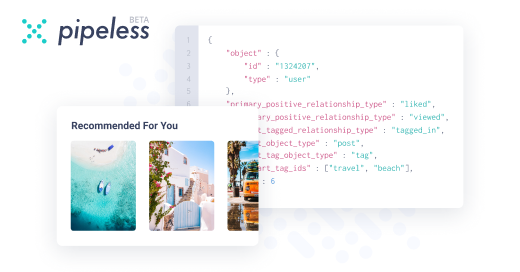
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Related Rants
How deep do you go when trying to find a solution?
I have a need for combinations of items. I have used built in functions in Python for this. When I first used those I wanted to learn how they worked internally. I read through the source and thought that was cool. I don't think I really understood that code very well.
Now I need the same solution in C++. There is not a prebuilt combinations function in C++. There is a prebuilt verion of next_permutation. I can build upon that to make my combinations code. However, I am in the middle of trying to make something work. So I found this nice SO question:
https://stackoverflow.com/questions...
The code I ended up using:
template<class RandIt, class Compare>
bool next_k_permutation(RandIt first, RandIt mid, RandIt last, Compare comp){
std::sort(mid, last, std::bind(comp, std::placeholders::_2, std::placeholders::_1));
return std::next_permutation(first, last, comp);
}
template<class BiDiIt, class Compare>
bool next_combination(BiDiIt first, BiDiIt mid, BiDiIt last, Compare comp)
{
bool result;
do{
result = next_k_permutation(first, mid, last, comp);
}while(std::adjacent_find(first, mid, std::bind(comp, std::placeholders::_2, std::placeholders::_1)) != mid);
return result;
}
I am mostly able to figure out what is going on with the templates. I still am not understanding the basic algo behind permutations.
Our data set is tiny. 4 items max. So efficiency isn't really a big issue here.
How long do you spend learning how it works versus just finding a solution for the task at hand?
In general I need to spend more time learning different kinds of algorithms. So I should probably add permutations to that list of ones to study.
question
learning
algorithm