Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
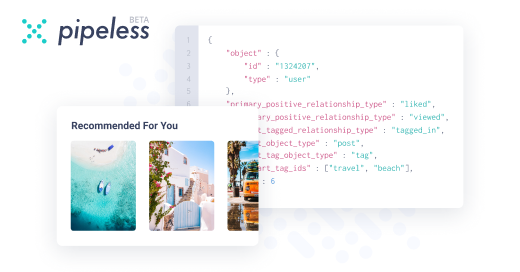
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
You don't use cacheeevict in write operations. You use cacheput.
That runs the write in both the cache and the rds.
Still, not everything is meant to be cached. Only cache frequent read/infrequent write data that you can live with being outdated, such as comments, etc.
Never for application critical info such as user credentials, etc. -
b2plane62952y@CoreFusionX hmm... Thats kind of difficult to determine when should i use it. the comments part makes sense. But what if i post this comment on devrant and immediately i realized i made a typo so i edit the comment. In that case the app would still show the cached comment (with the typo) instead of the updated one, right? So my question still remains the same even in such a scenario like that, how am i supposed to know when was entity updated so i can fetch it from postgres and not redis cache?
Also what about chat apps? Some people suggest using redis over kafka and other technologies to create a chat app. Taking into consideration what u just explained "redis should be used for infrequent data" means it should never be used for chat apps? Cause they are literally the most frequently changing things ever -
b2plane62952y@CoreFusionX the only solution i can think of so far is to manually check with the if statement if user.updated (bool), and if so then i fetch data from postgres, otherwise return data from redis cache and set the bool back to false. Is this even acceptable way to control it?
-
@b2plane
To put it simply. You don't. You have no way to know if the cache is up to date unless you query both cache and origin, which kinda defeats the purpose to begin with.
Hence why I said you use it with infrequently *written* data.
As for the second part, redis is a software on its own. Using it as a cache for a rds is just one of its multiple uses.
Of course you can create a chat app using redis, which you would do with the pub/sub mechanism. -
b2plane62952y@CoreFusionX imagine if there are millions of chat messages. How would that work with redis without causing memory heap overflow since redis is an in-memory database?
-
@b2plane
Because pub sub does not save what is published. It just relays to the subscribers.
Please go read the redis docs on pub/sub. -
b2plane62952y@CoreFusionX ...in that case why dont i just use socket.io? It solely works on event/emitter pub/sub mechanism and is much more simpler than any of them
-
@b2plane the millions of messages thing:
you can partition the stuff into active messages and archived.
that way the cache can be applied in a simple way, and you don't need to care about the specifics. If you want to move a message out of archive to edit it, put it in active and override the message in frontend/view
There are lots of ways to get performance out of a cache, without needing whacky invalidation stuff. -
@b2plane
A) you don't use socket.io because it's cancer for people who refuse to deal with real sockets, but unfortunately for them, the rest of the world is built on real sockets.
B) socket.io does not offer ACL, or other configuration options if you don't roll them on your own.
C) related to A, socket.io adds a non negligible amount of overhead over every message, which is noticeable once things start scaling in volume.
D) socket.Io does not offer clustering, again, unless you roll your own, etc. -
b2plane62952y@CoreFusionX how many users do you think socket.io can handle?
I just found a github example implementing chat app using socket.io with spring boot and react. It is exactly what i needed and it works perfectly fine and fast. Is it fine to use this piece of example at least for the v1 of the production web app that I'm building?
I am new to redis and confused how this works
To keep it simple lets say i have a CRUD service for user
- POST user, just creates user
- GET user by id, fetches user but using annotation @CacheEvict(). This method has a Thread.sleep(3000) before fetching user
- GET all users, fetches all users but using annotation @Cacheable()
- PUT user by id, updates a single with annotation @CacheEvict(). This method has a Thread.sleep(3000) before fetching user
- DELETE user by id, deletes a single user with annotation @CacheEvict()
---
GOOD:
When i GET user by id, i wait 3 seconds and then get the fetched user
When i GET user by id again, i get the fetched user instantly in 5 ms. This means it has pulled the user from REDIS cache instead of postgres
---
PROBLEM:
If i PUT user by id, update some data, and then if i GET user by id, it will return the user in 5 ms BUT the outdated user! Not the newly updated one. Because the Redis cache configuration has not expired yet. So there are now data inconsistencies
---
QUESTION:
How can i know When was something updated, deleted or whatever, so that i can fetch data from postgres (latest data) instead of Redis cache (outdated data)?
rant