Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
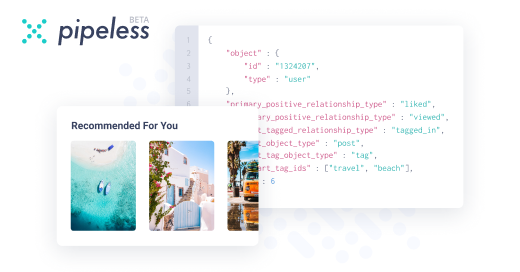
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
Error7072937y@Froot
that's the code for changing calendar date hehe ~~~ if the gif work, it will show that boy changed his calendar date and the deadline reaper go back from the start
Related Rants
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <time.h>
#include <sys/time.h>
void setDate(const char* dataStr) // format like MMDDYY
{
char buf[3] = {0};
strncpy(buf, dataStr + 0, 2);
unsigned short month = atoi(buf);
strncpy(buf, dataStr + 2, 2);
unsigned short day = atoi(buf);
strncpy(buf, dataStr + 4, 2);
unsigned short year = atoi(buf);
time_t mytime = time(0);
struct tm* tm_ptr = localtime(&mytime);
if (tm_ptr)
{
tm_ptr->tm_mon = month - 1;
tm_ptr->tm_mday = day;
tm_ptr->tm_year = year + (2000 - 1900);
const struct timeval tv = {mktime(tm_ptr), 0};
settimeofday(&tv, 0);
}
}
int main(int argc, char** argv)
{
if (argc < 1)
{
printf("enter a date using the format MMDDYY\n");
return 1;
}
setDate(argv[1]);
return 0;
}
undefined
reset
c
deadline