Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
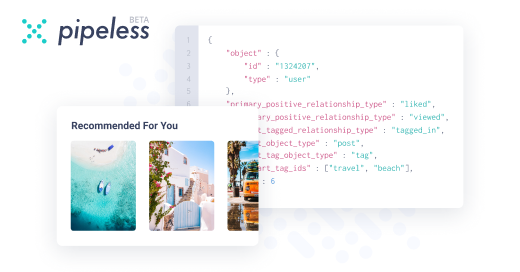
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
umnikos9268yIkr! Exactly my point yet some people managed to present me problems that are better done with recursion...
-
++ for memes in communication, -- for saying that recursion is not useful at all! Try to search in a tree without recursion. You will have to use a stack and things will get messy.
The algorithm to solve the Hanoi puzzle takes about 3 lines of code!
# a, b, c are the stacks of rings
# a is the source, c the destination
# and b is used as a helper
def hanoi(a, b, c):
if not empty(a):
hanoi(a[1:], c, b)
move(top(a), c)
hanoi(b, a, c)
Now try to solve it with for loops. I assure you that the solution will be so much more complex!
And, like that, there are numerous problems that are easier solved with recursion. -
Disclaimer: the pseudo code in the previous comment is not totally correct, but it gives you an idea of the algorithm
-
dootdoot1438y@Joserc87 good for academical purposes, in years programming for loops >> recursion (business logic). No client will give you extra points for solving something recursively
-
@dootdoot no, they won't give you extra points for using recursive algorithms. They will give you extra points for finishing earlier. They will give you extra points for cleaner code. They will give you extra points for having less bugs.
Recursive algorithms are not something theorical, they are very real and implemented everywhere in systems in production. Some problems are much more approachable in a recursive way, and the only reason to mess with loops and stacks is that the developer hasn't learned the recursive mindset.
What exactly do you have against recursion? -
dootdoot1438y@Joserc87 recursion doesn't help readability at all. Yes it is brief, but brief != readability
-
@dootdoot I completely disagree. To me, recursion is very readable (when done right of course). You just have to know how to think in a recursive way and get used to it.
Do you have an example? -
bioDan55608y@Joserc87 I agree with most of what you say, but regarding the "readability issue", I think @dootdoot has a point.
I mean, for a non-programmer or a novice programmer procedural code is much easier to understand than recursive code, you said it yourself.
Therefore given the same syntax and descriptive naming of variables, functions and operations - procedural code is less complex to understand and thus more readable. -
@bioDan Yes, but that is only applicable to those who doesn't have an understanding of recursivity. Recursive code is usually more complex than most regular, procedural code. What I'm saying is that recursive code is simpler and more readable than THE EQUIVALENT procedural code.
Take the algorithm to search in a tree as an example. The recursive code is simple and readable. The procedural EQUIVALENT code involves a stack where we store the nodes that we have been visiting plus a while (not a for) that loops while the stack is not empty, a nested loop, etc. Chances are that a non-programer or novice can't understand the procedural version either ;) -
Besides, I think code like this is very readable (regardless of whether you think it's complex), because it almost reads like english:
Search(node, x):
if node.value:
return node
else
for child in node:
found = search(child, x)
if found:
return child
else:
return None
It reads like: "if the node contains x then return that node, otherwise search in the children". To me, the recursive description is so much easier to understand and definitely much more readable than the procedural version (which I can't type here) -
bioDan55608y@Joserc87 I agree with what you are saying, and I also love and am fascinated with recursion both in code and in nature. Also ofcourse there are cases which recursiveness is the preferred way to go.
But let me give you a personal real life example regarding recursion and readability.
My last workplace has a large codebase, i had to tdecouple advanced search options from minified variables and make sense out of them while they kept polymorphing themselves in a bounch of recursive functions.
I can't recall the procedures themselves, I just remember that after about 2 hours of trying to make sense of it, I removed the code and I spent another 4 hours rebuilding it myself.
(But I also used recursion :) )
!rant example of effective cross-language communication. A Drake meme can save up to 140 characters on avg.
undefined