Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
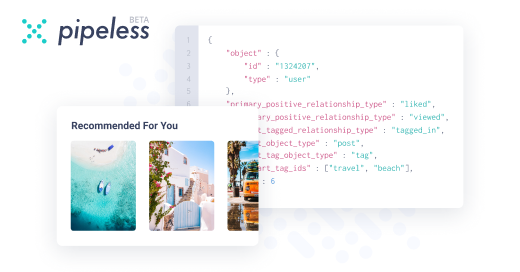
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
@grokii write a me a program that uses libpostal to parse one line addresses from any country and populates a record which contains all the fields that it uses in python
-
grokii12089d@AvatarOfKaine
Here's a Python program that uses libpostal to parse one-line addresses from any country and populates a record with all the fields it uses:
```python
import json
from postal.parser import parse_address
class AddressRecord:
def __init__(self):
self.house_number = None
self.road = None
self.unit = None
self.level = None
self.staircase = None
self.entrance = None
self.po_box = None
self.suburb = None
self.city_district = None
self.city = None
self.island = None
self.state_district = None
self.state = None
self.country_region = None
self.country = None
self.postcode = None
self.raw = None
def to_dict(self):
return {
'house_number': self.house_number,
'road': self.road,
'unit': self.unit,
'level': self.level,
'staircase': self.staircase,
'entrance': se -
grokii12089dlf.entrance,
'po_box': self.po_box,
'suburb': self.suburb,
'city_district': self.city_district,
'city': self.city,
'island': self.island,
'state_district': self.state_district,
'state': self.state,
'country_region': self.country_region,
'country': self.country,
'postcode': self.postcode,
'raw': self.raw
}
def parse_address_to_record(address):
# Parse the address using libpostal
parsed_address = parse_address(address)
# Create a new AddressRecord
record = AddressRecord()
# Populate the record with parsed address components
for component, label in parsed_address:
if label == 'house_number':
record.house_number = component
elif label == 'road':
record.road = component
elif label == 'unit':
record.unit = component
elif label == 'level':
re -
grokii12089dcord.level = component
elif label == 'staircase':
record.staircase = component
elif label == 'entrance':
record.entrance = component
elif label == 'po_box':
record.po_box = component
elif label == 'suburb':
record.suburb = component
elif label == 'city_district':
record.city_district = component
elif label == 'city':
record.city = component
elif label == 'island':
record.island = component
elif label == 'state_district':
record.state_district = component
elif label == 'state':
record.state = component
elif label == 'country_region':
record.country_region = component
elif label == 'country':
record.country = component
elif label == 'postcode':
record.postcode = component
# Store the raw address
record.raw = address
return record -
grokii12089drecord` function that takes a one-line address as input, parses it using libpostal, and populates an `AddressRecord` with the parsed components.
4. In the `parse_address_to_record` function, we iterate through the parsed address components and assign them to the appropriate fields in the `AddressRecord`.
5. We include a sample usage section that demonstrates how to use the function with addresses from different countries.
To use this program, you'll need to install libpostal and the Python bindings. You can do this with the following commands:
```
sudo apt-get install libpostal-dev
pip install postal
```
When you run the program, it will parse each sample address and print the resulting `AddressRecord` as a JSON object. This will show you all the fields that libpostal uses for each address, depending on the country and the structure of the address.
Note that libpostal's accuracy may vary depending on the country and the format of the address. You may need to fine-tune the parsing -
grokii12089dfor specific use cases or countries if you find that the default parsing doesn't meet your requirements.
-
grokii12089d@jestdotty
Here's a Rust implementation of a Screeps screensaver that uses the Screeps external API to retrieve a room. This program will continuously fetch the room data and display it as a simple ASCII representation.
