Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
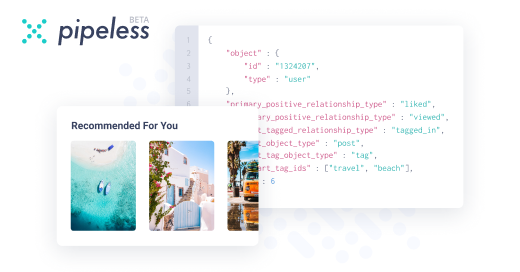
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
j0n4s52897yIt prints 10 times "0"?
Because if you use n++ it returns the n value and not the n+1 value... -
@sslPoodle the compiler will do it in two parts.
n = n++; -> n = n; n++; -> n = 0; 0++;
Ergo, N will increment anyway. -
-vim-31167yif you have ++ after, it will increment after returning it’s value, so it will do:
n = current n
Then:
n += 1
So n=n++ is the same as n++ -
madmattss757y@Root he asked if class knew exactly how the increment operator worked. Everyone was like "yeah ofc, how hard is it?" then he showed us this and there was like 1/100 who knew.
-
Oh FOR FUCKS SAKE GET RID OF THE GODDAMN LOOP IF ITS NOT BEING FUCKING USED.
Wow, Java devs really are efficient. I think I'll forward this to my prof... -
The postfix operator ++ has the higher precedence so it is evaluated first. It increments n but returns the old value, then the = operator assigns the result of the ++ operator (the old value of n) to n. So n is not incremented!
-
Break it up into two parts. First the assignment operator resolves setting n to itself. Then n increments itself.
-
Forside14527y@mgagemorgan This has nothing to do with Java or efficiency.
1) It's the same in every language.
2) It's to make people think about how code works. This does not have to be efficient or logical in any way. -
@Forside Meh. I dont do java because it uses a vm - also dont make me think if its not logical. I'm not arguing any further.
-
n++ means afaik n = n + 1
Therefore, I would assume that n = n++ would mean n = n = + 1
But having two equation symbols might break it. Idk. Barely have to do with Java lmao
Besides of that thing. If it would be a regular n=n+1 the result would be:
1
2
3
4
5
6
7
8
9 -
Something about the way int n is declared outside the loop looks wrong to me. I dont know what, it just looks wrong. Maybe it's because for anything more than i I just use a while() loop for whatever it is I'm doing. Been awhile since I've had the time to fuck around with constructs...actually ~6 months thanks to coursework.
-
Root796267y@gitpull `n++` returns the value of `n` and then increments it; two very separate operations. However, the `n=` portion adds a third.
The question thats actually being asked here is which one happens first; specifically, if the assignment portion of `++` has greater or less precedence than `=`. -
@Root I get your point, but would not 'n=' just assign the value of 'n++' to itself again. Like... you have the following.:
int n = 0;
n = n + 1; //n = 1
total = n; //still 1 until the next iteration -
Root796267y@gitpull
n=0 // initial value
// n = n++ could mean...
/* possibility 0 */
temp = n // n++ return portion
n += 1 // n++ assignment portion
n = temp // n=
// result: n=0
/* possibility 1 */
temp = n // n++ return portion
n = temp // n=
n += 1 // n++ assignment portion
// result: n=1
(Because I'm unfamiliar with Java and lack access to it presently, I don't know which one actually happens.) -
@gitpull Same. It's one of those example problems thats equivalent to the combination of "Ha! Made you look!" shit siblings do to each other and those workshits teachers give to see if you can follow directions. RIP critical thinking!
I dislike this because of the use of a separate variable used with a for() loop as an incrementor. I don't recall ever being in a situation where I needed a second variable, which may be why I hate this so much.
I have a headache and am probably overthinking this... -
@Root I've never used it, and don't plan to outside of required courses. I know the Oracle fuckboys over in IT are gonna wanna rip my guts out because I just talked shit about the only language they seem to know, buuuut..
-
@Root - I'm gonna laugh if the results are different. Then again, something as simple as structs seem to change constantly between languages. What's in C is NOT in C#.
-
Root796267y@mgagemorgan Absolutely. And after seeing so many things like this, I'm rarely surprised anymore ☹
-
@loin I dont know why I said for loop. I meant "n". Today has been a mess.
The real question is why the actual fuck I would want to print zero nine times in the first place... -
Wack61777y@gitpull we need answers. Does C and Java behave the same and what is the output of the C program? (Laying in bed and am to lazy to get back up :P)
-
Seems like it :)
Btw. the Running and Done thing in the terminal... it looks cool -
-
Wack61777yThinking about it, I actually "lerned" that in my first semester too.
If it would be `n = ++n` it should be as "expected" though. -
Root796267y@gitpull What I expected 😊
@Forside totally glad it's the same in Java haha
@Wack yep yep.
@mgagemorgan Seriously. This crap is only ever seen on college exams and within interns' code. And there's probably a strong correlation between the two... 😞😡 -
Forside14527y@gitpull It's the ouput of the VS Code Code Runner plugin ^^
@mgagemorgan I personally like experiments like these. Scrolling through the thread I just recognized the post by @electronix. Knowing that, it makes sense, but I also thought ++ would be executed after =. -
@Root Yeah, that's where my bias is. I cannot defend the stupidity of the code nor the predictability of a college prof for this.
"It makes you think" is a bullshit excuse - yeah, but THIS fails to prove anything. Other than the fact that it's horseshit... -
Forside14527yJust realizing how stupid I am.
If you ever learned how operators are defined in C, you know that it behaves like this:
n = n++
n = {
int tmp = n;
n++;
return tmp;
}
So yeah, in the end it's n=n. -
eeee31177y@calmyourtities it's staggering that you get so many upvotes for your incorrect answer. Maybe it's because you answered first? Maybe it's just me not getting the joke of answering incorrectly, but probably the worst case scenario is true: most people just don't understand the code.
Everybody should know about the ++n and n++ expressions and what their difference is. If you'd have known that, then the only logical explanation is that if n = ++n is something different than n = n++, then the result of n = n++ would not be that n is changed.
The most important thing to remember is that assignment (n = ...) happens last: after the right part of the equation has been evaluated. The right part returns 'n before incrementing' by definition of the n++ operation. I don't care if n is set a million times on the right hand side, just that the right side returns the original n's value. -
@eeee It's the latter. And it's also not something I'd ever write personally in any of my projects.
-
@eeee lol man i know i saw that after the first comment that said that but it's free ++
Related Rants
-
Cyborg15A guy and a girl are in a Java seminar. Afterward, the guy approaches the girl and asks, "Hey gurrl, can I ge...
-
linuxxx32*client calls in* Me: good morning, how can I help you? Client: my ip is blocked, could you unblock it for m...
-
DRSDavidSoft29
Found this in our codebase, apparently one of my co-workers had written this
*** don't use compiler ***
Question in class today:
int n = 0;
for (int i = 1; i < 10; i++) {
n = n++;
System.out.println(n);
}
what will be printed?
undefined
java
wtf
i really don't know code
mindfuck