Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
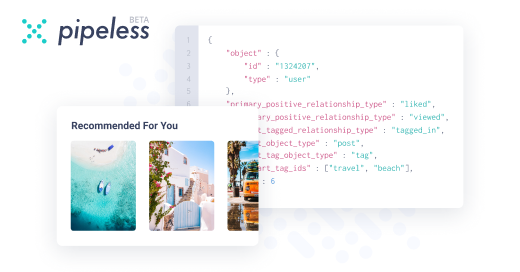
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "typedef"
-
Today I experienced cruelty of C and mercy of Sublime and SublimeLinter.
So yesterday I was programming late at night for my uni homework in C. So I had this struct:
typedef struct {
int borrowed;
int user_id;
int book_id;
unsigned long long date;
} entry;
and I created an array of this entry like this:
entry *arr = (entry*) malloc (sizeof(arr) * n);
and my program compiled. But at the output, there was something strange...
There were some weird hexadecimal characters at the beginning but then there was normal output. So late at night, I thought that something is wrong with printf statement and I went googling... and after 2 hours I didn't found anything. In this 2 hours, I also tried to change scanf statement if maybe I was reading the wrong way. But nothing worked. But then I tried to type input in the console (before I was reading from a file and saving output in a file). And it outputted right answer!!! AT THAT POINT I WAS DONE!!! I SAID FUCK THIS SHIT I AM GOING TO SLEEP.
So this morning I continued to work on homework and tried on my other computer with other distro to see if there is the same problem. And it was..
So then I noticed that my sublime lint has some interesting warning in this line
entry *arr = (entry*) malloc (sizeof(arr) * n);
Before I thought that is just some random indentation or something but then I saw a message: Size of pointer 'arr' is used instead of its data.
AND IT STRUCT ME LIKE LIGHTNING.
I just changed this line to this:
entry *arr = (entry*) malloc (sizeof(entry) * n);
And It all worked fine. At that moment I was so happy and so angry at myself.
Lesson learned for next time: Don't program late at night especially in C and check SublimeLInter messages.7 -
PM is such a fucking cunt
telling me that my data structures describing the layout of binary data would be confusing for devs, and that we shall introduce
typedef fuckingRetardedObfuscatingName uint8_t;
in our code. everyone is fine with the concepts i provide to describe this binary data, not only at our company but also in other software i've worked on and common standards i've worked with, we work like that and every fucking idiot knows what a uin8_t is.
you fucking braindead imbecile have no fucking idea how we work and you don't care, you don't even try to understand what we are doing.
god i hope you die being hit by a fucking bus or something8 -
Today, I found this gem here in the codebase I've taken over:
#define BYTE unsigned char
FFS, use typedef, it's there for a reason. Solving the puzzle in the first comment.6 -
As an exercise lets see how many different ways we can wish devRant Happy Birthday in code. Try not to copy peoples examples, use a different language or different method.
A couple of examples to start the process:
* LOLCODE *
HAI 1.3
LOL VAR R 3
IM IN YR LOOP
VISIBLE "Happy Birthday"!
IZ VAR LIEK 1?
YARLY
VISIBLE "Dear devRant"!
NOWAI
VISIBLE "to you"!
KTHX
NERFZ VAR!!
IZ VAR LIEK 0?
GTFO
KTHX
KTHX
KTHXBYE
* C *
#include <stdio.h>
#define HP "Happy birthday"
#define TY "to you"
#define DD "Dear devRant"
typedef struct HB_t { const char *s; const char *e;} HB;
static const HB hb[] = {{HP,TY}, {{HP,TY}, {{HP,DD}, {{HP,TY}, { NULL, NULL }};
int main(void)
{
const HB *s = hb;
while(s->start) { printf("%s %s", s->s, s->e); }
return 1;
}12 -
Whyyyyyyyyyy are you making typedefs for a type that you only need once
Just leave the array<uint32, 20> there
It's actually less readable to typedef it6 -
Making a function pointer in C and typedef-ing it.
Done wrong. Every single time.
Look up in the internet.
Copy a typdef-d function pointer.
It works.
Check it's syntax.
THE SAME FCKIN SYNTAX AS DONE FIRST!
And that's why I hate function pointers.2 -
Currently working on a GUI config generator using MFC in VS.
Firstly, fuck sake Microsoft. Why can't I just use a normal string? The amount of times I've had to do god awful conversions to/from CString using their numerous typedefs L, _T and don't even get me started on LPCTSTR, LPCWSTR... It's just ugly and tedious. I've gotten used to it and all but still, ugh.
Secondly, some of the functions are just stupid. Want to disable a control? Hmm, we'll there's a function called EnableWindow, but no DisableWindow. How did I do it before? Oh, so to disable the control it's EnableWindow(FALSE). Of course it is, duh. Why am I so stupid?
Let's use the GetWindowText function. Simples. CString something_txt = GetWindowText().
Nope, it takes the CString as a parameter and copies it into that rather than just returning the text. Now one line becomes two. I get that this is a really small semantic thing but it irks me.
I just want to go back to my fedora partition. Wah.
PS: I'm sure there's good reasons for what I'm ranting about, but I really don't care. I just need to rant about my frustrations. 😂1 -
Working with the codebase from hell here:
struct UnitNode: Node {
typedef Node super;
Stop making C++ look like Java! -
One of those "you have got to be kidding me moments":
struct Speaker: UnitNode, MemBufferBase {
typedef UnitNode super;
…
}
And then elsewhere:
#define Node UnitNode
#define Speaker AudioSpeaker
Never seen anyone typedef base class as super in C++ nor use a #defined variable as a class name. And of course elsewhere in the code class names are normal literal a but are referenced via a #define (and sometimes not via the #define)... The same obfustication done two different ways! -
typedef bool Bool;
Class Description
{
public:
inline Bool IsTypeA() const { return IsType(TYPE_A); }
inline Bool IsTypeB() const { return IsType(TYPE_B); }
inline Bool IsTypeC() const { return IsType(TYPE_C); }
Bool IsType(DescriptionType T) const { return (T == Type()); }
DescriptionType Type() const { return m_Type; }
private:
DescriptionType m_Type;
}
I can't make this shit up3 -
I FUCKING HATE TYPEDEF.
I have NEVER seen a use of typedef that wasn't UTTERLY pointless AND did absolutely nothing except add obfuscation to code.
What the fuck is the point of typedeffing a size_t to "MapIndex".. And THEN typedeffing a vector... Of vectors... Of size_t (not MapIndex!) AND THEN TYPEDEFFING A MAP OF "MapIndex" to that previously typedeffed vector of vectors....
ITS FUCKING BEYOND STUPID. I absolutely hate typedef. I have never seen a single NOT RETARDED usage of it.
Have you?7