Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
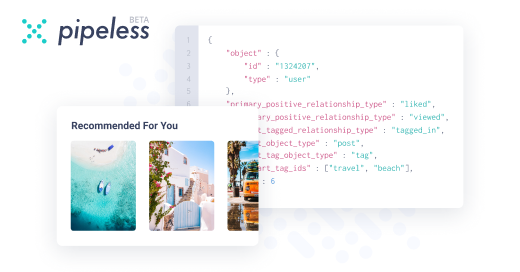
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
Bonus tip. The name is convention only. The double stars are the magic. A single star (*args by convention) is for ordered, unnamed arguments like [2,19,0] and a double star is for named arguments, usually as a dict!
Bonus bonus tip: you can do this to any function!
Def add(a, b):
Return a+b
Add(*[2,3])
Add(**{'a':2, 'b':3})
The more you know! -
@deadPix3l this, I just put in kwargs to make it easy to understand but now I see it can be misconstrued.😂
-
Ximidar8367y>>> def func1(foo, bar=False):
... print foo
... print bar
...
>>> func1(12, bar=True)
12
True
>>> args = [15]
>>> kwargs = {'bar':True}
>>> func1(*args, **kwargs)
15
True -
mahoraz567ywait... so its employing json style objects using C/C++ style pointers? Weird but interesting.
-
ltlian21307yCame to poke fun at keyed warts, but I'll toss in a couple "I wish I knew this sooner" python things as well while I'm here:
This style of deconstructing a dict also lets you union dicts. I had made a huge messy function doing it manually until I discovered you can just go 'both = {**first, **second}'
The * operator can be used as a "rest" when iterating over iterables with unknown or varying amounts of values to unpack. You can go 'for arg, *args in vargs', and 'args' in that scope will be a list with the rest of the unpackables. I've only used it for tuples so I can't vouch for other types. Maybe it works for ** too.
Related Rants
Python tip: some python functions have **kwarts (key-word) arguments, that means you can construct your parameter dictionary like so:
kwargs = { 'a':1, 'b':2}
And pass those arguments like so:
function(**kwargs)
random
python
dev tips
functions
kwargs