Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
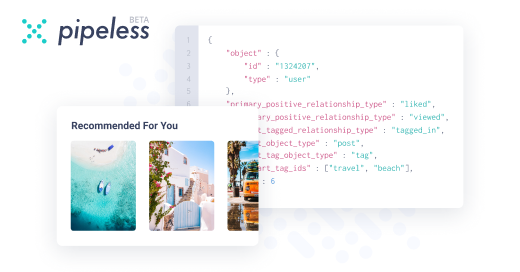
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
Ximidar8387yI would just keep my head down and try get through it. Some groups have a style guide, other's don't, but it is good practice to follow the style guide whether or not you agree with it. Attention to detail is one of the best skills you can learn, it will serve you well in coding and pretty much any other area in life. Also it's only a semester of work, after this class you can code any way you want.
-
Stop blaming the IDE - you are responsible for your code.
The order of header files is important because you may use stuff in your header files that is defined in system header files, e.g. uint32_t. Now if you include your header files first, then this doesn't work because upon encountering uint32_t, that has not been defined yet.
It becomes more annoying when you do the wrong order in several files and then add e.g. uint32_t in one of your header files. You'd have to fix the order in every single source file, which becomes work if you aren't on a toy project.
Students always complain when they screw up a bit and get deducted marks. Looks like the prof should have deducted even MORE marks to get the point across. -
@irene I want to see right in a file which system headers are pulled in, not hidden in some header file from who knows where.
-
It is nit picking, but it's getting you used to following a standard, aka something you're gonna have to do in the future.
-
@irene I've seen enough issues arise with multiple includes of system header files. Yeah, there should be include guards in them, but that's not always the case in reality, especially for embedded.
It gets worse if you accidentally have defines in your own headers that run into ifdefs in system headers with strange to find bugs. Imagine you have your headers A and then B. A includes system header file 1, B header file 2. Even with proper if-guards, the final include order will be 1-A-2-B. -
@irene well yeah, but once you run into such a thing, it's really nasty to debug especially if it compiles. By contrast, if I get some compile error in foo.c with undefined uint32_t, it's trivial to fix so that I prefer to run into that kind of error.
For embedded, proper header guards wouldn't cost a thing because the compiler runs on the host PC, and define/ifdef doesn't blow up the resulting binary. It's more that the vendors sometimes just don't care. Sometimes stuff is even missing at all - I remember a case where ctype.h was not there, shit didn't compile, and I had to add that myself. -
con-fig3907yIncludes go in the files that use them. This keeps your code modular, tidy and self-sustained.
-
Your instructor is almost right. Except for the 'namespace in the guard' thing. It's just plain nit picking. Include guards are per file, not per namespace, it's pretty common that different files base upon the same namespace (std in STDC++), and it can happen that one file bases upon more than one namespace (pretty rare, tho). The only requirement for guard names it's not duplicating, so...
-
fdgram4797yOrder of includes should be: cpp file's hpp, local headers, libraries, standard libraries. If file.hpp depends on iostream, it should include it itself.
Otherwise, including file.hpp will work in places that is using iostream (and includes it before file.hpp) but will fail in places that aren't using iostream, requiring you to include iostream in a place that doesn't need it (or do the correct thing and include iostream in file.hpp (and then there's no point in including iostream before file.hpp in the former case)).
Using the correct inclusion order above will prevent this accidental dependency injection as including file.hpp will always fail because iostream won't have been included yet -- forcing you to include iostream in file.hpp like you should. -
swiss-dev347y@Fast-Nop if the vendors don't care it's an issue with them and not a reason for general good practice.
Header files should include all the files needed but no more.
Related Rants
So for almost all of my c++ assignments I've recieved various emails from the instructor about things like "incorrect header guard" and "library inclusions out of order".
The first being that I didn't include the namespace inside of the guard (I did "FILENAME_H" instead of "NAMESPACE_FILENAME_H")
The second is that I accidentally included header files from my project before any of the standard libraries. This one wasn't even intentional, it was caused by vscode when it formatted/prettified the file.
EX:
#include "test.h"
#include <iostream>
In my opinion these seem pretty nitpicky and, especially that first one, appear to be more like naming conventions or best practices than something to deduct marks for.
On the flip side though I did accidentally store a couple functions in the global namespace which I understand isn't particularly safe. I also made a couple one line conditional statements that simply never evaluate to true, but I didn't think this was a huge deal.
I don't normally code in any of the c languages outside of college so I'm not sure how important these are to actually follow. I've apparently been deducted an entire 10 percent off the assignment because of the head guard. I know that every professor has different criteria for deducting marks, but even this seemed rather unnecessary.
What does everyone think?
question
header guard
c++
college
nitpick?
professor
email