Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
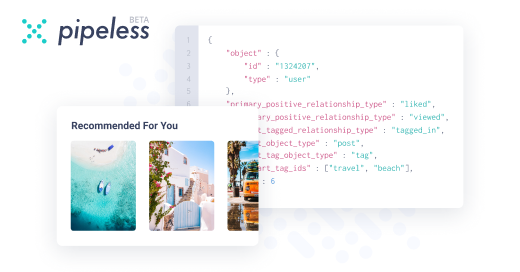
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
Well I‘m not familiar with ruby but this looks like it handles it like most high level languages do.
The kind of type determines if its instance is passed by value or by reference.
For example, structs are used as value types and classes are used as reference types. But instances of both are referred to as objects.
So it is clear what is happening. C++ is just different in that it doesn’t handle value/reference semantics at the type declaration but at the usage of the instances and declaration of the variables. -
I may have mixed passing primitives and passing objects (which happens by reference) but my point was that in language like C or C++ it is clear whether or not the current declared variable is of a concrete value or a reference to some value/memory. That's what I meant by explicit intent in that regard.
Related Rants
-
xjose97x20Just saw a variable in C named like this: long time_ago; //in a galaxy far away I laughed no stop.
-
Unskipp24So this happened last night... Gf: my favorite bra is not fitting me anymore Me: get a new one ? Gf: but it ...
-
sam966911
Hats off to this lady .... I would have just flipped the machine
So in Ruby, everything is passed to functions by value. However, when you manipulate objects, you're actually manipulating references. A simple example:
```
a = [3]
b = a
c = a
b.push(2)
print a
print b
print c
# => [3, 2][3, 2][3, 2]
```
Here's a more complicated example from the problem I was solving:
```
table = Array.new(5) { Array.new() }
1.upto(5 - 1) do |i|
1.upto(5 - 1) do |j|
table[i] = table[j]
end
table[i] << rand(1..6)
end
```
I have been running around in circles this morning because I forgot that. This makes C++ for example, more clear than Ruby since C++ explicitly shows the intent to the programmer.
rant
ruby
c++
references
memory
heap