Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
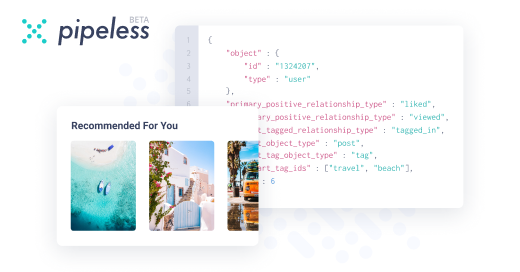
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
I take it you're never had a conversation with Brian Goetz. If you had, you'd know exactly where all the fuckery originates.
-
SomeNone7115yI mean, it's good to have consistent APIs between different data structures, but honestly, all a stack should have is peek(), pop(), and push(), while a deque should have add, remove, and peek operators for either end. Nothing else. I guess KISS was not a working motto for the designers.
-
I'd guesstimate that the reason was to allow the stack to inherit e.g. the copy and realloc methods of vector.
Obviously, that would have better been done with a common base class like "dynamic container" from which both vector and stack would inherit.
However, that didn't work because stack was probably forgotten and only introduced as afterthought. Which is exactly the shocker, to forget an important data structure like the stack in the design. -
@Fast-Nop That's what i exactly think of, i mean if you look at how Microsoft does this in C#:
System.Collections.Generic.Stack<T> implements System.Collections.Generic.IEnumerable<T>, and System.Collections.Generic.IReadOnlyCollection<T> and System.Collections.ICollection, and System.Collections.ICollection has only two methods CopyTo() and the inherited GetEnumerator() method from System.Collections.Generic.IEnumerable<T>
In Java, java.util.Stack<T> extends java.util.Vector<T> which implements java.util.List<T> which extends java.util.Collection<T>, and the last interface define all those useless methods, like the development team couldn't abstract anything
Related Rants
Oh yeah ... Java is cool in an utterly sick way even that i can't seem to find a non-retarded built-in stack data structure
Call me a racist, but java.util.Stack has a removeIf() method in case you want to remove odd numbers:
import java.util.Stack;
public class App {
public static void main(String[] args) {
int arr[] = { 2, 4, 7, 11, 13, 16, 19 };
Stack<Integer> s = new Stack<Integer>();
for (int i = 0; i < arr.length; i++) {
s.push(arr[i]);
}
s.removeIf((n) -> (n % 2 == 1));
System.out.println(s); // [2, 4, 16]
}
}
Stop using java.util.Stack they said, a legacy class they said, instead i should use java.util.ArrayDeque, but frankly i can still keep up being racist (in a reversed manner):
import java.util.ArrayDeque;
import java.util.Deque;
public class App {
public static void main(String[] args) {
int arr[] = { 2, 4, 7, 11, 13, 16, 19 };
Deque<Integer> s = new ArrayDeque<Integer>();
for (int i = 0; i < arr.length; i++) {
s.push(arr[i]);
}
s.removeIf((n) -> (n % 2 == 1));
System.out.println(s); // [16, 4, 2]
}
}
The fact that you can iterate through java.util.Stack is amazing, but the ability to insert element in a specified index:
import java.util.Stack;
public class App {
public static void main(String[] args) {
int arr[] = { 2, 4, 7, 11, 13, 16, 19 };
Stack<Integer> s = new Stack<Integer>();
for (int i = 0; i < arr.length; i++) {
s.push(arr[i]);
}
s.add(2, 218);
System.out.println(s); // [2, 4, 218, 7, 11, 13, 16, 19]
}
}
That's what happens when you inherit java.util.Vector, which is only done a BRAIN OVEN person, a very brain oven even that it will revert to retarded
If you thought about using this type of bullshit in Java get yourself prepared to beat the disk for hours when you accidentally call java.util.Stack<T>.add(int index, T element) instead of java.util.Stack<T>.push(T element), you will probably end up breaking the disk or your hand, but not solving the issue
WHY THE F*** CAN'T WE HAVE A WORKING NORMAL STACK ?
devrant
java