Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
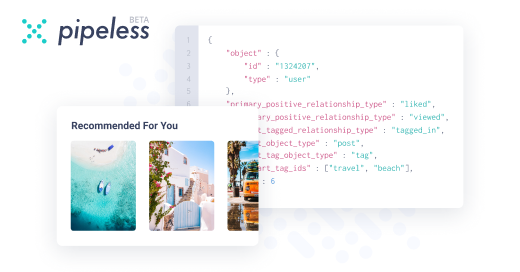
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
Hazarth97381yYeah!
C++ is not very difficult. The only thing to really look out for is memory management. Gotta learn how things are created and how they are destroyed and when. Malloc vs initializors vs new, Stack and Heap, Different kinds of pointers that help you manage it and ideally also make sure not to use unsafe functions if you gonna release your product some day. But you can also totally ignore most of these and get a "functional" software with memory leaks and undefined behavior that just about holds together by sheer luck, until it doesn't. In those situations, look at Valgrind, awesome piece of software. -
@Hazarth hey thanks Hazarth, any pointers on good memory management or pitfalls beginners tend to run into?
-
@kobenz long ass time ago when I was like eleven.
I woulda done c if so many modern libraries werent already in c++. -
Hazarth97381y@Wisecrack
Well super helpful pointer is to get used to using Valgrind, I can't overestimate how useful it is!
But in more general terms yeah:
- remember that everything you create with "new" you have to manage and clean using "delete" when done.
- You can avoid above case by using unique_ptr pointers. They will clean the object for you when it's out of scope. (You can return them from functions, they are moved implicitly to a new unique_ptr)
- remember that every bit of memory you allocate with malloc you have to manage and clean using "free" when done. Smart pointers don't work *out of the box* with malloc-ated memory.
- Everything else created on stack will be auto-managed and cleaned when the stack is popped. Which means you can't return pointers to these objects from functions if they were not created using new or malloc, they will reference unallocated memory. However you can return them directly (by-value)
- Also check out shared_ptr. One resource - multiple owners. -
Hazarth97381y@Wisecrack
In general I'd recommend avoiding "malloc" all together, but sometimes it's just more performant to work with raw memory, especially since you can control how it's placed in memory (since malloc allocates a block of memory on heap, while some other ways could allocate fragmented memory... depends on your usecase)
However allocating an array also allocates a nice block if memory and it gets auto managed on stack, which is likely what you want most of the time, but that also limits you cause it could cause a stackoverflow if you allocate something large on stack... unless you have an array of pointers, which would be the case with classes created using new, in which case you'd have fragmented memory. In that case the most readable way is using vector<T> which allocated continuous memory for your objects. vector also allocates on heap rather than stack, which will help you avoid stack overflow errors and it will manage the memory for you (unless it's a vector of pointers) -
Modern c++ is all about learning the difference between shared, unique, and weak pointers. Lvals rvals prvals. Copy and move constructors. Etc etc
-
@Hazarth I'd like you thank you and algorythm, but especially you hazarth for going the distance.
Related Rants
Bit the bullet and installed VS and relevant compilers for C++ and started fucking around with sdl.
Not as terrible as I thought it was going to be.
Pointers seem pretty intuitive. Apparently my time with python has not in fact mentally mutilated me.
random
visual studio
sdl
c++
cpp