Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
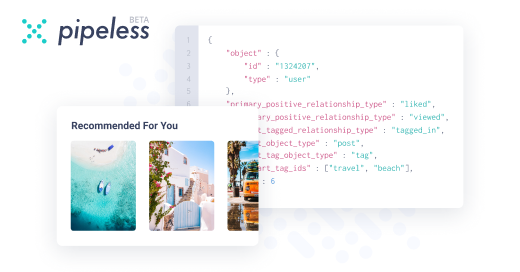
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
Use Slf4j. It's an abstraction on top of all the various java logging frameworks to provide consistency. You'll have Slf4j and a configured underlying log provider that it abstracts.
https://www.stackify.com/slf4j-java
https://baeldung.com/slf4j-with-log... -
donuts233865y@SortOfTested so how does it work
Slf4J-log4j, logback, whatever?
Will slf ever pull this shit...
Still gotta figure out how to mass change a ton of files... -
See links in edit.
Slf doesn't do any logging itself. It just exists to provide a common log interface over different applications, and to consistently integrate logging hooks from dependency libraries. -
donuts233865y@SortOfTested I see in baeldung, there's an log4j over slf4j.
Just tried it and seems like it fixed the renaming issue...
Removed log4j1 and replaced with slfj and log4j2... Compiles without changing the packages
Just need to test in uat.... And if it does it makes all that time spent renaming stuff this morning wasted (trying slf on a new branch from main). -
donuts233865y@SortOfTested I think he meant what I typed in the original as a joke.
I'm actually surprised you actually understood all of it cuz I was reading it again and going "wtf... The keyboard fcked up again" -
@donuts
You are far from the first person to suffer the pain of java logging sprawl 😋 all I needed to see was an enumeration of java logging tech to know what the question was. -
donuts233865y@SortOfTested and I thought the sprawl only happened in JS... Maybe it's just spreading with all the open source...
-
@donuts
I've long said that is the one true weakness of open source:
Any minor disagreement results in a new forked project. -
@donuts In a nutshell, this is what seperation into layers is all about.
Precise generic API which hides the actual API.
Aka "Fuck that everything changes" -
Okay, your rant made me double check, and yes, I was correct.
```
import org.apache.log4j.LogManager;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
```
If you're using slf4j already, why commit to using log4j? I mean it kind of beats the purpose of slf4j.
SLF4J is an abstraction. You can use slf4j's Logger in your code to make log entries. The best part - you can have *any* logging engine underneath, that implements slf4j's interfaces. This way you can swap/upgrade/replace/do wtv you want with your log4j w/o making a single change in your code. You can simply replace log4j dependency with logback and still it would work (with logback's properties ofc).
I got used to
private final Logger logger = LoggerFactory.getLogger(getClass());
since this approach only has to know about slf4j - the abstraction. And unless you're doing smth log4j-specific, I suggest you use this approach as well :)
Implementations often change [even with upgrades]. Abstractions change VERY rarely. -
And all this log4j/slf/logback/etc magic is quite simple actually.
package org.slf4j;
public interface Logger {
void info(String msg);
void debug(String msg);
void warn(String msg);
}
––––––
package org.apache.log4j;
public class Logger implements org.slf4j.Logger {
// implementations
}
––––––
package ch.qos.logback;
public class Logger implements org.slf4j.Logger {
// implementations
}
––––––
package my.homemade.logger;
public class Logger implements org.slf4j.Logger {
// implementations
}
–––––
and so on.
And the LoggerFactory basicaly does
package org.slf4j;
public class LoggerFactory {
public static Logger getLogger(Class cl) {
List<org.slf4j.Logger> available = findClassesInClasspathImplementing(org.slf4j.Logger.class);
if (available.size() == 1) return available.get(0);
else if (available.size() > 1) printTooManyLoggersError();
return NullLogger.instance();
}
}
That's pretty much a pseudocode ofc. But it should explain -
donuts233865y@netikras I'm using log4j1, need to upgrade to 2 at least. Then remember slf4j from somewhere at well as Logback... So basically was going "ok.... Which way should I go?"
And the answer was SLF4J, Log4J, + some plugin that fakes log4j.Logger so I don't need to rewrite any existing code. -
The whole issue stems from the fact that Java didn't have any sane built-in logging for about a decade, so everyone used log4j. Instead of bringing log4j into core, they then decided to release their own logging implementation, making things worse, not better.
Things then fragmented again when the log4j team split, forming logback and log4j2, then commons logging came to try to fix the situation by using classpath hacks - that hugely backfired, and now slf4j is here which is... a cludge, sure, but the best we can reasonably expect.
From memory it also contains code for converting to it from legacy log4j implementations, so you should be good there too.
Can someone explain to me Java lighting...
There log4j, slf4j, logback. Some are interfaces, others are implementations.
What is the setup so I can basically wit one and forget, or upgrade with the jar amount of code changes?
I need to upgrade log4j 1 to 2 and they changed the package name and how to init it....
Now it's logging.log4j.
And correct me if I'm working, Logger logger = LogMagager.getLogger(Clazz.class)
Does the log4j.properties need changing as well?
question