Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
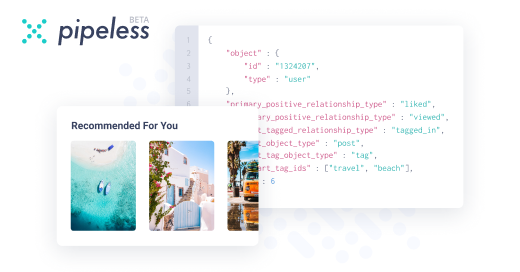
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Related Rants
I recently started working on laravel. As the community says it was easy to get along with the framework and its methodologies. But then i had to do multiple login with framework in same domain.
Oh man, i spent a week to make it work. All those guards and middlewares realted to login was driving me crazy. The concept was clear, but somehow the framework was like "You! I shall make you spend a week for my satisfaction". The project demo was nearing and i was doing all kind of stuff i found. Atlast after continous tries it worked. Never in my 4+ years as a developer i had to face such an issue with login.
So here is how it works,if anyone faces the same issue:
(This case is beneficial if you're using table structures different from default laravel auth table structures)
1. Define the guards for each in auth.php
Eg:
'users' => [
'driver' => 'session',
'provider' => 'users',
],
'client' => [
'driver' => 'session',
'provider' => 'client',
],
'admin' => [
'driver' => 'session',
'provider' => 'admins',
],
2. Define providers for each guards in auth.php
'users' => [
'driver' => 'eloquent',
'model' => <Model Namespace>::class,
'table' => '<table name>', //Optional. You can define it in the model also
],
'admins' => [
'driver' => 'eloquent',
'model' => <Model Namespace>::class,
],
'client' => [
'driver' => 'eloquent',
'model' => <Model Namespace>::class,
],
Similarly you can define passwords for resetting passwords in auth.php
3. Edit login controller in app/Http/Controller/Auth folder accordingly
a. Usually this particular line of code is used for authentication
Auth::guard('<guard name>')->attempt(['email' => $request->email, 'password' => $request->password]);
b. If above mentioned method doesn't work, You can directly login using login method
EG:
$user = <model namespace>::where([
'username' => $request->username,
'password' => md5($request->password),
])->first();
Auth::guard('<guard name>')->login($user);
4. If you're using custom build table to store user details, then you should adjust the model for that particular table accordingly. NOTE: The model extends Authenticatable
EG
class <model name> extends Authenticatable
{
use Notifiable;
protected $table = "<table name>";
protected $guard = '<guard name>';
protected $fillable = [
'name' , 'username' , 'email' , 'password'
];
protected $hidden = [
'password' ,
];
//Below changes are optional, according to your need
public $timestamps = false;
const CREATED_AT = 'created_time';
const UPDATED_AT = 'updated_time';
//To get your custom id field, in this case username
public function getId()
{
return $this->username;
}
}
5. Create login views according to the user types you required
6. Update the RedirectIfAuthenticated middleware for auth redirections after login
7. Make sure to not use the default laravel Auth routes. This may cause some inconsistancy in workflow
The laravel version which i worked on and the solution is for is Laravel 6.x
devrant
php
laravel