Details
-
Skillsjs
-
LocationLondon
-
Website
-
Github
Joined devRant on 4/7/2018
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
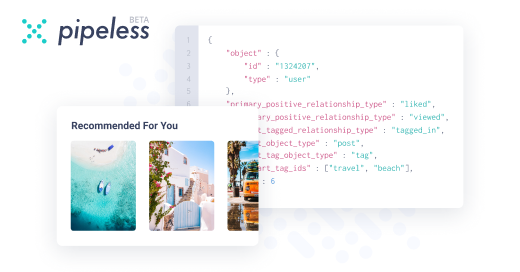
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
-
When I started with javascript long time ago I thought JS is weird enough, but Swift is even more.
Why does it allow me to compile the code below? In the last line `taker.take(view)`, the `view` is an optional passed into a function that expects non-optional `some View`. How is this even possible!? I tried to change the view with some the other protocols, then it complaints, why the `View` protocol is different from the others?
```
import SwiftUI
struct ViewStruct: View {
var body: some View {
Text("")
}
}
class Taker {
func take (_ view: some View) {
print(view)
}
}
class Container {
var view:ViewStruct?
func createView() {
view = ViewStruct()
}
func test() throws {
let taker = Taker()
guard let viewIsView = view else {
throw fatalError()
}
taker.take(view)
}
}
```7 -
when the swift language cannot infer the generic type from the invocation, it also doesn't want you to explicily specify generic type in the bracket...
says i have a method:
```
func createDeferred<T>() -> ABC.XYZ.Deferred<T>
```
then if you call it like this:
```
let dfd = createDeferred()
```
It complaints that it cannot infer generic type T, which make sense.
But it also doesn't want you to code it this way:
```
let dfd = createDeferred<Int>("countProperty")
```
if you do so, it mumbles gibberish: "Cannot explicitly specialize a generic function".
What it actually trying to say is, you should put the type somewhere else so that it can show off its smartness to "infer" it from there:
```
let dfd: ABC.XYZ.Deferred<Int> = createDeferred()
```
with a few more typing and findout what exact type it is, it finally works.
the moral of the story is, in order to communicate with the wonderful work apple genius made, you don't tell it what is the answer straight away, that's defiance, you must hide the answer somewhere intricately and let the smarty swifty swift to find it out for you.6 -
in 2023, swift still doesn't have a tracing GC, and they are still using reference counting to decide when to deallocate an instance, surprise! what's even worse is closures are everywhere and the default way to define a closure make it possible to keep a reference to variables in the parent scope.8
-
in swift, the default modifier for `init()` of a struct is `internal`, regardless what the struct's modifier is. why can't they just change it to inherit the modifier from the struct? why would anybody want to define a public struct and yet keep the `init()` internal????3
-
in apple's blog they explained why they don't want a `protected` in swift:
https://developer.apple.com/swift/...
> It doesn’t actually offer any real protection, since a subclass can always expose “protected” API through a new public method or property."
Isn't the same thinking applies to `internal` keyword as well? Yet they allow `internal` to be there as default modifier for `class` in a package. Also I don't think `protected` is for the sole purpose of "protection", but for the cleanliness of externally visible interface, some methods are just useless to be exposed and will confuse the consumer if they don't understand internally how the class works. So it doesn't have to be 100% securely `protected` (arguably the term `protected` is a poor choice tho).
but hey, it is apple, being opinionated doesn't surprise me.6 -
to slice a string in swift in 2022:
```
let anotherIndex = str.index(str.endIndex, offsetBy: -1)
let newStr = str[...anotherIndex]
```
Wow, thanks apple5 -
In google play store we enabled internal testing track of my app for my testing account. when I visit the page with testing account it says "Internal beta", and few lines below it says I am a "beta tester". Is beta tester the same as internal tester? can't google even consolidate the terms?2
-
Added vapor core swift package to my project so that I can use some of the extensions, had to `rm -rf ~/Library/Developer/Xcode/DerivedData/` to make xcode realise the repo is actually exist.
Then I set the Core package as dependency of one of the targets, XCode complaints "unknown package Core", ok fine then I remove Core dependency, Core complaints "dependency Core is not used by any target".
How can xcode comes to this contradicting conclusion is out of my imagination, it just never gonna be happy about however i write.1 -
why can't apple even provide a consistent interface to load swift package? "add package dependency" interface allows you to add remote package, but doesn't allow you add local in development swift package. to do that, you will have to "drag and drop"... why can't apple just add a button on the same interface for navigate local package? is this suppose to be some hidden up up down down left right left right konami secret thing?
-
when xcode fails to load swift package dependency, it just fail silently, it removes targets from my build menu quietly and say nothing about why that fail, leaving me stranded and confused and spent hours to figure out why the target defined in the package.swift file just doesn't show up. I remember "UX" used to be the buzz word Steve Jobs bragging about?1
-
React's `useEffect()` won't fire if you have someone in your team wrote a hook that maintain a state of an array, mutates the array, empties it, and then set it back to the state.
https://codesandbox.io/s/...
Reported it, ticket closed without asking, told should avoid mutating the object stored in useState.
Isn't it bluntly obvious that if someone spent hours to spot the line in hundreds of lines of code, which actually caused the problem and reduce the whole piece of turds into some understandable minimal reproducible example means they must of course for sure know that by avoiding mutating the array it will fix the bloody issue?
Isn't that bluntly obvious they are trying to say that there is a bigger issue behind those twisted wires?9 -
why did kotlin documents use these fucking idiotic font that combines 2 characters into one stupid looking icon? how is it friendly to a noob? how am i suppose to know how exactly am I suppose to input these piece of shit?21