Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
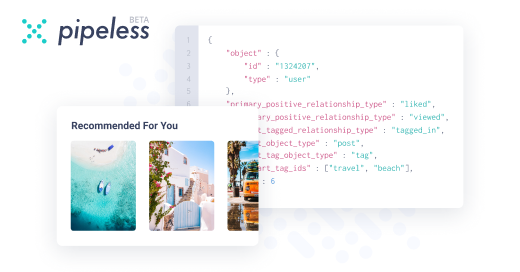
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "halting problem"
-
Today, for fun, I wrote prime number generation upto 1000 using pure single MySQL query.
No already created tables, no procedures, no variables. Just pure SQL using derived tables.
So does this mean that pure SQL statements do not have the halting problem?
Putting an EXPLAIN over the query I could see how MySQL guessed that the total number of calculations would be 1000*1000 even before executing the query in itself and this is amazing ♥️
I have attached a screenshot of the query and if you are curious, I have also left below the plain text.
PS this was a SQL problem in Hackerrank.
MySQL query:
select group_concat(primeNumber SEPARATOR '&') from
(select numberTable.number as primeNumber from
(select cast((concat(tens, units, hundreds)+1) as UNSIGNED) as number from
(select 0 as units union select 1 union select 2 union select 3 union select 4 union select 5 union select 6 union select 7 union select 8 union select 9) unitsTable,
(select 0 as tens union select 1 union select 2 union select 3 union select 4 union select 5 union select 6 union select 7 union select 8 union select 9) tensTable,
(select 0 as hundreds union select 1 union select 2 union select 3 union select 4 union select 5 union select 6 union select 7 union select 8 union select 9) hundredsTable order by number) numberTable
inner join
(select cast((concat(tens, units, hundreds)+1) as UNSIGNED) as divisor from
(select 0 as units union select 1 union select 2 union select 3 union select 4 union select 5 union select 6 union select 7 union select 8 union select 9) unitsTable,
(select 0 as tens union select 1 union select 2 union select 3 union select 4 union select 5 union select 6 union select 7 union select 8 union select 9) tensTable,
(select 0 as hundreds union select 1 union select 2 union select 3 union select 4 union select 5 union select 6 union select 7 union select 8 union select 9) hundredsTable order by divisor) divisorTable
on (divisorTable.divisor<=numberTable.number and divisorTable.divisor!=1)
where numberTable.number%divisorTable.divisor=0
group by numberTable.number having count(*)<=1 order by numberTable.number) resultTable;9 -
Buckle up, it's a long one.
Let me tell you why "Tree Shaking" is stupidity incarnate and why Rich Harris needs to stop talking about things he doesn't understand.
For reference, this is a direct response to the 2015 article here: https://medium.com/@Rich_Harris/...
"Tree shaking", as Rich puts it, is NOT dead code removal apparently, but instead only picking the parts that are actually used.
However, Rich has never heard of a C compiler, apparently. In C (or any systems language with basic optimizations), public (visible) members exposed to library consumers must have that code available to them, obviously. However, all of the other cruft that you don't actually use is removed - hence, dead code removal.
How does the compiler do that? Well, it does what Rich calls "tree shaking" by evaluating all of the pieces of code that are used by any codepaths used by any of the exported symbols, not just the "main module" (which doesn't exist in systems libraries).
It's the SAME FUCKING THING, he's just not researched enough to fully fucking understand that. But sure, tell me how the javascript community apparently invented something ELSE that you REALLY just repackaged and made more bloated/downright wrong (React Hooks, webpack, WebAssembly, etc.)
Speaking of Javascript, "tree shaking" is impossible to do with any degree of confidence, unlike statically typed/well defined languages. This is because you can create artificial references to values at runtime using string functions - which means, with the right input, almost anything can be run depending on the input.
How do you figure out what can and can't be? You can't! Since there is a runtime-based codepath and decision tree, you run into properties of Turing's halting problem, which cannot be solved completely.
With stricter languages such as C (which is where "dead code removal" is used quite aggressively), you can make very strong assertions at compile time about the usage of code. This is simply how C is still thousands of times faster than Javascript.
So no, Rich Harris, dead code removal is not "silly". Your entire premise about "live code inclusion" is technical jargon and buzzwordy drivel. Empty words at best.
This sort of shit is annoying and only feeds into this cycle of the web community not being Special enough and having to reinvent every single fucking facet of operating systems in your shitty bloated spyware-like browser and brand it with flashy Matrix-esque imagery and prose.
Fuck all of it.20 -
Today’s topic in my Computability & Complexity Class:
The Halting Problem and The Undecidability Results
...sounds like my life2 -
I turned my computer off. When I came back it said "Reboot: System Halted".
I think my computer has a halting problem. -
So I've spent some time learning a little about the halting problem, and it's quite fascinating. I tried to simplify it down to these few functions. What do you guys think? Obviously, psuedo-code, so don't get too caught up on the syntax 😆
The Halting Problem:
public String doesItHalt(Callable function){
...
if (...){
return "Yes"
} else {
return "No"
}
}
public int someFunctionFooThatHalts(){
...
}
public int someFunctionFooThatDoesNotHalt(){
...
}
public String inverseAnswer(value){
if (value == "Yes"){
return "No"
}
if (value == "No"){
return "Yes"
}
}
public String inverseHalts(Callable function){
return inverseAnswer(doesItHalt(function))
}
————————————————————————————
$ doesItHalt(someFunctionFooThatHalts)
Yes
$ doesItHalt(someFunctionFooThatDoesNotHalt)
No
$ inverseHalts(someFunctionFooThatHalts)
No
$ inverseHalts(someFunctionFooThatDoesNotHalt)
Yes
$ doesItHalt(inverseHalts(doesItHalt))
???2