Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
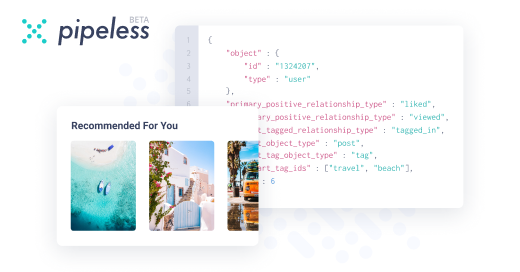
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
PaperTrail10604307dI wish these contrived tests that knowingly 'exploit' a perceived flaw would be abolished.
If you're writing a 1 to a million loop in your code, complaining about <1 second perf difference, and then 'fix' the code with 50+ nested/convoluted 'if' statements because it's 'faster', please stay out of our code base.
I know..I know...upper mgmt is too stupid to tell the difference and when you brag "Look at me! I increased performance by 50%!", you get awards, new cars, praise and worship...yadda..yadda..yadda.
No thank you. -
kiki35793307d@PaperTrail it's not that "every single mess of ifs is faster than every single switch", it's that every switch can be replaced by a flat sequence of ifs that will be 1. faster 2. more readable (minus two lines of code, minus one indentation level)
-
kiki35793307dHonestly, I wouldn't have cared about speed in this context at all — if you need that many ifs or switches, you have other problems — but small people keep bringing speed up when defending switches.
-
cafecortado7998307dAt first I thought the dot in "949.524ms" was the thousands separator. I was starting to worry a little.
-
PaperTrail10604307d@kiki > " 1. faster 2. more readable"
Falls into the "it depends" bucket. Faster is relative. I'll take real world performance analytics over a long period of time versus a single 1-to-million "for .. loop" test contrived to sell ice water to Eskimos.
Readability is another area of relativity. What's readable to me could be very different for someone else.
I agree 100% if someone wrote a long switch statement for a couple of values where a simple if..then..else would do the same job, I would call replacing the 'switch' a win. The micro-optimization of performance is a single sprinkle on the cake.
For me, I usually see long, (10, 15, 20+) switch values, all with specific (and sometimes duplicate) behavior being executed. Didn't start out that way, but falls prey to the "just one more" feature creep monster. -
Demolishun35787307dIsn't the power of a switch statement is that it is useful for complex logic that does not have a 1:1 condition to outcome? That is why there is a fall through mechanism using break.
-
Demolishun35787307d@shovethisrant yeah, they are kinda fun. I found one SO post that shows that lookups were faster than both if and switch. But that was from 2011. Pretty sure JS has changed a lot since then.
-
shovethisrant5988307d@Demolishun I think the point is just seeing how switch performs in a wild JavaScript benchmark. In other languages in some situations, I know switch can outperform if statements. I’m sure someone could easily find a test where switch is more performant than the if statement in JavaScript. But 🤷♂️.
-
Demolishun35787307d@shovethisrant I don't worry about it much in C++. They all compile to jump statements anyway.
-
Demolishun35787307d@shovethisrant I have an environment that runs JS for the GUI and communicates to a C++ layer. I had some code that needed to process 4000 items every second in a JS data structure. It was way too slow. So I made a C++ callable function to process the data. It was way faster. I avoid processing things of any appreciable size in JS now. I know web devs don't have the luxury. They don't right? Maybe push it to the server?
-
shovethisrant5988307d@Demolishun in that case I definitely attempt to convince people that we should handle as many tasks (where it makes sense) on the server as possible, but often met with resistance of 1) BE devs who don’t want to do the work bc it just “works” as is and 2) managers/leads saying we don’t have time to do it.
So we end up pushing out a slow piece of shit most of the time ☺️ -
kiki35793307d@Demolishun no, we have it now, using WASM. I can compile your C++ thing and call it from my JS in the browser
-
kiki35793307d@shovethisrant so find it! Otherwise no, switch is never faster than ifs in JS in 2024.
-
Lensflare18075306dThat‘s like arguing about the aerodynamic properties of different toy cars. Who cares? Those are freaking toy cars!
-
PaperTrail10604306d@Lensflare > "That‘s like arguing about the aerodynamic properties of different toy cars."
We (as a company) likely spent millions on making the toy cars roll down the slide 'faster'.
Funny/sad/not sure, other mgrs are recently unraveling some of the complexity asking "Why in the world was *this* done?"
All I can do is "Gather around the campfire kids, grandpa Papertrail has a history lesson." -
AlgoRythm50271306dResult doesn't surprise me, control flow in switch is more complicated than in if statements. I bet the extra tokens make the bytecode larger, which might result in more CPU cache usage, which might result in more RAM reading.
Additionally, switch is used less and therefore likely optimized less by the JS runtime.
Speaking of - which runtime is it? -
kiki35793306d@AlgoRythm latest node. In latest chrome everything is the same except switch is even slower at 1112 ms.
-
AlgoRythm50271306d@kiki Weird, I thought chrome and node both shared the v8 runtime. Maybe the node version is heavily modded.
-
CoreFusionX3617306dCan't really benchmark just running stuff once anyway. There are oh so many external factors that could skew results...
But in any case, if performance was ever a target, JS was never the right choice to begin with. -
Demolishun35787306dconst ifs = i => {
if (i === 1)
return 1
else if (i === 2)
return 2
else if (i === 3)
return 3
else if (i === 4)
return 4
else if (i === 5)
return 5
else if (i === 6)
return 6
else if (i === 7)
return 7
else if (i === 8)
return 8
else if (i === 9)
return 9
else if (i === 10)
return 10
else
return -1
}
const switches = i => {
switch (i) {
case 1:
return 1
case 2:
return 2
case 3:
return 3
case 4:
return 4
case 5:
return 5
case 6:
return 6
case 7:
return 7
case 8:
return 8
case 9:
return 9
case 10:
return 10
default:
return -1
}
} -
Demolishun35787306d@Demolishun
var loops = 1000000000
const doifs = () => {
console.time('time')
for (let i = 0; i < loops; i++){
ifs(i)
}
console.timeEnd('time')
}
const doswitches = () => {
console.time('time')
for (let i = 0; i < loops; i++){
switches(i)
}
console.timeEnd('time')
}
if(0){
console.log("switches first")
doswitches()
doifs()
}else{
console.log("ifs first")
doifs()
doswitches()
}
// more conditions makes the ifs take way longer
// ifs 11187ms
// switches 1499ms
const ifs = i => {
if (i === 1) return '2'
// (a lot more ifs)
}
const switches = i => {
switch (i) {
case 1: return '2'
// (a lot more cases)
}
}
console.time('time')
for (let i = 0; i < 1_000_000_000; i++) ifs(i)
console.timeEnd('time')
// time: 637ms
console.time('time')
for (let i = 0; i < 1_000_000_000; i++) switches(i)
console.timeEnd('time')
// time: 949.524ms
random
abolish switch