Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
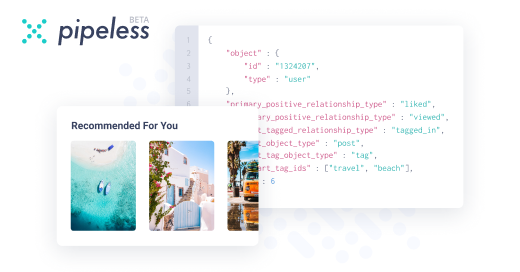
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
Look up A Star Path Search. Maybe you can even solve this with the Bus Against The Wall algorithm.
-
Root778215yThis is hardly a programming problem. It’s a math problem. Do the math first, then write the code.
Your current solution a) has too many steps (it requires exactly one step), and b) is implemented backwards.
(Also, you’ve misspelled “bus” four out of four times!) -
One liner
int empty_seats = input % 50 == 0 ? 0 : input <= 50 ?
50 - input : 50 - (input % 50); -
If you're too stupid to solve this, just quit programming as career option and try flipping burgers, maybe that's something you can do.
-
iiii95205y@GiddyNaya a bit too complicated.
It should be:
n%50==0 ? 0 : (50 - n%50)
Or if you feel hacky:
n%50 && (50 - n%50) -
@iiii "n%50 && (50 - n%50)" is either 0 as intended if n is divisble by zero, but otherwise, the result is 1 like with any boolean expression.
And "(50-n)%50" won't work as intended for n>50. -
You can get the number of passengers on the last bus by calculating the number of passengers total modulo 50 since modulo gives you the number “left over” when one number is divided by another and doesn’t divide evenly. (Eg: 5 mod 2 is 1 since 2/2 = 4, leaving 1 left over)
Then to calculate the number of empty seats on the last bus, you subtract that value from 50.
It’s easy to overthink these types of problems, especially if you don’t have a solid grasp of mathematics to begin with (which I don’t necessarily think is a requirement for programming - though it definitely makes things easier).
That being said, no idea what AOA means, so sorry if you were asking a group of people that doesn’t include me. 😅 -
Typing on a phone is annoying.
int lastBus(int passengers) {
int emptySeats = 50 - (passengers % 50);
if (emptySeats == 50) {
return 0;
}
return emptySeats;
} -
@iiii It does work as specified in the standard: "(a/b) * b + a%b shall equal a".
For e.g. (-60)%50, that's a=-60 and b=50. a/b is -1, times 50 is -50. The only thing that you can add to -50 and get -60 as result is -10, so:
(-60)%50 == -10.
That's because % isn't actually modulo, it's the remainder. -
@iiii Not particularly helpful if the ask is for help writing a program in C++, friend.
-
iiii95205y@AmyShackles I know. I just mixed up languages in my head. I thought that shorthand actually works.
-
Doesn’t modulo work the same way in C++ and JavaScript? They both return the remainder...
-
@iiii That's because the % operator isn't a modulo operator in C/C++. It's the remainder, not modulo.
-
iiii95205y@AmyShackles I meant this expression:
n%50 && (50 - n%50)
This works in JavaScript and returns the result of the second expression if the first one returns a non-zero value. -
@iiii Eh, I think the whole “actually returning a boolean from a logical comparison” rather than “returning one of the expressions from a logical comparison” makes more sense, honestly.
-
iiii95205y@AmyShackles dunno. Numbers representing booleans are pretty logical: it's either a zero and false or a non-zero and true. It does not really have to be a number. Just bytes and a basic machine command of comparing to zero, or even a zero-flag which indicates whether last arithmetic operation returned zero or not 🤷♂️
-
@iiii C/C++ do it their way because it gives the compiler more leeway for optimisation. E.g. the actual calculation doesn't have to be computed if the compiler can do something equivalent under the "as-if-rule".
-
iiii95205y
-
@iiii Just because you _can_ do things in one line doesn’t mean you _should_, friend.
AOA friends please help me to solve the program of C++
#include <iostream>
using namespace std;
int main() {
int passengers = 126;
int empty_seats = 0;
//passengers =126;
passengers after 1st bas leaving= passengers - 50;
passengers after 2nd bas leaving= passengers - 50;
passengers after 3rd bas= passengers;
empty_seats= 50 % passengers;
cout << "Empty seats in last bas"<< empty_seats <<endl;
return 0;
}
question