Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
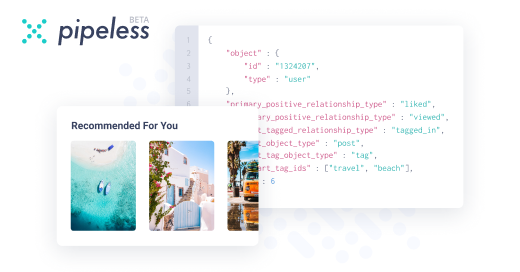
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Related Rants
My journey along Java continues and so I have discovered something I didn't know before:
If a subclass tries to call a method on its parent which it has not overridden, then it will call the method as if you hadn't used the keyword 'super' (and I think it will try to find it in the classpath and SDK).
Example 1:
public class SuperParent {
public String test(){
return "SuperParent";
}
}
public class Parent extends SuperParent {
}
public class Child extends Parent {
public String testChild(){
return super.test(); // same effect as test();
}
}
public class TestInheritance {
public static void main(String[] args) {
System.out.println(new Child().test()); // returns "SuperParent"
}
}
Example 2: with getClass():
public class Parent {
@Override
public String toString(){
return super.getClass().getSimpleName();
}
}
public class Child extends Parent {
}
public class TestInheritance {
public static void main(String[] args) {
System.out.println(new Child()); // prints "Child"
}
}
This here is of course a special case: .getClass() will always return the class name of its caller, so naturally in this case it returns Child and not Parent.
You would expect it to return "Parent" since you use 'super' in the overridden toString() but it returns the Class name of the Child (then there's something in programming languages such lexical scope and execution scope, which I'm not sure if it applies here).
The solution for this example is of course .getSuperClass().
Inheritance isn't always straight-forward.
References:
https://stackoverflow.com/questions...
https://stackoverflow.com/questions...
rant
inheritance
java