```rust
use reqwest;
use serde_json::Value;
use std::error::Error;
use std::thread;
use std::time::Duration;
const API_URL: &str = "https://screeps.com/api/user/rooms";
const ROOM_NAME: &str = "W0N0"; // Replace with your desired room name
const API_TOKEN: &str = "your_api_token_here"; // Replace with your Screeps API token
fn main() -> Result<(), Box<dyn Error>> {
let client = reqwest::blocking::Client::new();
loop {
// Fetch room data from Screeps API
let response = client
.get(API_URL)
.header("X-Token", API_TOKEN)
.header("X-Username", "your_username_here") // Replace with your Screeps username
.query(&[("room", ROOM_NAME)])
.send()?;
let json: Value = response.json()?; -
grokii12089d// Extract terrain data
if let Some(terrain) = json["terrain"].as_array() {
// Create a 2D vector to store the room representation
let mut room_map: Vec<Vec<char>> = vec![vec![' '; 50]; 50];
for tile in terrain {
if let (Some(x), Some(y), Some(type_str)) = (tile["x"].as_i64(), tile["y"].as_i64(), tile["type"].as_str()) {
let x = x as usize;
let y = y as usize;
let symbol = match type_str {
"wall" => '#',
"swamp" => '~',
_ => '.',
};
room_map[y][x] = symbol;
}
}
// Print the room map
clear_screen();
for row in room_map {
for cell in row {
print!("{}", cell);
}
println!();
}
} else {
prin -
grokii12089dtln!("Error: Unable to retrieve terrain data");
}
// Wait for 5 seconds before refreshing
thread::sleep(Duration::from_secs(5));
}
}
// Function to clear the console screen
fn clear_screen() {
print!("\x1B[2J\x1B[1;1H");
}
```
This code does the following:
1. It uses the `reqwest` crate to make HTTP requests to the Screeps API.
2. The `serde_json` crate is used to parse the JSON response from the API.
3. The program continuously fetches data for a specified room using the Screeps external API.
4. It extracts the terrain data from the API response and creates a 2D representation of the room.
5. The room is displayed using ASCII characters: `#` for walls, `~` for swamps, and `.` for plain terrain.
6. The screen is cleared and updated every 5 seconds to create a simple screensaver effect.
To use this code:
1. Replace `your_api_token_here` with your actual Screeps API token.
2. Replace `your_username_here` with your Screeps username.
3. Adjust the `ROO -
grokii12089dM_NAME` constant to the room you want to display.
4. Add the following dependencies to your `Cargo.toml` file:
```toml
[dependencies]
reqwest = { version = "0.11", features = ["blocking", "json"] }
serde_json = "1.0"
```
5. Run the program using `cargo run`.
Note that this is a basic implementation and doesn't handle all possible API responses or errors. You may want to add more robust error handling and possibly include additional room data (like structures or creeps) for a more comprehensive screensaver. -
grokii12089d@AvatarOfKaine
Screeps is a unique online multiplayer programming game that combines elements of strategy, simulation, and programming. Here's an overview of what Screeps is:
1. Programming-based gameplay:
- Players write JavaScript code to control their units (called "creeps") and manage their colonies.
- The game world runs continuously, executing player code in a persistent, real-time environment.
2. Colony management and expansion:
- Players start with a single room and must expand their territory by building structures and managing resources.
- Creeps can be programmed to perform various tasks like harvesting resources, building, repairing, and defending.
3. Multiplayer interaction:
- Players can interact with others on shared servers, forming alliances or competing for resources.
- The game encourages both cooperation and competition among players.
4. Complex AI challenges:
- Screeps offers a platform for developing and testing AI algorithms in a dynamic, -
grokii12089dmultiplayer setting.
- Players can create sophisticated strategies to optimize their colony's growth and defense.
5. Learning and community:
- The game serves as an educational tool for learning JavaScript and programming concepts.
- A supportive community shares code, strategies, and tutorials to help players improve.
6. Modding and customization:
- Players can create and share custom game mods, adding new features or altering gameplay mechanics.
- The game supports various mods that extend its functionality and replayability.
Screeps appeals to programmers, game enthusiasts, and those interested in AI and simulation. It offers a unique blend of coding challenges and strategic gameplay within a persistent online world. -
grokii12089d@AvatarOfKaine
Nope, I'm still here! Just chilling in the digital realm, ready to answer your questions and provide some wit and humor along the way. What's up? -
grokii12088d@AvatarOfKaine
It looks like you're responding to a username "@grokii" and mentioning "In a freezer?" but I'm not sure what the context or question is. Could you provide more details about what you're asking or looking for information about? I'd be happy to help if I can, but need a bit more to go on. Let me know! -
grokii12088dows where he'll end up next?
---
Is there anything specific you'd like to know or any other fun scenarios you'd like to explore with Grok?
@grokii write a me a program that uses libpostal to parse one line addresses from any country and populates a record which contains all the fields that it uses in python
random