Ranter
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
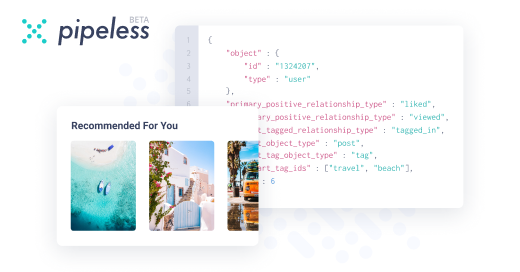
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Comments
-
It's definitely not how you want to use php, but if you need some temp code to be hacked together winthin seconds, php allows these weird things that allow utterly rapid coding..
But if you arent going to delete this within minutes (come on, everyone of us wrote some code to be one-time-use to replace us doing manual task, no?) it screams for refactor.
But yes, you can do that. There is also runkit that allows you to unset function among other things. Even unset built in function to replace it with own implementation for even more "O.O"-ness
https://php.net/manual/en/...
(Requires extension)
Php is weird language that gives you all the tools, to write properly and improperly and basically leaves you with 'you do you' -
C0D4652034yYes. And you as the dev will be slapped with my keyboard so hard you'll be eating alphabet soup for a month.
Just because you can, doesn't mean you should 😏 -
You can do this with almost any scripting language. I don't see how this is strange.
I also don't see how you would do this with C without using function pointers, which are a bit more explicit.
Lua:
if _G.some_function == nil then
function some_function() end
end
Javascript:
if (typeof some_function === 'undefined')
function some_function() {}
Python (pseudo code, can't remember off the top of my head)
if locals().get('some_function', None) is None:
def some_function():
pass
ad nauseum... -
@junon well... yes, that's what I mentioned on the end there, except i said JavaScript instead of "almost any scripting language".
the thing is, lately, I'm kind of... more and more wishing for a JS-like language, in syntax (partially), and in how the dev console in the browser is basically a living environment, except with a sane logic to it...
while at the same time the only languages I know that are kind of close to it are precisely the moronic ones like JS and Python, which is probably what causes the confusion of "this is cool, but weird, but cool, but stupid, but cool..." -
Root777904yIn Ruby, you can open an instance of a class and redefine methods on only that instance.
Sound crazy?
It’s incredibly useful for specs since you can open an instance and curry or replace a method to count its runtime invocations, check call arguments, override its return value, etc. -
@Root Javascript too, it's just becoming a lost art with all of the new Class syntax.
I really should learn Ruby, though. It's the only mainstream language I still haven't really looked at at all... -
Root777904y@junon I will always agree with that.
It’s always good to pick up another language. Especially when it’s Ruby or Gaelic. 😉 -
@Root oh shit, uh... gaelic doesn't have no, so... "Cha agat"? Is that close?
It was cursory xD I have no command of any of it whatsoever. Do you speak it? -
If you find this fascinating, dig deep into reflection.
And FFI.
Shit you can do will make you regret the choice too look at it.
Forbidden fruit ;) -
Hazarth93944ywhen you think about it, this isn't all that far off from doing a command pattern... just instead of creating a new object implementing a common interface you just directly return the function so...
interface SomeAction
class Action1: SomeAction{
void run(){...code1...}
}
class Action2:SomeAction{
void run(){...code2...}
}
SomeAction action = null;
if (var == something) action = new Action1();
else action = new Action2();
this is roughly what it's doing and this is.. while rare, still a valid design pattern (though I doubt that php used it correctly, I'd need to see more of the code? and if it is then it's stateful and ...ew..)
so it's arguably shorter to write it by passing functions around (you can do it in C too)
but yeah, I always found objects to be more... readable? ... really depends on the code though!
so to me this isn't a huge mindfuck I think, it's just mildly annoying that someone would do it like that :D -
@Hazarth yeah, that's probably my only real problem with it: it's implemented in such a way that it's absolutely not visible it's happening.
at least with objects i am aware there might be some overloading going on and where to look for it.
with functions explicitly assigned to a variable it's obvious that this can happen, possibly even that it does happen which is why the function was assigned into a variable.
with this conditional definition of standalone function nothing hints that this could be the case and the only way to find it is to do a project-wide search for "function functionName".
which you wouldn't know to do unless you already know it's been done to the function, which you wouldn't know unless you do the search (or start seeing aggravating UFO bugs) -
on an unrelated note, can anyone explain what the difference between UFO bug and a heisenbug is?
-
@Midnight-shcode Keeping in mind these are not terms people really use often (more for fun, less for actual work):
- Heisenbug means the bug changes its behavior when you try to debug it.
- UFO bug is a bug that customers/users insist exist, even though they can't reproduce it on command, and might still insist it exists even after you've proven it does not/cannot exist.
Related Rants
did you know, that in PHP, you can do:
if ( ! function_exists('function_name'))
{
function function_name()
{
//code of the function
}
}
which apparently means you can do
if($var == 'something'){
function functionName(){
//some code
}
} else if($var == 'something else'){
function functionName(){
//some completely different code
}
}
so now, apparently:
1. before this code executes, the function doesn't exist at all (okay, i can live with that)
2. after this code executes, any call to that function can result in any of those two completely different bodies of the same-name function executing, depending on what the $var was set at that time?
...so... now not only the same call to the same(name) function can do two completely different things, *but if you change the value of $var afterwards, you can't even properly find out which version of that function is in effect for the remainder of the run of the script*...?????
WHAT.
THE.
...i mean... I can't help but think that the idea of conditional function declaration like this is... kind of cool (have I been warped by JavaScript too much?), but at the same time... WHAT THE FUCK.
rant
php