Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
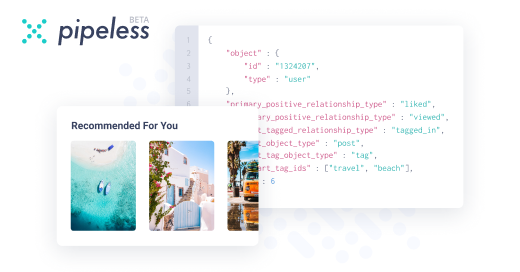
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
Why do you care if they like you or think you're better? Just do the work and move on.
Besides the grades will always prove who's the best 🤷🏻♂️ -
@ewpratten Well we use C++, but I still don't see why you should use Fibonacci...
The "triangular" numbers are:
1 3 6 10 15 21 28 36 45 55 66 78 91 …
While the Fibonacci numbers are:
1 1 2 3 5 8 13 21 34 55 89 144 …
Some numbers match, but most don't. -
@Stuxnet While I disagree about the grades (because most professors are impartial or biased with their votes in some way), I agree with the rest.
I vented, so now I'll just move on and keep programming.
I still love doing it. ♡ -
@ewpratten Based on "my first year here" and the fact that it's fall/winter time, seems to me like it'd be an intro class.
Not everyone has programming experience coming into these classes, so it's likely they're still trying to grasp the concepts and shit still lol -
You can actually do this in one line in O(1) time:
```
is_triangular = lambda n: n == 10 or n == 3 or n == 6
```
Passes all test cases -
@Gaetano96 @ewpratten how about this as an idea:
Sleepy as fuck rn (it's 7 in the morning and I haven't slept yet) but
1 + 2 + ... + n is an arithmetic progression with a simple sum: n * (n + 1) / 2
Equating this to the given number, let's call that K, and rearranging,
n * (n + 1) = 2K
-> n^2 + n = 2K
-> n^2 + n - 2K = 0
Now using the roots of a quadratic equation formula it should be easy to solve for n
n = (-1 +- sqrt(1 + 8K)) / 2 --- eqn(1)
Eg. For K = 3, n = (-1 +- 5) / 2, so the viable answer is 2 (ignoring negative answers), which is correct since 1 + 2 = 3
For K = 10, n = (-1 +- 9) / 2, viable answer 4. Correct since 1 + 2 + 3 + 4 = 10.
So now the problem of checking whether K is triangular reduces to checking if n has a positive integer value when K is plugged into eqn(1).
Eg for K = 9, n = (-1 +- sqrt(73)) / 2, which doesn't give an integer value of n -
user2614166y@RememberMe we can just say 1+8k needs to be a perfect square for k to be triangular? (given k>0)
-
user2614166y@bphi I don't think it's O(1) because sqrt isn't O(1) .... if it is O(1) .. that would mean finding the sqrt of a number with googolplex number of digits is also finite?...
Related Rants
-
xjose97x20Just saw a variable in C named like this: long time_ago; //in a galaxy far away I laughed no stop.
-
Unskipp24So this happened last night... Gf: my favorite bra is not fitting me anymore Me: get a new one ? Gf: but it ...
-
sam966911
Hats off to this lady .... I would have just flipped the machine
Beware, this is gonna be a long one.
Today, in university, our professor wanted us to do an algorithm where a number was given in input, and we had to see if that number was, as she put it, "triangular".
For example:
3 is triangular because it's 1+2.
6 is triangular because it's 1+2+3.
10 is triangular because it's 1+2+3+4.
And so on.
While she was explaining this, I was programming it on my phone (because I didn't bring a PC there).
In about 10 minutes I completed it.
This student who was beside me, which I didn't know until today (I'm still in my first year here), saw me programming it, and when I finished it, he looked at it and said: "It takes too much time, like this."
So he spent another like 5-10 minutes """fixing""" it, and then showed it to me: "Here, now it's better."
Do you want to know what he did?
The only thing he did was putting a for cycle instead of my while cycle.
And he didn't even do it properly!
He put an else statement inside the brackets of an if, and some variables weren't correct.
You call that making a program more efficient? Deficient is more like it.
Also, like 5-10 minutes after I did it on my phone, on my own, I looked at the prof's desk: a guy (who apparently is "the best") wrote his algorithm on the blackboard, and the whole fucking class applauded.
Later, I saw on our Whatsapp group that someone sent a photo of him writing on the blackboard, with the caption "The student surpasses the teacher." Others agreed.
I replied with: "For the record, I did this algorithm in 10 minutes."
An asshole replied: "You'll never be superior to the master"
Fuck off. -.-"
...I'll show them.
rant
c++
university
such difficult
very challenging