Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
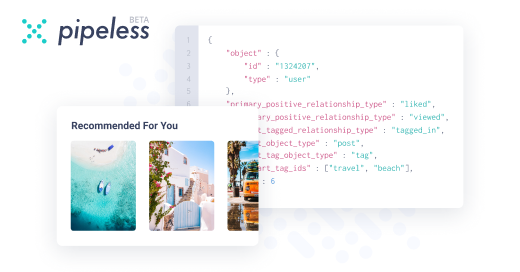
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
@AndSoWeCode depends on the exact language, but I've seen implementations that use a bitwise XOR to do it:
x = x XOR y
y = y XOR x
x = x XOR y
That said... Unless you work in systems programming, I feel like this kind of trivia is more or less useless. I've been a professional software developer for about 3 years now and have never seen a situation where swapping things was even needed, let alone swapping things within such tight constraints. -
Less lines of code don't mean less processing. Actually list($a, $b) = array($b, $a) implies an array creation and possibly iteration, which is even less efficient in terms of cpu and memory than an extra variable...
-
@Zaphod65 sorting algorithms do. Anyways, brilliant solution for swapping, might try some day.
-
In Python(and I think Rust) you can just write
(a, b) = (b, a)
I'm not sure if it's really more efficient than a temp variable, but it sure as hell looks clearer. -
@Zaphod65 FFS I TRIED AND IT WORKS!!
@Gradle now you can tell you teacher how to do it in any language. Using XOR bitwise operators.
@localjoost this is the code in PHP by the way:
$x = $x ^ $y;
$y = $y ^ $x;
$x = $x ^ $y; -
@ithinkihaversi has the answer. And thank you everyone! You surprised me with these 29 notifications. 😅😁
-
The point is:
THE way to interchange 2 values is by using a third. Everything else is worse:
It either creates a lot more variables in the background
OR
It only works for binary data with the same format that support the XOR operation
OR
is even worse than all of these.
Just because it's stupidly simple doesn't mean that it has to be changed. -
I had this moment of "Are you even a good programmer?" to most other programmers when I first figured out how to swap two variables without using a temporary variable on my own (as a part of a challenge I read somewhere). The truth is, most great programers have never really given it a thought coz it's a useless thing to know really. @gradle, I hope you're not in this moment yourself. xD
-
@AndSoWeCode I'm not saying that this is the proper way to do it, some things are just brain teasers like this one!
-
If one likes brain teasers, one shouldn't waste time with such trivial teases, and instead learn Hodor
http://www.hodor-lang.org/ -
rangler1107y@AndSoWeCode what isn't binary? Everything can be swapped with the xor method
Unless you don't have access to pointers, in which case efficiency is probably not a concern for you -
@rangler the vast majority of languages only support xor for integers. The reason is because it doesn't make sense to xor floating points since every bit means something else depending on another bit, and xor-ing strings is useless since they're just pointers and are of variable lengths. XOR on more complex data structures is even more ridiculous.
That's why performing binary arithmetic or general arighmetic for swapping variables, in general, is simply wrong. -
rangler1107y@AndSoWeCode well, thats kind of what i said you could do with an xor, it doesn't matter if the bits "make sense" like with integers. I do admit that it only applies to langs that have low level access, but if you need to save the 8bytes an auxiliary variable takes you are probably working low level.
Strings aren't xorable nor assignable to a temp variable because they're pointers, just like more complex structures. You can store said pointer in a temp var but this could also be achieved with an xor.
Cloning is another story.
Anyway, fun discussion 😁 -
@rangler in modern languages strings are treated like immutable scalars, rather than pointers to arrays in memory. An assignment is always a copy.
-
rangler1107y@AndSoWeCode a string assignment is a copy of the pointer, a string pool is maintained to save memory
Related Rants
-
shelladdicted11CS teacher tip of the day: server side input checks and sanitization are always useless. because nowdays all ...
-
Python18After working as a developer for 4-5 years I finally took up school again. The teacher at our first programmi...
-
MauriceDynasty4Teacher: hey, your good with computer programming, right? (Thinking I finally have a chance to prove myself pr...
Software development lessons are so boring and the teacher is so stupid. He can't swap two variables without a temporary var. He said that he never saw this kind of swaping before. I pay attention sometimes, but I'm just drawing in my exercise book.
rant
boring lesson
stupid teacher
swapping without temp
lessons