Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
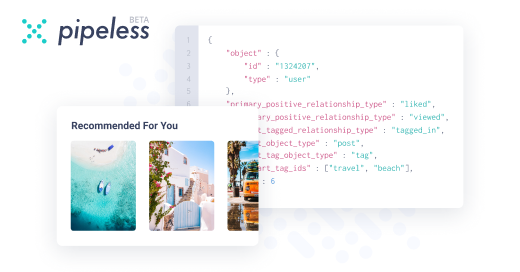
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
An array is one example where this is used, namely with the [] accessor:
int a[] = { 5, 6, 8 };
// with accessor
a[2] // == 8
// with pointer arithmetics
*(a+2) // == 8
I don't see why pointer arithmetics should not be "allowed", since it's a very fundamental and necessary thing of C and C++ and more widely used than you might expect.
Your last line has a tiny mistake, it's "int pp" not "int* pp" because you dereferenced your pointer in the right statement.
"pp" is 5555 because you declare and initialize the local variable "tt" right after "t", so the compiler will (only most likely!) put "tt" in the next higher memory address after "t" (don't forget: the memory location of "tt" depends on the compiler AND in your case on the type-size of "t"). -
The nice thing about pointer arithmetics is that it increases/decreases the address by the exact right amount of bytes that your underlying data type needs.
This comes in really handy when you're using pointers to structs that may even have or not have padding depending on the architecture (32/64 bit).
In your example, it wouldn't even make sense to increase p by 1 byte because the following dereference as integer then would be from an odd address, and a lot of CPUs just crash with unaligned access.
Oh, and pp should have type int anyway, not int *. ;-) -
Thanks, I noticed the wrong initialisation of pp some time after it posted the rant, but thanks for pointing that out (no pun intended). Still pretty new to c++, I'm slowly getting beyond that "wtf is this for a garbage language" to "hey that's pretty cool".
I am coming from memory safe languages like php, golang (although golang has pointers, but doesn't allow you to move them), so that's a pretty new concept to me. -
Well, pointer represent a memory address. As such they are integers (you can cast a pointer to an integer).
This becomes useful if you program operating systems: For graphical output you have to copy your text to a specific memory address, further text to address + x and so on.
This also allows some funny thing (iterate over array until 0, it is obviously only save for 0 terminated arrays):
while (*(c++)) ... ; -
@kolaente since you are learning C++ and not C: a big difference is that idiomatic C is pointer heavy, and while that is legal also in C++, it is no longer idiomatic. In C++, the use of plain C pointers is regarded at least as bad style.
In C, arrays become pointers when you hand them over to a function, thereby losing the length info. So you hand over pointer and length, and you need to manually ensure that they match. In C++, you would use a container class instead that has its length info inside so that no mismatch can occur.
Then in C, you would loop through that stuff with pointer arithmetics (or array indexing, which is the same). In idiomatic C++, you would use iterators instead.
But in order to understand what is going on in C++, you still need a firm grasp of C pointers even if you don't actually use them. :-)
Related Rants
-
xjose97x20Just saw a variable in C named like this: long time_ago; //in a galaxy far away I laughed no stop.
-
Unskipp24So this happened last night... Gf: my favorite bra is not fitting me anymore Me: get a new one ? Gf: but it ...
-
sam966911
Hats off to this lady .... I would have just flipped the machine
Why on earth can one perform calculations on pointers in c++? I can think of a dozen ways this could go wrong, but none where this is useful.
Following example:
int t = 1234;
int tt = 5555;
int* p = &t;
int* pp = *(p + 1):
Here pp will give me 5555...
question
c++
golang is really c++ light