Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
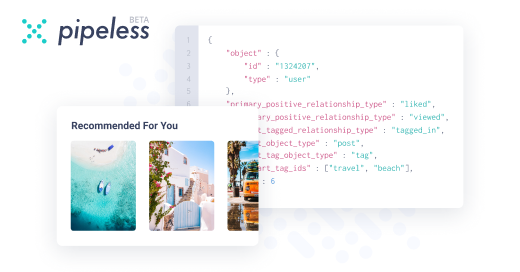
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
I hate functions returning strings when they don't have to, because the function signature is less clear, and the function should know what the function using it expects
-
Traser10317yWell.. The thing is, you shouldn't really. Comparing strings is way more intensive then let's say a boolean. In order to compare the string your cpu has to compare every character apart from each other.
Comparing boolean requires only 1 check.
If you want to go deeper into this logic you can search up "Arithmetic Logic Unit"
Here is nice video explaining the cpu, including the ALU part:
https://youtu.be/cNN_tTXABUA
And as told above, enums are the way to go in this kind of checks. -
lopu9137y@hubiruchi nah ignore my previous message my question actually covered your answer, I thought I specified inputs I'm really sorry
-
lopu9137y@nitwhiz very interesting but enums are like codes and that requires everyone running functions to know the codes
what if I just want to run the function through a url simply by api.url.domain/?stringboolean="something"
I'd have to know the code, my only argument for this is the user-friendliness, cause otherwise you have to store the enum database everywhere...?
or can you do the enum checking only function and use the inputted string to generate the enum code? So you use key hashing to navigate string match checking????????????? ohhhhhhhhh???? -
lopu9137y@hubiruchi but it seems like storing a sort of hash table for known wanted incoming strings is a hack around comparing strings, magical
-
Rikan2817yJust use enums, like nitwhiz already said. Everything else is just a fucking mess. It's way more structured, because you have one defined place in your code where you list all available options, it's faster, it's easier to refactor, you have autocompletion, other developers don't break out in tears if they see the code and it's easier for them to understand your code.
In the frontend/API side, it's no difference to using strings, either way you have to provide all possible options to select/use and the consumer doesn't care if it's a string or an enum in your backend.
Also, use switch-case expression instead of an if statement, in general it's faster and you can provide an default case, that is used if your value doesn't match any of your enum. -
@lopu @lopu enums should turn everything into an index iirc, so it would be an integer compare. C# for instance would give each successful enumeration an incremented value (for the index) so
enum Day (Sun, Mon, Tue, Wed, Thu, Fri, Sat}
Would set Sat=0, Mon=1,...Sat=6.
Probably easier than comparing strings of potentially different length -
Definitely go for an enum in your case. It makes no sense using a string as a boolean. Assuming that you take concepts like single responsibility into account, would it make sense to call your generateUsername function passing in generateUsername = false? No, you just wouldn't call the function if you don't need to generate. Also, for maintainability and reusability an enum is better; no typos, better performance, use the same enum anywhere you need it etc.
If you'd submit that code, written for my team's code base, in a pull request it would definitely be rejected. Sorry :) -
Rikan2817yDepending on your language you can Do something like this:
enum UsernameType {
HumanReadable = "humanReadable",
Gibberish = "gibberish"
}
If (queryParam == UsernameType.HumanReadable) {
// do stuff
} else if (queryParam == UsernameType.Gibberish) {
// do other stuff
} else {
// and so on
}
Or you use a switch-case
switch(queryParam) {
case UsernameType.HumanReadable:
// stuff
break;
case UsernameType.Gibberish:
// stuff
break;
default:
// stuff
}
Related Rants
Benefits of using Strings for Boolean intended logic?
I'll go first
easily implement cases before finally checking if true
generateUsername: { type: String }
if(generateUsername == 'humanReadable'){
// generate a username in a human readable format AKA yoDudeImRainbow
} else if(generateUsername == 'hash'){
// generate a username by using a random hash
} else if(generateUsername){
// generate a username by using a random hash
}
question
logic
standards
ideas
hacks
js