Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
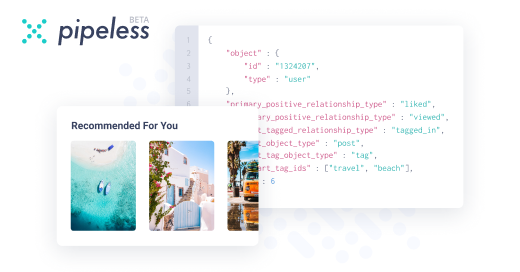
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "why do i need all that overhead...?"
-
Okay, story time.
Back during 2016, I decided to do a little experiment to test the viability of multithreading in a JavaScript server stack, and I'm not talking about the Node.js way of queuing I/O on background threads, or about WebWorkers that box and convert your arguments to JSON and back during a simple call across two JS contexts.
I'm talking about JavaScript code running concurrently on all cores. I'm talking about replacing the god-awful single-threaded event loop of ECMAScript – the biggest bottleneck in software history – with an honest-to-god, lock-free thread-pool scheduler that executes JS code in parallel, on all cores.
I'm talking about concurrent access to shared mutable state – a big, rightfully-hated mess when done badly – in JavaScript.
This rant is about the many mistakes I made at the time, specifically the biggest – but not the first – of which: publishing some preliminary results very early on.
Every time I showed my work to a JavaScript developer, I'd get negative feedback. Like, unjustified hatred and immediate denial, or outright rejection of the entire concept. Some were even adamantly trying to discourage me from this project.
So I posted a sarcastic question to the Software Engineering Stack Exchange, which was originally worded differently to reflect my frustration, but was later edited by mods to be more serious.
You can see the responses for yourself here: https://goo.gl/poHKpK
Most of the serious answers were along the lines of "multithreading is hard". The top voted response started with this statement: "1) Multithreading is extremely hard, and unfortunately the way you've presented this idea so far implies you're severely underestimating how hard it is."
While I'll admit that my presentation was initially lacking, I later made an entire page to explain the synchronisation mechanism in place, and you can read more about it here, if you're interested:
http://nexusjs.com/architecture/
But what really shocked me was that I had never understood the mindset that all the naysayers adopted until I read that response.
Because the bottom-line of that entire response is an argument: an argument against change.
The average JavaScript developer doesn't want a multithreaded server platform for JavaScript because it means a change of the status quo.
And this is exactly why I started this project. I wanted a highly performant JavaScript platform for servers that's more suitable for real-time applications like transcoding, video streaming, and machine learning.
Nexus does not and will not hold your hand. It will not repeat Node's mistakes and give you nice ways to shoot yourself in the foot later, like `process.on('uncaughtException', ...)` for a catch-all global error handling solution.
No, an uncaught exception will be dealt with like any other self-respecting language: by not ignoring the problem and pretending it doesn't exist. If you write bad code, your program will crash, and you can't rectify a bug in your code by ignoring its presence entirely and using duct tape to scrape something together.
Back on the topic of multithreading, though. Multithreading is known to be hard, that's true. But how do you deal with a difficult solution? You simplify it and break it down, not just disregard it completely; because multithreading has its great advantages, too.
Like, how about we talk performance?
How about distributed algorithms that don't waste 40% of their computing power on agent communication and pointless overhead (like the serialisation/deserialisation of messages across the execution boundary for every single call)?
How about vertical scaling without forking the entire address space (and thus multiplying your application's memory consumption by the number of cores you wish to use)?
How about utilising logical CPUs to the fullest extent, and allowing them to execute JavaScript? Something that isn't even possible with the current model implemented by Node?
Some will say that the performance gains aren't worth the risk. That the possibility of race conditions and deadlocks aren't worth it.
That's the point of cooperative multithreading. It is a way to smartly work around these issues.
If you use promises, they will execute in parallel, to the best of the scheduler's abilities, and if you chain them then they will run consecutively as planned according to their dependency graph.
If your code doesn't access global variables or shared closure variables, or your promises only deal with their provided inputs without side-effects, then no contention will *ever* occur.
If you only read and never modify globals, no contention will ever occur.
Are you seeing the same trend I'm seeing?
Good JavaScript programming practices miraculously coincide with the best practices of thread-safety.
When someone says we shouldn't use multithreading because it's hard, do you know what I like to say to that?
"To multithread, you need a pair."18 -
Don't waste your time - they said.
Use Spring - the good ol' framework - they said
It's not slow - they said
me: ignores them, builds a custom jetty-based webserver with the same functionality Spring+tomcat can offer (mappings, routings, etc).
My app: boots up completely in <300ms, while Spring tutorials say a hello-world app takes 3+ seconds to spin-up http://websystique.com/spring-boot/...
me: already set for deployment in lambda. I bet I can tune it up even further with lazy-loading if I really have to...
Moral of the story: sometimes bare-bones solution is a better choice: more performant, more extendable, more testable, more lightweight.
That, dear folks, is the classic LESS IS MORE :)12 -
In a meeting yesterday working through our WebAPI coding standards, starting from File -> New project..etc..etc.. and ironing out some of the left-or-right decisions so we can have a consistent coding style, working in a meeting room with an overhead projector and sharing keyboard around with one another.
Then we hit the routing 'rules' in the WebApiConfig, "api/{controller}/{id}"…
DevMgr: "Do we need the 'api' prefix? It seems redundant."
