Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
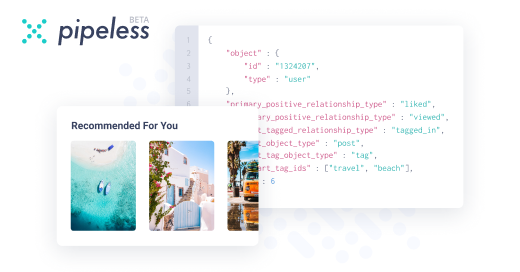
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Related Rants
Today I learned:
In Java, you're supposed to compile a source file in its package one directory up, outside of its package. You can't compile the source file in its own package directory, for it will state "cannot find symbol" on files in the same package, even though they're in the correct package directory. That can be quite confusing at first.
Given the following directory structure:
|_
|_ \pkg
|_ _ Src1.java
|_ _ Src2.java (interface with static method)
and the following source:
package pkg;
public interface Src2 { static void doStuff() { ... }; } // assuming JDK8+, where static default methods are allowed
package pkg;
public class Src1 { public static void main(String[] args) { Src2.doStuff(); } }
..being inside the pkg/ directory in the console,
this won't work:
javac -cp . Src1.java
"cannot find symbol: Src2"
However, go one directory up and..
javac -cp pkg/*.java pkg/Src1.java
..it works!
Yeah, you truly start learning how the compiler works when you don't use the luxury of a IDE but rather a raw text editor and a console.
rant
packages
wtf
today-i-learned
java