Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
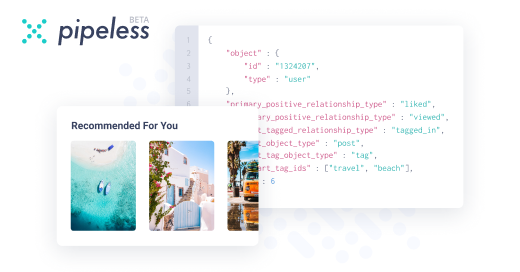
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
sgoel015978yBeing a software engineer, that's important. You should know how machines work, because it is very close to that. I suggest you to take the hard way and learn about the memory architecture. How data is stored and retrieved. Pointer would be an easy topic then.
-
Let's say I have a number A and A is 5. A is going to be located in random space in memory, that, quite frankly, I don't care about. A pointer to A is not a number, it simply points to the area in memory where A is stored. Which means I can do the following
int A = 5; //create a number
int *B; //create a pointer to a number
B = &A; //assign the location of A to B
Now B holds the location of A. Which means B directly points to A, whatever you do to B happens to A and whatever you do to A happens to B.
However B just points to A, in order to use it, I need to reference it.
*B = 6;
*B tells the compiler I want to access the memory spot of B, which, in this case, is A.
So now if you were to print out A, it would be 6. If you printed, B , it would give you the location in memory, and if you printed *B, it would give you whatever's stored in RAM at B, in this case, A, or 6. -
I picked up "Understanding and Using C Pointers: Core Techniques for Memory Management" by Richard M Reese. It's a short book on pointers that I used as a refresher.
-
Every variable value is stored in specific block of memory. The block is represented as memory address. Pointer is a variable that points to the memory address of another variable.
-
hisetip8168y@rainmitchell thank you!! I can't tell how much I've searched in books and online but all the explanations were too abstract and long. Thanks, really
-
hisetip8168yProps to everyone that helped. I'm studying computer science and this subject is computers architecture and i was really lost with the difference between int* or int (for example) but now i get it! Cheers people
-
Even in Java everything is a pointer, it just doesn't expose it. A pointer literally just points to a location in memory. When you increment the pointer it points to the next position in memory. The offset is based on the data type you are using. For example a 32 bit integer would jump 4 bytes, if the pointer is a char pointer, it jumps just 1 byte.
You can do all kinds of neat tricks too.
For example, an IPV4 address is actually a 32-bit integer when you receive it. But you want the octets of the ip. So you can do the following:
int ipAddress = 3232235801;
unsigned char* octets = &ipAddress;
Now you can iterate through the octets. But because of little endian the octets will be in reverse. -
@LicensedCrime he also could start with Vala, which supports pointers as well and is closer to Java (what he apparently was using before)
-
willol13898yYou don't know it but pointers are EVERYWHERE. In Python, in Java, in PHP, in JavaScript... It's hidden but if there's OOP, there's pointers. Mutability, parameters by reference, dynamic typing, callbacks... It's all about pointers.
Knowing about pointers and how to use them is a huge step forward a better understanding of programming, you'll be able to recognize magic in languages and quickly understand and correct some bugs in most languages
I can't understand C! I can't understand pointers, I'm lost!!
undefined
love java
studying computer science