Ralph: "Yes it's needed. Prefixing the controllers with 'api' is industry best practice. Otherwise, how is anyone to know it's a web api"
Prancer: "Yea, it's part of the REST standard."
Me: "I don't think so. That is only part of the Asp.Net routing rule. We can put anything we want or take anything out."
DevMgr: "Yea, it looks silly. All the new services are going to be business process specific."
Ralph: "That's how everyone does it. It's kind of the point of why REST services are called WebApi"
Prancer: "What's the point of doing any of this work if we're not going to follow industry standards."
Me: "I understand if the service is part of larger web site, but we're developing standalone services. Prefixing routes with 'api' is redundant. I mean who are these 'everyone' you're talking about?"
<ralph rolls his eyes>
Ralph: "Lets see …uhhh… Netflix?. They're kinda a big deal."
Me: "Like I said, it's an integral part of their site and the services they provide. That's fine. I'm talking about the 12 other 3rd party services we integrate with. None of them have 'api' on any of their routes."
Prancer: "We're talking about serious web services."
Me: "Last time I checked, UPS is a big and serious service."
Ralph: "Their services are a fracking joke" – he didn't say fracking.
Me: "Our payroll system, our billing system, billion dollar companies, didn't have '/api' prefix anywhere. Heck, even that free faxing service we used for a while was a dead-simple routing path."
<I take the keyboard away from Ralph, remove the 'api' from the route.>
Me: "There. Done. Now, lets talk about error handling.."
Rest of the meeting Ralph and Prancer don't say much of anything, arms crossed…I swear Ralph looked like he was going to cry.
This morning I catch my boss…
Me: "What did you think of the meeting? I thought Ralph was going to take a swing at me when I took the keyboard away from him."
DevMgr: "Oh yes…I almost laughed out loud….blows my freaking mind how worked up people get about crap that doesn't matter. Api..or not…who the frack cares. Just make it consistent"
Me: "Exactly…I didn't care either way, but I enjoyed calling out that nonsense."
DevMgr: "Yes..waaay too much."
If I didn't call them on their BS and the 'standard' allowed to continue, I can bet my paycheck when the subject comes up in a few months (another mgr asks 'isn't this api prefix redundant?') Ralph and Prancer will be the first to say "Yea, its stupid. We fought really hard to remove it from the standard...its not our fault...its <insert scapegoat> fault." -
WFH is nice, esp when you can spare a separate room for work at your house/apartment. VoIP is also not a problem, it's even better is several ways than the real thing, as you can chat with multiple people at the same time without dragging them off their desks. Even better - screen sharing sessions.
However, there's one thing I don't understand.Why would anyone do video calls? I mean.. why...? What's the point? If I'm on metered network I'll be charged extra only because I'll have to stream out my ugly face to people who don't want to see it and stream in all the other ugly faces I don't want to see. My voice will become laggy, I'll miss out on some details, and all that because of some completely unnecessary overhead.
So why would you want me to enable my webcam? And why do you feel the need to show your face to everyone else? Why is this necessary...?24 -
Just discovered https://twitter.com/ExpertBeginner1. It's the story of my life. Giant classes, copying and pasting, and architects who create frameworks. It's great when we combine all three: A "framework" created by an architect which is made of giant classes that you copy and paste. Imagine a giant generic class where the generic argument is only used by dead code. Pause for a moment and try to visualize that.
It inherits from a base class with lots of virtual methods called by base methods that throw NotImplementedException, so if you don't need them you have to override them to return empty collections. If you're going to do something so messed up you could just put those default implementations in the base. But no, you can inherit, it compiles, and then it throws a runtime error unless you override methods the compiler doesn't require you to override.
The one method you're required to override has a TODO comment telling you what to put there. Except don't ever do what the comment says because that's the old standard. The new standard says never, ever do that.
Most of the time when I read about copy-and-paste coding it's about devs who copy and paste because they don't know how to write or reuse code. They don't mention the environments where copying and pasting the same classes over and over again is the requirement and you're not allowed to write your own code.
Creating base classes where you just override a method or two can potentially work, but only in the right scenarios and only if you do it right. If you're copying and pasting a class that inherits from the base class and consists entirely of repeated code, why the heck isn't that the base class? It could be a total mess, but at least it would be out of sight and each successive developer wouldn't become responsible for it by including it in their own code.
It's a temporary engagement, but I feel almost violated. I know it's a first-world problem, and I get to work indoors and take vacations. I'm grateful for those things.
Before leaving I had to document the entire process of copying and pasting an entire repo, making a ton of baseline edits that should just be in the template but aren't, and then copying and pasting from other places into the copied and pasted code. That makes me a collaborator. I apologize more than once in the documentation, all 20 pages of it that you have to read and follow before you even get to the part where you write the code for what you actually need it to do.
This architect has succeeded in making every single thing anyone does more about servicing the needs of his "framework" than about writing actual code to do what needs doing. Now that the framework is in and around everything it creates the illusion that it's a critical part of our operations. It's not. It's useless overhead.
Because management is deceived into thinking they need it they overlook the fact that it blows up, big and small, every single day. The log is full of failures that I know no one ever sees. A big chunk of what they think it does fails silently, and they don't even notice until months later when they realize how much data they're missing. But if they lose, say, 25% they'll never notice.
When they do notice they just act like it's normal, go into fire drill mode, and fix it. Doom. You're all doomed. I'm standing on the deck of the Titanic next to my jet ski.1