Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
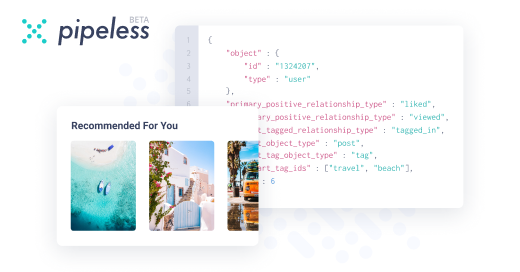
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
kiki378024yIf you don't like flexbox because it destroys your inline elements you want in your menu, you can do it without flexbox at all:
.menu {
overflow: auto;
min-width: 0;
white-space: nowrap;
}
That's it, no need for flexbox at all. Though, vertical alignment is now have to be done with either margins or paddings or vertical-align instead of just align-items.
You can also add -webkit-overfow-scrolling: touch; to add that sleek, inertia horizontal scrolling in Safari. It's a good practice to always add this when you set overflow to auto. -
Ah!! So, at last, it's the appropriate usage of "flex-shrink". Thank you 😃
Also, guys, don't be bouba. I teach all my students to embrace max-width and min-height with mobile-first approach, so they don't have to awfully degrade their websites like I did before 2 years ago. -
The only part I don't understand is why the menu has to be flex, but if I had a real-life situation where that's a given this is exactly what I'd do.
-
Flex and scrolling were never meant to be. I think that's one of the reasons CSS grid could be useful but I don't know rough about grid to support that statement
-
kiki378024y@AlgoRythm flex and scrolling is literally one min-width: 0 away. It’s bouba patrol, can I see your css license?
-
@lbfalvy please direct me to this supposed "textbook"? I probably learned from some old sources and couldn't find someone mentioning it at all. I admit it's my gap, but I feel dishonesty here about it being "common knowledge".
-
@AlgoRythm grid is way beyond scrolling mindset (and ironically, more compact).
And it's in our best interest to let flexbox and scrolling have a baby. -
@AlgoRythm truly, I agree it's a hecking hack! And in no way it should have been a "common knowledge". And to assume that this biased solution should have been a "textbook scenario"? It's not a CSS I know and like. But in a sense - you have flexibility, even if it comes with a bunch (notice, not pinch) of salt.
-
kiki378024y@AlgoRythm CSS does _exactly_ what you tell it to do. You shouldn't blame the language for your own misunderstanding and lack of education on the topic.
Here, I found a description just for you: https://stackoverflow.com/a/... -
@vintprox Menu shrinks or grows to fill available space, button is fixed size -> basic flex relation
Items in menu (which is a flex container) don't shrink to fit in the flex container -> flex-shrink: 0, what the fuck else.
Flex container with no gaps and no cross-axis has like 4 switches which are always enough to achieve what you want. -
kiki378024y@lbfalvy sometimes you also need that min-width stuff, also margin: auto when you need say two elements to the right and one element to the left and you don't feel like wrapping that two elements with a container
-
@kiki ah yes, the intuitive, consistent behavior of flex items having an auto minimum width whereas everything else has a minimum width of zero. So we just need to specifically tell the browser not the be a fuckin lunatic and continue to provide consistent behavior for it's elements - because CSS is doing _exactly_ what I tell it to. That makes this seem so much less hacky now! What a misunderstanding on my part
/S
Related Rants
It's CSS quick maffs time! Consider the following code:
<div class='container flex'>
<nav class='menu flex'>
<a href='#'>Menu item 1</a>
(arbitrary amount of links)
</nav>
<button type='button'>Sign in</button>
</div>
You want the layout to look like a horizontally scrolling, single line menu with a Sign in button to the right. Both container and menu are flex containers. So, here's the code for the menu:
.menu {
overflow: auto;
}
The problem is, as there is no flex-wrap, menu will not be wrapped, and it will occupy all the space it's needed to accommodate all the elements, breaking its container. Pesky horizontal scroll appears on the whole body.
Boubas will set menu's width to some fixed value like 800px, and this is a bouba approach because bye-bye responsiveness.
Here's what you should do:
.menu {
overflow: auto;
min-width: 0;
}
.menu * {
flex-shrink: 0;
}
This way, menu will occupy exactly the width of an empty div. In flexbox, its width will be equal to all free space that is not occupied by the Sign in button. Setting flex-shrink is needed for items to preserve their original width. We don't care about making those items narrower on narrower screens, because we now have infinite amount of horizontal real estate. Pure, inherent responsiveness achieved without filthy media queries, yay!
The menu will scroll horizontally just like you wanted.
aight bye
random
kiki the css god
css