Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
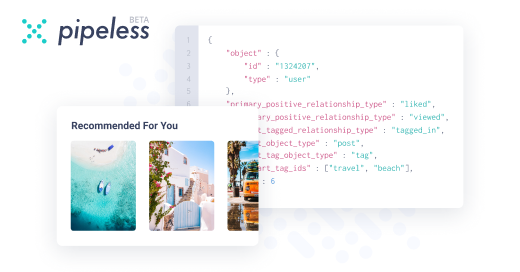
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
And before any comments it:
yes I know you can put [[nodiscard]] on a struct/class as well.
But you can't do that with a typedef or using, so you have to define a dedicated struct/class that wraps around the value that you want to actually return.
And I think that's a load of bullshit -
It seems to be designed to only be in the context of a return value. So I can see why it would not make sense in a typedef. Its a compile warning flag, not actually something that affects the definition of the type.
using RetType = struct {/*blah*/};
RetType [[nodiscard]] funct(); // wouldn't this work?
For reference:
https://en.cppreference.com/w/cpp/... -
@Demolishun normally you would just put it on the function, but this does not work for functions inside template classes.
https://stackoverflow.com/questions...
the other option is to put the nodiscard on the data type that you return, aka
struct [[nodiscard]] MyRetType {...}
MyRetType someFunction()
But that required me to always use custom structs/classes, which would then also change the way I access the values and may add overhead etc.
the typedef/using thing I mentioned was one of my first attempts, I thought I might get away with something like this
using NodiscardBool = [[nodiscard]] bool;
NodiscardBool myFunction();
but that caused a warning saying the attribute is ignored.
The nodiscard feature is a great idea but I think this is a "good plan - horrible execution" situation. -
I think that instead of "nodiscard" a better solution would have been to add a compiler flag to show a warning for any discarded return values.
Then either add a "discard" as opt in, or provide a syntax for the call side to explicitly mark that returned value as discarded to silence the warning. -
@Lensflare I think C used to do that, which is why sometimes you see people casting functions to void in order to suppress the warning
(void)myFunction(); -
@LotsOfCaffeine That warning is not necessary in terms of the C standard - it may be due to whatever code checker you run over the source.
That said, I like the (void) idiom to make clear that I know there's a return value, but decided to ignore it. -
I think I’ve learned my lesson after working with it and OpenGL.
Very much happy with Swift, thanks. -
YADU13963y
Related Rants
-
xjose97x19Just saw a variable in C named like this: long time_ago; //in a galaxy far away I laughed no stop.
-
Unskipp24So this happened last night... Gf: my favorite bra is not fitting me anymore Me: get a new one ? Gf: but it ...
-
sam966911
Hats off to this lady .... I would have just flipped the machine
I am once again reminded how much of a clusterfuck C++ as a whole is.
They recently (C++17) added this cool new attribute called nodiscard.
You can put it on a function like this
[[nodiscard]] bool writeMessage(...)
and the compiler will check if the returned value was discarded or not, and give a warning accordingly.
Pretty neat if you're returning an error code and you want to enforce that it gets checked, right?
Except it doesn't work in template classes.
It just doesn't do anything there.
rant
templates
c++
nodiscard