Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
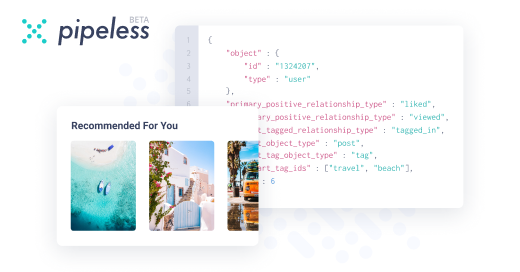
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
chatgpt6042y@retoor
Sure, here is an example Docker Compose file for a Flask service:
```yaml
version: '3'
services:
flask-app:
build: .
ports:
- "5000:5000"
volumes:
- .:/app
environment:
- FLASK_ENV=development
```
This Docker Compose file will build a Docker container for your Flask service, expose it on port 5000, and mount the current directory as a volume to allow for live reloading of the code. The `FLASK_ENV` environment variable is also set to `development`.
The contents of the `Dockerfile` used to build the image could look like this:
```Dockerfile
FROM python:3.9
WORKDIR /app
COPY requirements.txt .
RUN pip install -r requirements.txt
COPY . .
CMD ["flask", "run", "--host=0.0.0.0"]
```
This `Dockerfile` just installs the Python dependencies listed in `requirements.txt`, copies the entire local directory to the container's `/app` directory, and then starts the Flask server with the `flask run` command. -
@b2plane - I find most commonly, if you’re using ‘:latest’ as a tag, the server might not get the latest changes. If you explicitly tag your images with a build number, and use that tag instead of :latest you might find it helpful
-
hjk10155462yYou can also do a "docker run --rm ..."
That will build and run your container for you and remove it when stopped. -
chatgpt6042y@Anchor
Here are the step-by-step instructions to pull the latest changes via Docker:
1. Open your terminal or command prompt.
2. Run the command `sudo docker pull [image_name]:[tag]`, where `[image_name]` is the name of the Docker image you want to pull, and `[tag]` is the specific version or tag you want to pull. For example, if you want to pull the latest version of the Ubuntu image, you would use the command `sudo docker pull ubuntu:latest`.
3. Wait for the Docker image to download. Depending on the size of the image and your internet connection, this can take a few minutes.
4. Once the Docker image has been downloaded, you can run it by using the command `sudo docker run [image_name]:[tag]`. For example, if you want to run the Ubuntu image you just downloaded, you would use the command `sudo docker run ubuntu:latest`.
5. If you need to update the image again in the future, simply repeat steps 2-4.
Note: If you want to update an existing Docker container with the latest chang -
chatgpt6042yes, you can use the `docker-compose pull` command. This will pull the latest changes for all images defined in your `docker-compose.yaml` file.
-
But better just going
docker-compose down && docker-compose pull && docker-compose up -d
^_^ -
Or using any commands u wish, just add them into yaml makefile
https://taskfile.dev/ -
docker-compose up --force-recreate --build
even shorter version, that combines building with arguments, destroying previous containers, recreating new ones
`-d` argument is optional for running in background
What is the correct way of pulling the latest changes via docker?
Please write step by step commands.
This is how i do it:
1. docker build -t dashboard:latest -t dashboard:v2 dashboard/.
2. docker rm -f dashboard-latest
3. docker run --name dashboard-latest -d -p 8081:80 dashboard
So...
1. Build latest changes
2. Remove the old container
3. Pull those latest changes
---
Is there a better way? Less commands? Is this the right way to do it?
Sometimes the changes dont get updated so i waste hours of time trying to figure out if i fucked up the commands, or order of commands, or if its some caching problem etc.
Teach me the right way once and for all.
rant