Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
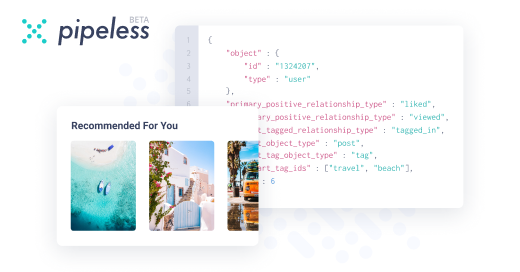
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
import java.security.MessageDigest;
MessageDigest messageDigest = MessageDigest.getInstance("SHA-256"); //or whatever you want
messageDigest.update(stringToEncrypt.getBytes());
String encryptedString = new String(messageDigest.digest()); -
@sharktits with that, 6 letter passwords take little time to crack from their hash.
That's why there's the PBKDF2 standard, to mitigate this issue.
The basic idea is to eliminate that weakness by making it take far longer to compute the checksum, without losing entropy (which you would get by hashing the hash of the hash ... ). It's crazy simple, and goes only a bit over the basic usage of SHA. -
@sharktits
1. Hashing is very different from encryption.
2. SHA-256 is extremely insecure for passwords (far too fast to compute).
BCrypt/Argon2/Scrypt would be far more appropriate for passwords. -
@AndSoWeCode hashing is not there to be uncrackable, its there to dont store plaintext passwords.
-
@systemctl literally the first line of this post is 'hashing passwords atm' which part do you fail to understand
-
@systemctl I've been reading about BCrypt/Scrypt comparison, and without going into too much detail, my conclusion was that one was extremely easy to pick on ASICs, and the other one was extremely easy to pick on computers. Because they rely on algorithm quirks, rather than brute slowness by doing the same thing over and over and over and over and over and over again.
From my limited understanding, PBKDF2 is the simplest, fool-proof, universally slow and secure method.
But then again, that might be just cognitive ease talking to me. -
@sharktits (Cryptographic) Hashing was created, to make it extremely hard to get back the byte sequence used to create the hash. It wasn't made to not store passwords in plaintext, as they wouldn't be in plaontext when the Caesar Cypher (rot (13, but it doesn't have to be always 13)) would be applied, or when the password would be properly encrypted (properly = AES/Serpent/RC6/RSA/EC/Threefish, etc), but these methods would still be considered extremely insecure.
-
@sharktits it can be both.
Let's face it - if your system gets big, it will be eventually cracked, because the janitor decided to watch some porn from a flash drive he found in the parking lot, on a computer connected to the intranet. Unless you make it a police state, there's no way to 100% secure your company from breaches.
So start from that: your database will get breached if it lives long enough. What are you gonna do about it? Will you let your customers be in danger of their passwords (that they re-use everywhere) be stolen because you built it on some moral programming principle? Or will you use PBKDF2 and nobody loses anything? -
After talking so long about algos...please don't forget using a user-specific salt😅
-
@AndSoWeCode Scrypt is applied with different arguments for the algorithm (Passphrase, Salt (protect against rainbow table attacks), N (CPU/Memory cost), r (Add. Memory cost), p (Parallelization parameter), dkLen (Intended output length))
(Similar things for Argon2)
When computers get faster, these parameters just need to be incremented, instead of having to find a new hashing algorithm.
When a (bigger) user database get's breached, and the hashes of the passwords get leaked, it would be extremely costly to compute every single one of them, for both bcrypt and scrypt, although bcrypt doesn't have any additional parameters you can apply (afaik). If you are already using bcrypt (it's used a lot in PHP applications), it's not that bad to just stay using it to hash passwords. But when creating newer applications, it would be pretty good to use a password hashing algorithm, which you can supply parameters to, so that when cracking power increases, you can increase cracking cost too. -
sigfried5327y@sharktits I see your point. But you better learn it good thr first time. You don't wanna hash your passwords without salting.
Related Rants
-
dfox60
Hey everyone! As many of you have already seen, @trogus and I are happy to announce the release of devRant++, ...
-
linuxxx19Did a very tiny migration for a client which would normally be done against our hourly rate but decided to do ...
-
exploreTheC28Officially graduated with a bachelors in computer science. YYEEESSS!!!!
hashing passwords atm.
i have a java backend, should i look into bcrypt or just use a loop?
also how many times would you recommend i hash passwords?
and should i look into hardware acceleration?
question
thanks