Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
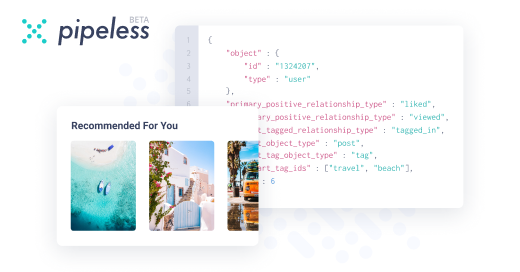
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Related Rants
My biggest influence on coding style is working with other people's code. I know the temptation to write "clever" code and I've been (and probably still occasionally am) guilty of it myself, but it's not until you have to debug someones oneliner iterator which has !(i-j) as the stop condition that you start to appreciate dumb, boring, obvious code.
If having a series of if checks in a long list makes it readable, keep it that way. If it makes it more readable to rewrite it into a nested switchcase with a couple of ternary bits, go ahead. Just don't spend half a day wrapping it up into two layers of abstraction that will require an onboarding process for the rest of the team.
rant
wk231
principle of least astonishment
cool but confusing
code golf